ā Input into an int array
The input would be something like "1 2 3 4 5", how could I convert it into an int array/several variables?
140 Replies
Think about it.
You have a single string that contains all your values.
There is a method that takes a string and parses it into a single int. We cant use that here, as it would be confused
if you were to do this in pseudocode, just describing the steps, what would you do?
hmm probably convert the string char by char until a space, then move to the next variable/array slot
hm this is probably doable with a cycle, but is there any better method?
Well, there is a built in function to split one string into many
by giving it a pattern to look for
what is it?
string.Split
you can use it on your string directly
yourInputVar.Split(...)
oh I think this is exactly what I was looking for, thanks!
almost š
it gives you a
string[]
back
so you still need to do something to that to turn it into an int[]
found
Array.ConvertAll
on google and it works perfectly, ty for helpthat works, I guess. Not what I'd have gone with personally
What would you do?
s.Split(' ').Select(x => int.Parse(x)).ToArray();
huh, intresting
tysm again
Or even simpler:
s.Split(' ').Select(int.Parse).ToArray();
It's not considered bad practice using those extension methods in non-LINQ code? I know they are part of
Enumerable
and not necessarily LINQ limited, didnt see them very often until LINQ though.
(sorry OP if im bloating your post)wdym?
why would it be bad to use LINQ?
I mean I dont know, I dont think its bad. Not sure if thats normal
you mean use linq on an array not a list ?
I wouldn't do it in my 144 fps games hot loop, but in 99% of normal code its not only normal, its encouraged
I mean an Array would be deriving from IEnumerable, so it being an array doesnt matter
it does, yes
I mean okay, were these extension methods initially meant for LINQ but OK to be utilized outside of LINQ queries?
LINQ leads to short, readable, understandable code, at the cost of a tiny tiny performance degradation (over manual loops)
uhm
what do you think LINQ is?
this IS a linq query
Yes, since you it a linq query by using the extension methods
you don't have to write the sql like things to use linq
I just dont understand your question, in either of your sentences
I know they are part of Enumerable and not necessarily LINQ limited.Select and .ToArray are both LINQ methods but what is "non-LINQ code" and "LINQ code"? thats... not a distinction LINQ is just a collection of extension methods that work on most if not all collections, thanks to
IEnumerable
If i am not wrong this doesnt utilize any of the LINQ extension methods
and achieves the same, this is what i'd refer to as "non-LINQ" code, simply not using LINQ extension methods and therefore not creating a query
hm, wasn't aware that
Converter<TIn, TOut>
had an implicit operator for Func<TIn,TOut>
but yeah, this would do exactly the same thingthe whole concept of just creating a "query" to put values into an array seems so forgein to me ig
seems wrong
.. a query? there is no query
not in this case
there is a lazy select iterator
I mean in the examples given above by you and the xtreit
there is no query in those either, by most definitions of query
so utitlizing LINQ extension methods != it being a query?
also, can we even use the term query without a context?
like, how do you define a query
like, a question or request i suppose
in english that is
when talking about SQL-context its more request
but there is no SQL involved here, no databases
its just an array.
