❔ For loop is being skipped after a do while loop.
Hello, Im trying to build a simple connect four game in console and I've come across an issue where my for loop is being skipped but the if statement is being reached.
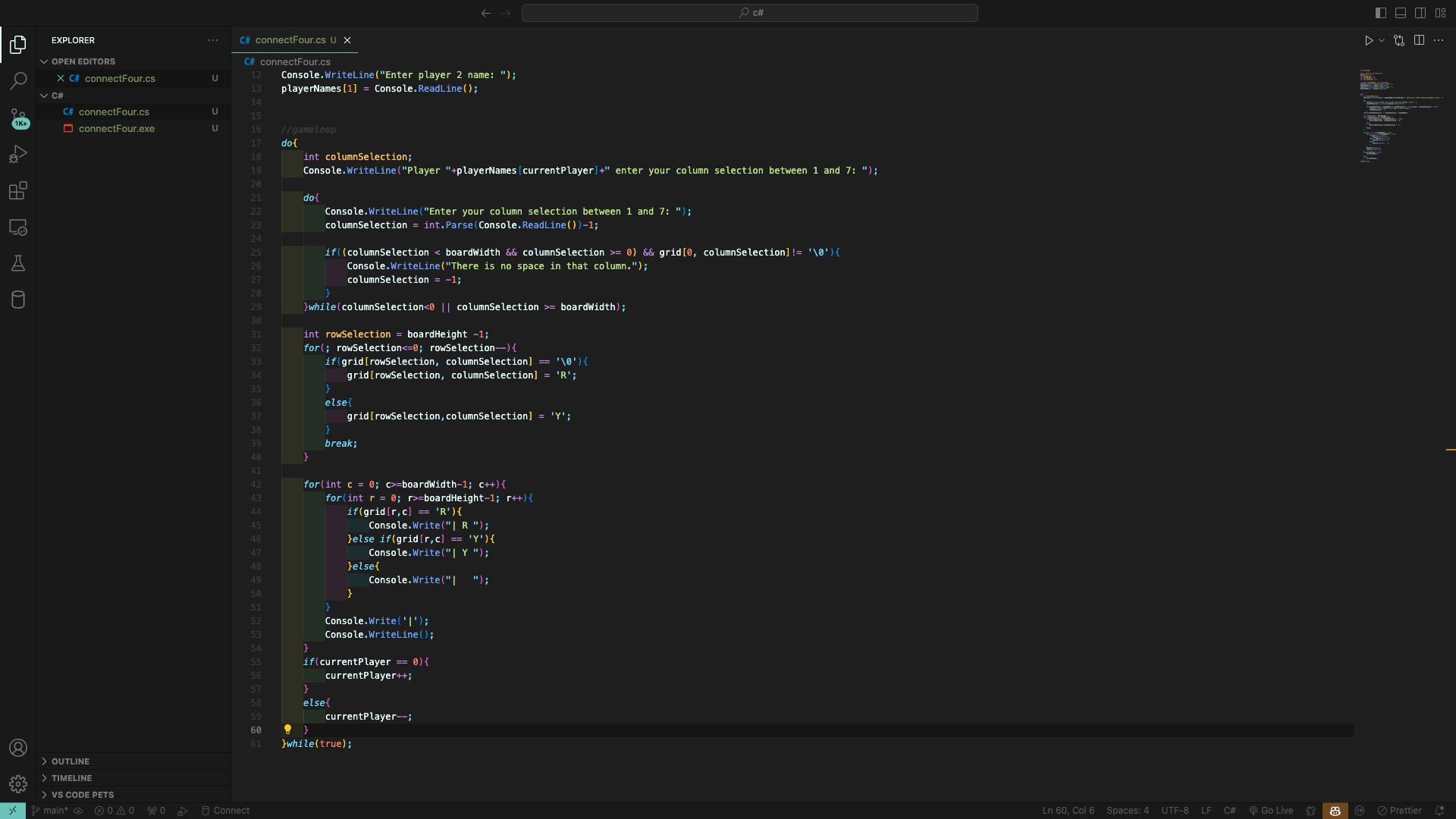
33 Replies
Both of the for loops are being skipped after the do while at the beginning of the game loop
please share your code rather than a screenshot of your code
even better if you can upload to github that would be nice
$code
To post C# code type the following:
```cs
// code here
```
Get an example by typing
$codegif
in chat
For longer snippets, use: https://paste.mod.gg/Oh right sorry about that.
