Editing interaction message gives an error
I am making a queue system for my discord bot and I have a command where you do it sends a message and a join queue button attached to the message. If you click that button it edits the original message and adds: "Queue:
member.user.tag
" Then it also sends you an ephemeral message letting you know that you joined, attached to that ephemeral message is a Leave Queue button that if you click it is supposed to edit the original "Queue: member.user.tag
" and remove member.user.tag
from it. But it errors when I do interaction.message.edit({content: queueMessage,});
in leaveQueue.
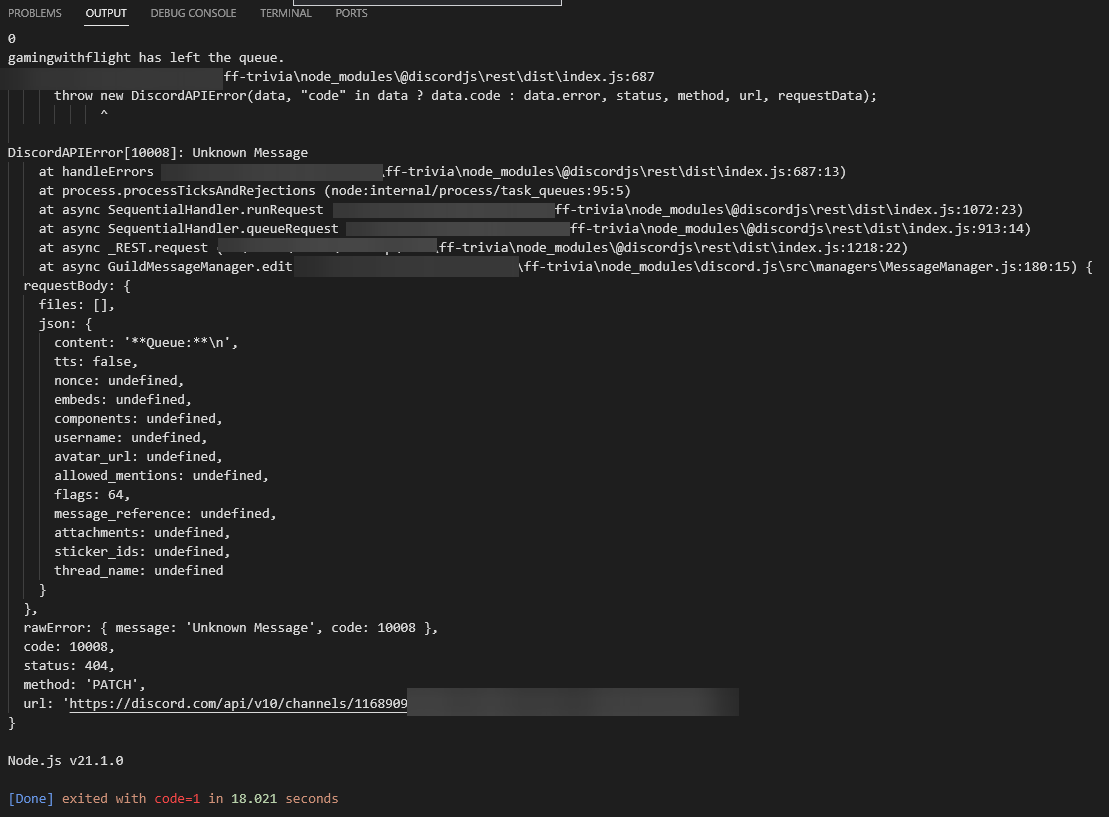
8 Replies
- What's your exact discord.js
npm list discord.js
and node node -v
version?
- Not a discord.js issue? Check out #other-js-ts.
- Consider reading #how-to-get-help to improve your question!
- Explain what exactly your issue is.
- Post the full error stack trace, not just the top part!
- Show your code!
- Issue solved? Press the button!
- ✅
Marked as resolved by OPVersions:
You have to call editReply on the original interaction
ephemeral messagez cannot be edited like that
How would I get the original interaction? I am not trying to edit the ephemeral message, I'm trying to edit the original message.
You can use interaction.update
I did:
Is this solution fine?
did you reply to the interaction before?
Yea I replied when they clicked the join queue button: