How to use volume with nextjs?
I am trying to persist image on volume.
RAILWAY_VOLUME_MOUNT_PATH =/images
I've tried to implement the change for the railway volume but without success.
This is the api route for local storage on /public/upload_images
Solution:Jump to solution
The file is being saved now. I've also created an new endpoint to fetch the content from the volume, following the same approach.
```ts
options.uploadDir = process.env.RAILWAY_VOLUME_MOUNT_PATH ?
process.env.RAILWAY_VOLUME_MOUNT_PATH : path.join(process.cwd(), "/public/upload_images");...
28 Replies
Project ID:
e46bd0a0-4466-4489-a2d3-6355d062c901
e46bd0a0-4466-4489-a2d3-6355d062c901
where are you storing your images on
/images
? <:mommy_confused:1023749002069540935>
if your mount path is /images
then you need to store the images thereI tried that already
I've tried like this:
any errors?
Only when trying to access the image

and disk usage didn't change
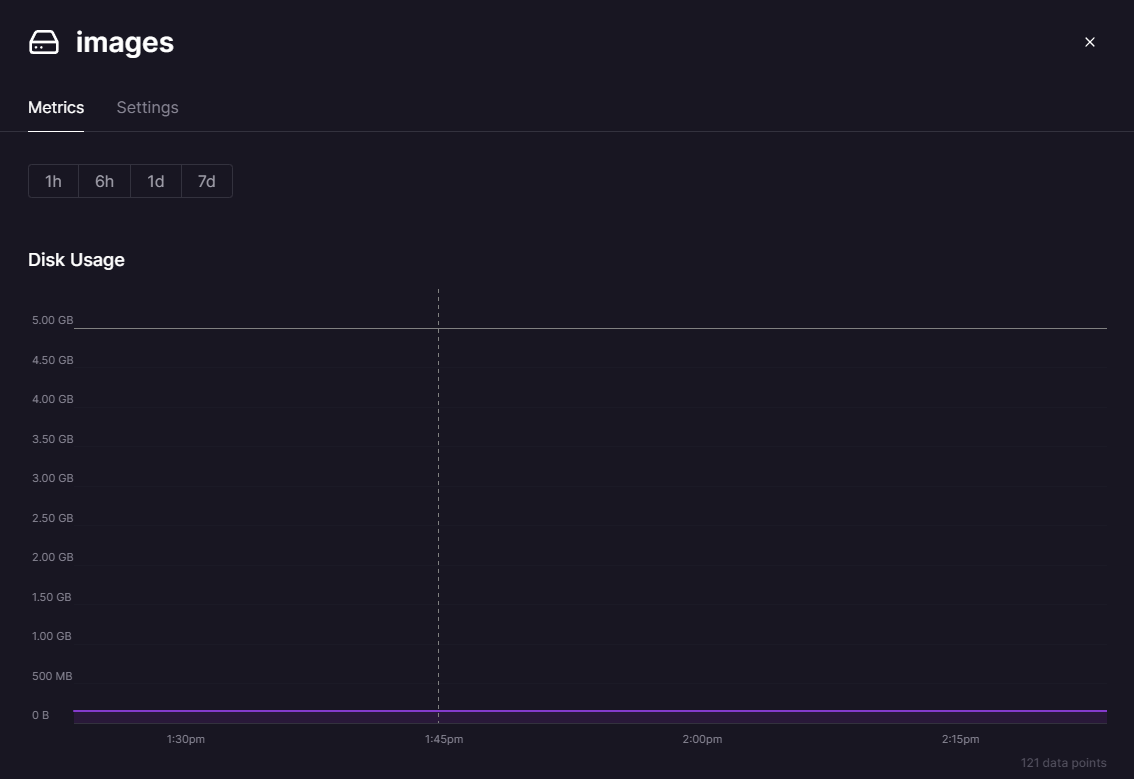
why is your volume mount path on /images and the dir ur trying to access is /public/upload_images?
isnt your volume path going to be the same?
I've tried to do that. I used the second snippet I've sent
here
i remember someone having the same issue but i can't find the thread
I've been searching aswell but nothing about this :/
wild guess but try uploading at
/app/RAILWAY_VOLUME_MOUNT_PATH/uploads
i'll try that
if that doesn't work we better wait for a specialist (brody)

Ahahah, let me try without the /uploads
see if it detects the path
dayum, it wasnt supposed to be this hard to use a volume storage lmao
xD
Solution
The file is being saved now. I've also created an new endpoint to fetch the content from the volume, following the same approach.
Ok, apparently the image is being saved. I've added a new endpoint to fetch the image content from the volume!
If no futher info is needed, this ticket can be closed 🙂
thanks
you can close it by doing this
@RUNNb make sure the images are still there between deployments, it would be easy to save a file into the container and lose it on the next deployment
but seeing the disk usage change on the volume it would mean they are going to the volume right?
..not really, nextjs can use that volume too (idk if it does tho)
better test redeploying ur service and seeing if they are still there
okok
also, be careful to not ping conductors or team #🛂|readme 5)
oh ok sorry