yeah exactly
you said it was a query though
previously
this
well, I used that term because you did
because technically its true
if you refer to an IEnumerable iterator as a query
like, thats fine, its fairly common to do so
but there is no... request being made here
not to a data source like SQL, XML, etc no
so I wouldnt call it a query
then
personally
okay, then there are no queries š
its just a method being called once for each item in an array
either way, so no harm in using these LINQ extensions anywhere I wish
unless I want performance
linq queries are much lighter and simpler than they seem
for example, ToArray takes into account the size of the source array to allocate the space it needs right away
there are plenty of such optimizations there
I don't see any reason to use any more "specific" methods for working with collections
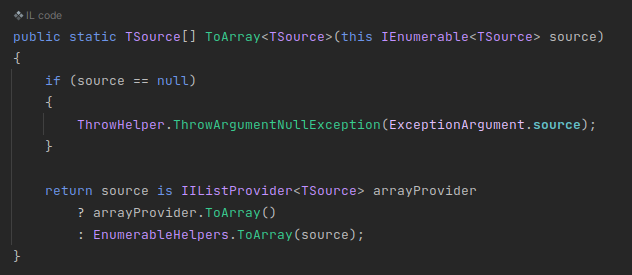
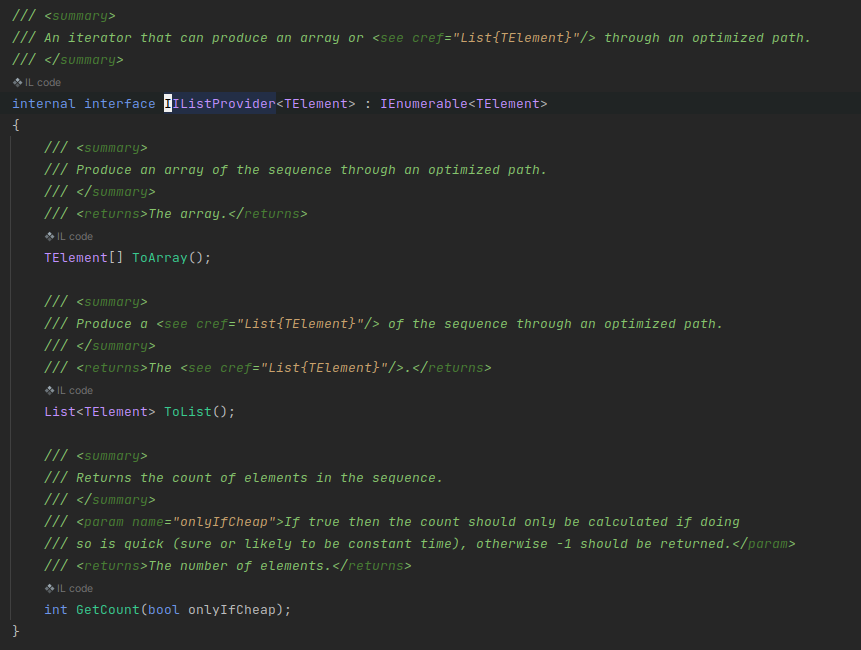
yeah, LINQ is incredibly well written and uses some freaky magic in the background to make it faster
it has a tiny overhead compared to self-made
for
loops
tiiinyOkay, seems like LINQ extension methods should be considered universal and utilized for both actual queries (from a DB for instance) and normal code.
mhm
yep!
I'll keep that in mind
and actually, when used against a DB via something like EFCore
its not actually LINQ š
though if I want performance I prob wouldn't opt for C# due to my lack of knowledge and inability to optimize as much as I could in C++
they just have the same methods that behave the same way, but its on
IQueryable
and uses expressions to compile into SQLmaybe that changes after I have a better grasp on C#
makes sense, thanks
yeah depends on the usecase tbh. C# can be very fast, if written well - but ultimately its a garbage collected language
if you want to reach those blazingly fast perf numbers, you need C/CPP/Rust/Zig etc
at the cost of your sanity š
yeah, and readability š
Ill make a new post about some async clarification, thanks for the help and sorry OP for bloating your topic š
thats what I like about C#, its very fast for what it is but still maintains good readability
yeah, though I dont like some of it
like the
it looks so odd in a class, especially for people that just started with C# its weird
expression bodies?
they are ā¤ļø
they are fire ye, theyre nice syntax sugar to have
but yeah, there is... a lot to take in for someone new to the language
but its such an odd thing to see for the first time
I also dont like Nullable types
O_?
heathen
the
?
, id prefer Nullable<T>
being the only option
so its more clearI'm preparing for a programming contest so hopefully they allow LINQ lol
I shared a non LINQ solution to that one problem :D
saved that too, ty btw
wouldn't be backwards compatible, which ruled that out quickly.
np bro
we are forced to use this syntax since there are no difference for nullable reference types and non-nullable reference types for CLR
all null checks are just compiler checks, nothing else
expect some metadata provided by attributes
forced to use the
?
syntax?yes
because it makes sense only for compiler
remember, the alternative to
?
was not having it at allwhile Nullable<T> is a generic type
ie, all reference types being nullable
I mean doesnt
?
yield Nullable<T>
to the compiler?no
only for value types
thats so illegal
what
T cannot handle reference types?
