Unknown Interaction
I keep reaching the Unknown Interaction error as showed in the picture. Do note that I have a defer on each file as I am using ephemeral.
const { Client, Collection, Events, GatewayIntentBits, PermissionsBitField, ActivityType } = require('discord.js');
const { getDatabase, ref, set, child, get, remove, update, increment } = require('firebase/database');
module.exports = {
name: Events.InteractionCreate,
async execute(interaction) {
if (!interaction.isChatInputCommand()) return;
const command = interaction.client.commands.get(interaction.commandName);
if (!command) {
console.error(`The command "${interaction.commandName}" does not exist!`);
return;
};
const botMember = interaction.guild.members.cache.get(interaction.client.user.id);
if (!botMember.permissions.has(PermissionsBitField.Flags.Administrator)) {
interaction.reply({ content: 'Bot is missing the `ADMINISTRATOR` permission!', ephemeral: true });
return;
};
try {
await command.execute(interaction, interaction.client, interaction.user.username, ref(getDatabase()));
} catch (error) {
console.error(`Failed to execute "${interaction.commandName}"!`);
console.error(error);
}
},
};
const { Client, Collection, Events, GatewayIntentBits, PermissionsBitField, ActivityType } = require('discord.js');
const { getDatabase, ref, set, child, get, remove, update, increment } = require('firebase/database');
module.exports = {
name: Events.InteractionCreate,
async execute(interaction) {
if (!interaction.isChatInputCommand()) return;
const command = interaction.client.commands.get(interaction.commandName);
if (!command) {
console.error(`The command "${interaction.commandName}" does not exist!`);
return;
};
const botMember = interaction.guild.members.cache.get(interaction.client.user.id);
if (!botMember.permissions.has(PermissionsBitField.Flags.Administrator)) {
interaction.reply({ content: 'Bot is missing the `ADMINISTRATOR` permission!', ephemeral: true });
return;
};
try {
await command.execute(interaction, interaction.client, interaction.user.username, ref(getDatabase()));
} catch (error) {
console.error(`Failed to execute "${interaction.commandName}"!`);
console.error(error);
}
},
};
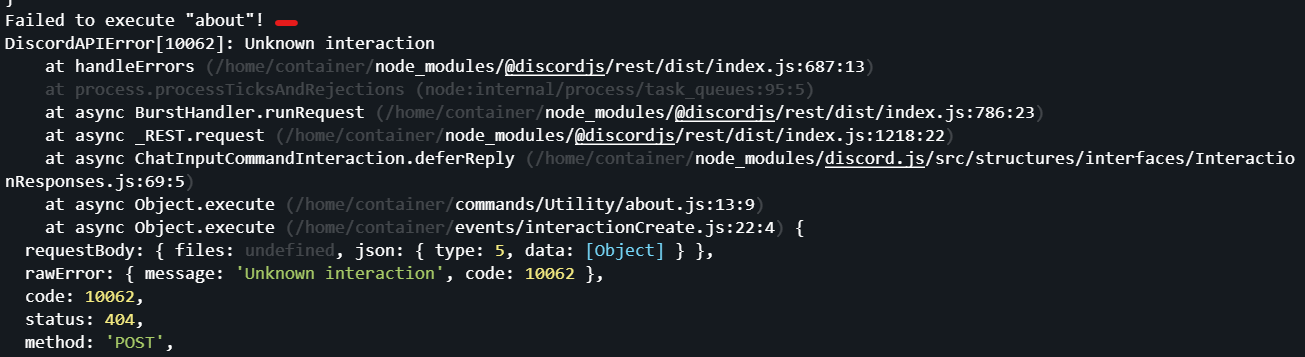
6 Replies
- What's your exact discord.js
npm list discord.js
and node node -v
version?
- Not a discord.js issue? Check out #other-js-ts.
- Consider reading #how-to-get-help to improve your question!
- Explain what exactly your issue is.
- Post the full error stack trace, not just the top part!
- Show your code!
- Issue solved? Press the button!
- ✅
Marked as resolved by OPTag suggestion for @DaRealGandhi20:
Common causes of
DiscordAPIError[10062]: Unknown interaction
:
- Initial response took more than 3 seconds ➞ defer the response *.
- Wrong interaction object inside a collector.
