awaitMessages does not reject on timeout?
I've got the following simple code collecting a single message from a user:
Output upon waiting 3000 milliseconds:
Why is it that when the
awaitMessages()
times out, the then
statement is still running? Shouldn't it reject and go to the .catch
block?9 Replies
- What's your exact discord.js
npm list discord.js
and node node -v
version?
- Not a discord.js issue? Check out #other-js-ts.
- Consider reading #how-to-get-help to improve your question!
- Explain what exactly your issue is.
- Post the full error stack trace, not just the top part!
- Show your code!
- Issue solved? Press the button!
- ✅
Marked as resolved by OPI see someone posting something else here earlier today regarding
awaitMessages
but I don't think it is relevant.You have to use the
errors: ['time']
option to make it reject if time expired; otherwise, it still resolves the promiseOh say what? Really?
Why isn't that mentioned here?
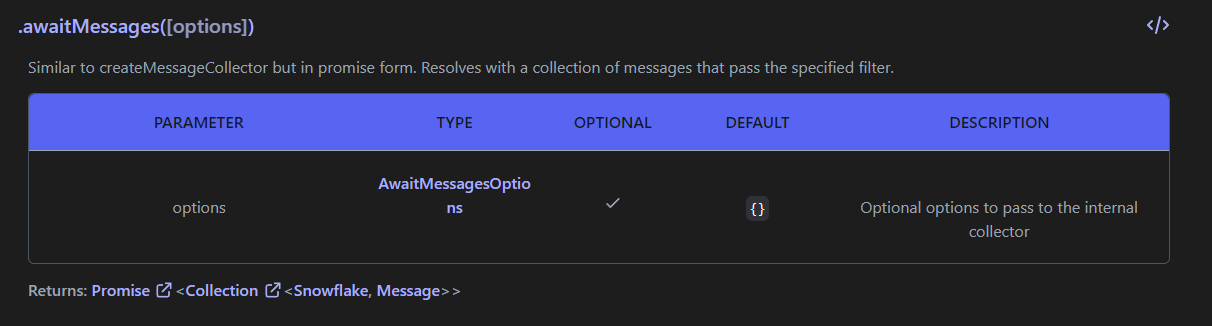
Huh, and why don't other functions like
awaitMessageComponent()
require that argument?Just different design decisions when that was introduced
Valid point to look at consistency though
I think the thing there was that time is the ONLY reason a message component collector could end
It either collects one component, or times out
That function only collects one interaction ever, so it unlike awaitMessages() which can return just an empty collection instead of a populated one, that collector's type would have to be changed depending on whether timeouts error since it would need to return null or something similar
awaitReactions works the same as awaitMessages since both can collect multiple of whatever structure, so awaitMessagecomponent is actually the odd one out
Gothca. Okay, well thanks for letting me know about the argument that needed to be passed! That solves my issue!