because all reference types in CLR are nullable historically
reference types are already nullable
ah
they always are
wtf
thats bad
yeah
by definition
legacy
a reference can point to null, thus they are nullable
backwards compatibility is a blessing tot he industry and a bottleneck to a programming's development team
sry, its not, its a wrapped struct
very yes
imagine being able to remove null as a concept in modern C#
ā¤ļø
legal, but you will get a warning in modern C#
because nullability checks are on by default
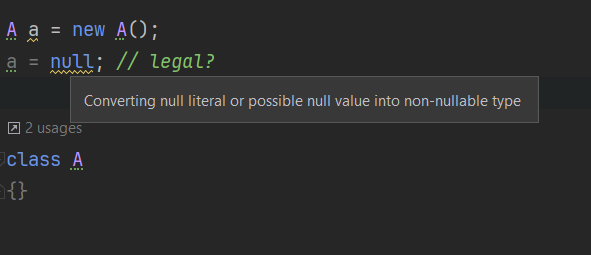
in some modern languages like Dart 3 there is something called "sound null safety"
but they had to rewrite almost all their libraries from Dart 2 to bring this feature
C# is much older and it will be impossible
+ reflection
so, all reference types will be nullable in the near future
Interesting, so they solved this issue by introducing
?
that would be universal to both reference and value types
I really dislike the whole value types ideology either way, I prefer just specifying when something is a reference on any types and not having a set default for a type
What's the upside to having certain types be a reference type, by default?
but lets say you do: Nullable<ClassType> obj
, that'd be considered redundant then no?
it's already implicitly nullable?it is not legal
you can't,
Nullable<T>
is a struct and has a where T: struct
requirementsince Nullable constrained to value types
oh they made it illegal, thats rough
so when you do
ClassType? obj
it just omits the ?
since its already nullable?yes
exactly
and emits some attributes with metadata about nullability
on properties, fields, parameters, etc...
where this type was used
that is nasty, thats why no one writes Nullable<T>, you'd create inconsisent code style between reference and value type
so you just opt for
?
and let the compiler handle it for youyes
yikers, thats sad. I find
?
pretty ugly sadly
oh well makes sense, thanks you two!can you imagine the use of interfaces (or, in common, abstract types) if any type can be both: value and reference just depending on how it is used?
I dont see how that'd implicate things honestly
I mean C++ has abstract classes, I can create instances of its child classes and they are value types by default
I can create a reference to that value type, an alias so to say
its dangerous because you can have a dangling reference, much like an invalidated pointer
I guess that's where it differs, these types are inherently a certain types, e.g value type or reference type
the main idea is that any reference type is always represented in memory as a reference with fixed size and it allows to consider them more abstract regardless their actual types
we can use interfaces (or abstract types) as properties, fields, parameters and we don't care about the actual types and their sizes in the memory
so what ure saying is that when you create a class instance
it creates the instance on the heap (I supposed, since we are using
new
)
on the stack we got a reference to the object
and that we pass/use
but the actual object isnt directly accessed by us, the programmer?yep
bruh
so its just heap allocation with pointers, conceptually, but applied with references
which is good in C# since we dont want them to manage memory anyway, we got a garbage collector
I guess its a good solution since you dont want the programmer to work with pointers in C#, unless its unsafe code block.
yes
but there are also a value types here to reduce the pressure on GC in places where it makes sense
simply there are primitives like numbers and bools
are value types always on the stack, or is that up to the compiler?
for example in python all numbers are placed in heap lol
depending on its size etc
I suppose it'd always be on the stack
always
ye, otherwise the distinction between ref and value types
would make no sense
and serve no purpose
can I manually
allocate it on the heap, a value type, lets say a
struct
you can box it
oh right
i forgot about that
or place as a field of the class (reference type)
"wrap it"
boxing powa
b would be a reference type, it references the heap obj thats now on the heap
yep
it doesnt invalidate
a
?no
you have move semantics?
a is still on the stack
yes
it just copies?
yes
it is not rust š
can I
std::move
the a
to another stack object for instanceyou can use ref i think
which would invalidate
a
since its moved to b
in parameters
would that invalidate the original object?
but it will not invalidate something
there are no such a concept in C#
i see
makes sense
it might just utilize smth like construct in place
that avoids unneeded copies
then I suppose
wouldnt this put it on the heap?
basically create an object in the heap and pass the reference?
no
it will pass the reference to the stack
where
a
is locatedmakes sense
otherwise it would suck lol
sure
heap allocation is expensive
is there a big difference between stack and heap allocation
in C#
it is the only reason while
ref
exists
in order not to box the value to just transfer it to the calling methodyeah, just allow references to value types
and ure good to go
not bad actually not so disappointed anymore in C#'s choices
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.