Step through the code in a debugger
Useful skill to learn
Also that code formatting is something else 😄
Yeah I've been doing that but it still just skips the code without any reason.
"without any reason" is going to turn out to be an incorrect statement....
Not entirely sure why it ended up like that ill be honest haha
Yeah maybe I should rephrase to "I have yet to find the reason its doing that and I might just be blind."
Do you have a build warning that says "unreachable code detected" ?
(That's a rhetorical question)
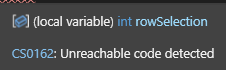
Yes.
haha
I put a print statement jsut after the do while loop in the game loop and that doesnt seem to output to the console.
it still just skips the code without any reasonYou must be confused about what is happening. what code do you believe is being skipped for no reason?
I always run with warnings as errors enabled so for me this code wouldn't even build.
The 2 for loops that are after the do while inside the game loop seems be getting skipped and im not sure why.
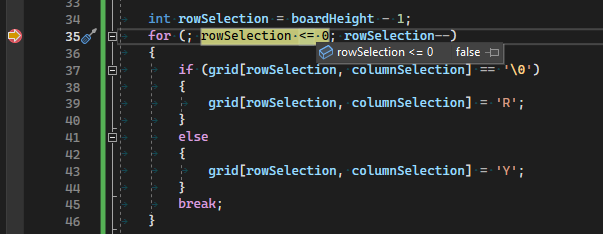
Nevermind the print statements work i just forgot semi colons haha
if you put a break point you will see why
rowSelection is 5, which is greater than 0
rowSelection will never be less than or equal to zero so this loop will never execute.
Is that supposed to be >=
Then question is why is the second loop being skipped? Or is that being caused because the first one wont be executed
nothing is being "skipped". code will do exactly what you tell it to. you have told your code not to run the loop if
rowSelection
is greater than 0, which it is
This will also never execute.
Ah yes, I see what you mean. Thank you very much!
Because this says "run this loop while c is greater than or equal to boardWidth - 1", but on the first iteration of the loop c is zero so this loop condition is never satisfied.
also, I have a version of connect 4 on GitHub if you would like to see it.
not trying to discourage you from coding your own, but if you want to see another person's code just ask and I can link it
Oh right so do I need to change the condition for it to be less than the boardWidth-1 so it satisifies it?
That would be helpful thank you! Ill try and keep coding it myself but it would be nice to see how other people have gone about it.
https://github.com/dotnet/dotnet-console-games it is one of the games in here 🙂 you can also play it online so you don't have to download and compile it if you don't want to
What do you think? 🙂
It might help to remind yourself that a
for
loop is just syntactic sugar to make writing a while
loop a bit less verbose.
This basically translates to the following:
Haha if only i could understand the code, I'm pretty new to this so its needing a bit of practice before i can understand. Either way thank you very much the both of you!
Oh right i see, so the condition in the middle needs to be satisfied for the for loop to continue
if you do look at it and have questiosn feel free to ping me
That makes sense why the for loops seemed as if they were being skipped when they just werent being satisfied at all.
Yes, in a
for
loop and in a while
loop the loop never executes if the condition is not satisfied.
In a do-while
loop it always executes at least once because the condition is checked at the end of the loop instead of the beginning.Thank you!
Oh right now I understand, thank you very much for clarifying.
That makes sense why a game loop uses a do while rather than just a while as well.
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.