- Two processes handling the same command (the first consumes the interaction, so it won't be valid for the other instance)
* Note: you cannot defer modal or autocomplete value responsesWhat´s about command code?
try:
main
about
const { Client, Collection, Events, GatewayIntentBits, PermissionsBitField, ActivityType } = require('discord.js');
const { getDatabase, ref, set, child, get, remove, update, increment } = require('firebase/database');
module.exports = {
name: Events.InteractionCreate,
async execute(interaction) {
if (!interaction.isChatInputCommand()) return;
await interaction.deferReply({ ephemeral: true });
const command = interaction.client.commands.get(interaction.commandName);
if (!command) {
console.error(`The command "${interaction.commandName}" does not exist!`);
return;
};
const botMember = interaction.guild.members.cache.get(interaction.client.user.id);
if (!botMember.permissions.has(PermissionsBitField.Flags.Administrator)) {
interaction.editReply({ content: 'Bot is missing the `ADMINISTRATOR` permission!', ephemeral: true });
return;
};
try {
await command.execute(interaction, interaction.client, interaction.user.username, ref(getDatabase()));
} catch (error) {
console.error(`Failed to execute "${interaction.commandName}"!`);
console.error(error);
}
},
};
const { Client, Collection, Events, GatewayIntentBits, PermissionsBitField, ActivityType } = require('discord.js');
const { getDatabase, ref, set, child, get, remove, update, increment } = require('firebase/database');
module.exports = {
name: Events.InteractionCreate,
async execute(interaction) {
if (!interaction.isChatInputCommand()) return;
await interaction.deferReply({ ephemeral: true });
const command = interaction.client.commands.get(interaction.commandName);
if (!command) {
console.error(`The command "${interaction.commandName}" does not exist!`);
return;
};
const botMember = interaction.guild.members.cache.get(interaction.client.user.id);
if (!botMember.permissions.has(PermissionsBitField.Flags.Administrator)) {
interaction.editReply({ content: 'Bot is missing the `ADMINISTRATOR` permission!', ephemeral: true });
return;
};
try {
await command.execute(interaction, interaction.client, interaction.user.username, ref(getDatabase()));
} catch (error) {
console.error(`Failed to execute "${interaction.commandName}"!`);
console.error(error);
}
},
};
const { SlashCommandBuilder, EmbedBuilder, PermissionsBitField, ModalBuilder, ActionRowBuilder, ButtonBuilder, ButtonStyle } = require('discord.js');
const { getDatabase, ref, set, child, get, remove, update, increment } = require('firebase/database');
const noblox = require("noblox.js");
const randomstring = require("randomstring");
const request = require('request');
module.exports = {
data: new SlashCommandBuilder()
.setName('about')
.setDescription('Info about bot')
.setDMPermission(false),
async execute(interaction, client, EmbedRBXUsername, DatabaseDownload) {
let dbinfo = "No database data found!";
let verinfo = "No verification data found!";
let subinfo = "No subscription data found!";
try {
const guildSnapshot = await get(child(DatabaseDownload, `GuildsDatabase/${interaction.guild.id}`));
if (guildSnapshot.exists()) {
dbinfo = `**Moderator:** <@&${guildSnapshot.child("modrole").val()}>\n**Data Viewer:** <@&${guildSnapshot.child("dataviewerrole").val()}>\n**Data Manager:** <@&${guildSnapshot.child("datamanagerrole").val()}>\n**Schedule Manager:** <@&${guildSnapshot.child("schedulemanagerrole").val()}>\n**Suggest Channel:** <#${guildSnapshot.child("suggestchannel").val()}>\n**Schedule Updates:** <#${guildSnapshot.child("scheduleupdates").val()}>`;
}
const verifySnapshot = await get(child(DatabaseDownload, `VerifyDatabase/${interaction.member.id}`));
if (verifySnapshot.exists()) {
const userId = verifySnapshot.val();
const username = await noblox.getUsernameFromId(userId);
verinfo = `Roblox: \`${username}:${userId}\`\nDiscord: \`${interaction.user.username}:${interaction.user.id}\``;
