Different modal IDs still trigger awaitModalSubmit multiple times
I need the user to click the button and enter feedback in the modal. After submission, update the embeds in the original message and another notify message . However, if the user closes the modal midway and then re-clicks the button and submits, awaitModalSubmit will resolve multiple times, resulting in multiple updates. How to avoid this situation?
Here are the output after open modal three times:
[01:58:03.598] DEBUG (27560): 1171388086941405195
[01:58:06.284] DEBUG (27560): 1171388098911948840
[01:58:08.420] DEBUG (27560): 1171388107808055337
[01:58:09.937] INFO (27560): Submission Approved!
Status updated from In Review to Complete
Feedback: 123
[01:58:09.937] INFO (27560): Submission Approved!
Status updated from In Review to Complete
Feedback: 123
Feedback: 123
[01:58:09.937] INFO (27560): Submission Approved!
Status updated from In Review to Complete
Feedback: 123
Feedback: 123
Feedback: 123
[01:58:10.117] DEBUG (27560): Unknown interaction
[01:58:10.147] DEBUG (27560): Unknown interaction
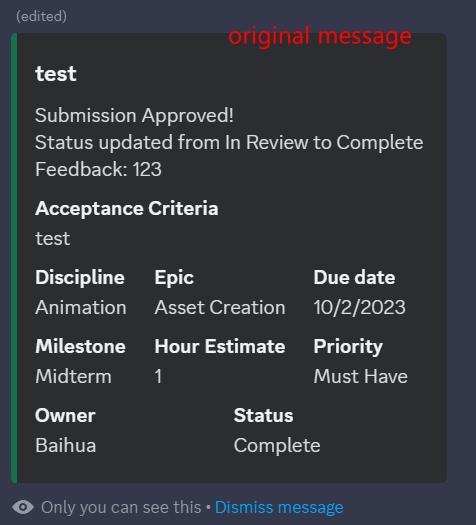
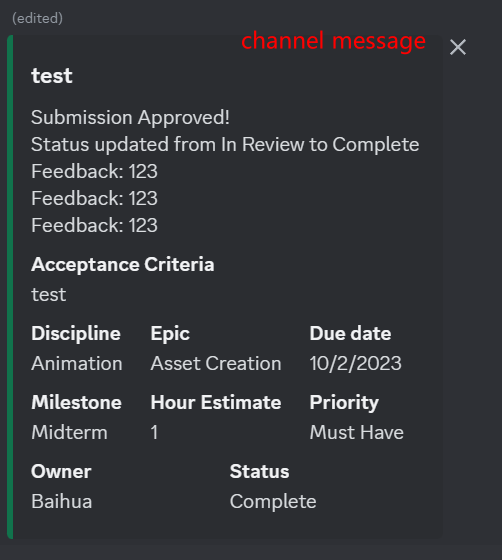
7 Replies
- What's your exact discord.js
npm list discord.js
and node node -v
version?
- Not a discord.js issue? Check out #other-js-ts.
- Consider reading #how-to-get-help to improve your question!
- Explain what exactly your issue is.
- Post the full error stack trace, not just the top part!
- Show your code!
- Issue solved? Press the button!
- ✅
Marked as resolved by OPHave different custom id for each modal or just handle modal submits in the
interactionCreate
event instead of collectors.I use ButtonInteraction id as modal id actually (.setCustomId(
feedback-${i.id}
)), it's different every time but awaitModalSubmit still resolve multiple times...It's not. As you said when user clicks the button 2 times it shows 2 models with the same custom id
I add a logger here:
it really gave me different modal ID:
[02:12:59.485] DEBUG (36040): feedback-1171391844618358805
[02:13:03.570] DEBUG (36040): feedback-1171391861789818991
[02:13:06.095] DEBUG (36040): feedback-1171391872426582026
Do I need to change other ID?
You could add a check for the matching id in the filter
It works! Thank you!