const smpSnapshot = await get(child(DatabaseDownload, `SideModPlus/${userId}`));
if (smpSnapshot.exists()) {
if (smpSnapshot.val() < Math.floor(Date.now() / 1000)) {
subinfo = `**SideMod Plus:** Expired!`
} else {
subinfo = `**SideMod Plus:** <t:${smpSnapshot.val()}>`
}
}
}
} catch (error) {
return interaction.editReply({ content: 'An error occurred. Please try again later.', ephemeral: true });
}
const Embed = new EmbedBuilder()
.setColor(0x8645b0)
.setTitle(`About ${client.user.username}`)
.setAuthor({ name: `${client.user.username}`, iconURL: `${client.user.avatarURL({ dynamic: true })}`, url: 'https://discord.gg/bhsXPb2U2M' })
.setFooter({ text: EmbedRBXUsername })
.setDescription(`${client.user.username} is a robust moderation system with a Points System, Schedule System, and its own API for easy integration.`)
.setThumbnail(`${client.user.avatarURL({ dynamic: true })}`)
.setTimestamp()
.addFields(
{ name: 'Verification Info', value: verinfo, inline: false },
{ name: 'Subscription Info', value: subinfo, inline: false },
{ name: 'Database Info', value: dbinfo, inline: false }
);
return interaction.editReply({ embeds: [Embed], ephemeral: true })
},
};
const { SlashCommandBuilder, EmbedBuilder, PermissionsBitField, ModalBuilder, ActionRowBuilder, ButtonBuilder, ButtonStyle } = require('discord.js');
const { getDatabase, ref, set, child, get, remove, update, increment } = require('firebase/database');
const noblox = require("noblox.js");
const randomstring = require("randomstring");
const request = require('request');
module.exports = {
data: new SlashCommandBuilder()
.setName('about')
.setDescription('Info about bot')
.setDMPermission(false),
async execute(interaction, client, EmbedRBXUsername, DatabaseDownload) {
let dbinfo = "No database data found!";
let verinfo = "No verification data found!";
let subinfo = "No subscription data found!";
try {
const guildSnapshot = await get(child(DatabaseDownload, `GuildsDatabase/${interaction.guild.id}`));
if (guildSnapshot.exists()) {
dbinfo = `**Moderator:** <@&${guildSnapshot.child("modrole").val()}>\n**Data Viewer:** <@&${guildSnapshot.child("dataviewerrole").val()}>\n**Data Manager:** <@&${guildSnapshot.child("datamanagerrole").val()}>\n**Schedule Manager:** <@&${guildSnapshot.child("schedulemanagerrole").val()}>\n**Suggest Channel:** <#${guildSnapshot.child("suggestchannel").val()}>\n**Schedule Updates:** <#${guildSnapshot.child("scheduleupdates").val()}>`;
}
const verifySnapshot = await get(child(DatabaseDownload, `VerifyDatabase/${interaction.member.id}`));
if (verifySnapshot.exists()) {
const userId = verifySnapshot.val();
const username = await noblox.getUsernameFromId(userId);
verinfo = `Roblox: \`${username}:${userId}\`\nDiscord: \`${interaction.user.username}:${interaction.user.id}\``;
const smpSnapshot = await get(child(DatabaseDownload, `SideModPlus/${userId}`));
if (smpSnapshot.exists()) {
if (smpSnapshot.val() < Math.floor(Date.now() / 1000)) {
subinfo = `**SideMod Plus:** Expired!`
} else {
subinfo = `**SideMod Plus:** <t:${smpSnapshot.val()}>`
}
}
}
} catch (error) {
return interaction.editReply({ content: 'An error occurred. Please try again later.', ephemeral: true });
}
const Embed = new EmbedBuilder()
.setColor(0x8645b0)
.setTitle(`About ${client.user.username}`)
.setAuthor({ name: `${client.user.username}`, iconURL: `${client.user.avatarURL({ dynamic: true })}`, url: 'https://discord.gg/bhsXPb2U2M' })
.setFooter({ text: EmbedRBXUsername })
.setDescription(`${client.user.username} is a robust moderation system with a Points System, Schedule System, and its own API for easy integration.`)
.setThumbnail(`${client.user.avatarURL({ dynamic: true })}`)
.setTimestamp()
.addFields(
{ name: 'Verification Info', value: verinfo, inline: false },
{ name: 'Subscription Info', value: subinfo, inline: false },
{ name: 'Database Info', value: dbinfo, inline: false }
);
return interaction.editReply({ embeds: [Embed], ephemeral: true })
},
};
Um btw I have some ephmeral: false on some files too so I don't think that's going to work.
oh I see