Absolute Beginner - Accessing Variables From Other Classes
Hi.
DecodeButton.cs
:
ReadStringInput.cs
:
In DecodeButton.cs
, I'm getting the error CS0120
about Object references but frankly I'm not understanding it. Can someone help me out?53 Replies
The exact error text is:
The error is telling you that you need an instance to access the
input
fieldOh right
The word
reference
was confusing me
Thank you!
I'll try it really quickly
I changed DecodeButton.cs
to this:
@🌈 Thinker 🌈 but i get a different error now
Just that readStringInput
doesn't exist
I'm guessing that this is because the IDE doesn't know whether Start() will be called before OnClick()?
But Unity still doesn't let me run the appYou're creating a variable
readStringInput
which is local to Start
, it's destroyed immediately after the method stops.But I can't really define it outside of the method, right?
yes you can
You did that with
input
Well isn't that a good point
So just?
Or do I include the
new()
bit as well
And then actually create the instance just before using ityep
Also about this, this is something called scope which determines where and how variables are accessible.
It's a pretty fundamental concept, so it's quite important to know about
Yeah I understand it from Python, just kinda forgot it here haha - still getting into the frame of mind needed for coding
Would it also work if I instantiated it within the scope of the entire class?
Yeah, and C# is quite different from Python
Oh yeah
Definitely
Quite cool though
Well yes, but that works a bit differently
How so?
Fields (variables in a class) cannot depend on each other, and you wouldn't be able to call any Unity methods from a field initializer.
So what could I use that for?
This wouldn't work
Ok
You set the value in
Start
or similarAnd that is because it cannot change? So that value must always be the same so it can't depend on what
GetComponent<Bar>().Value
actually is?not exactly
In field initializers, Unity methods do not work
Got it
You have to set the value of fields in Start or some other method
Though of course this is just in Unity
Yes
I understand
Not exactly why Unity methods don't work, though
Do you know what a constructor is?
Somewhat, yes
Called when the class is instantiated
yep
Sets some intial fields' values depending on parameters
That's about the extent of my knowledge on them
So field initializers are actually just put inside the class's constructor. Unity doesn't let you use constructors for
MonoBehavior
classes, for various reasons including multithreading (I believe). Constructors are called before the object has any information about its place in the scene, eg. what components it has, its transform, etc., and this prevents you from calling Unity methods in said constructor.That makes a lot of sense
Pretty logical to be fair
However
Start
is called after this initialization is complete, therefore you have to initialize fields from there.Got it
So
Start()
is effectively your constructor when using Unityyep
Okay
Thank you, that's actually quite useful to know
np 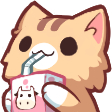
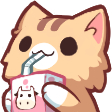
Really quick, I saw this thing in codecademy at school that's like if ? myBool x : y
Something vaguely resembling that
Like a really short if/else statement to set the value of a variable
And I've completely forgotten it
That's the one
Brilliant
Thank you
yeah, it's quite useful
Now I've just got to figure out how to restrict the contents of an Input Field in Unity to digits
Shouldn't be too hard
But knowing me I'll make some super complicated method for doing it when there's already a built-in function or something 😢
Hint: use
int.TryParse
Yep I'm using that rn
Just don't want to run that over every char every time the field is edited
I feel like Unity will have something in there that does it for me
@🌈 Thinker 🌈
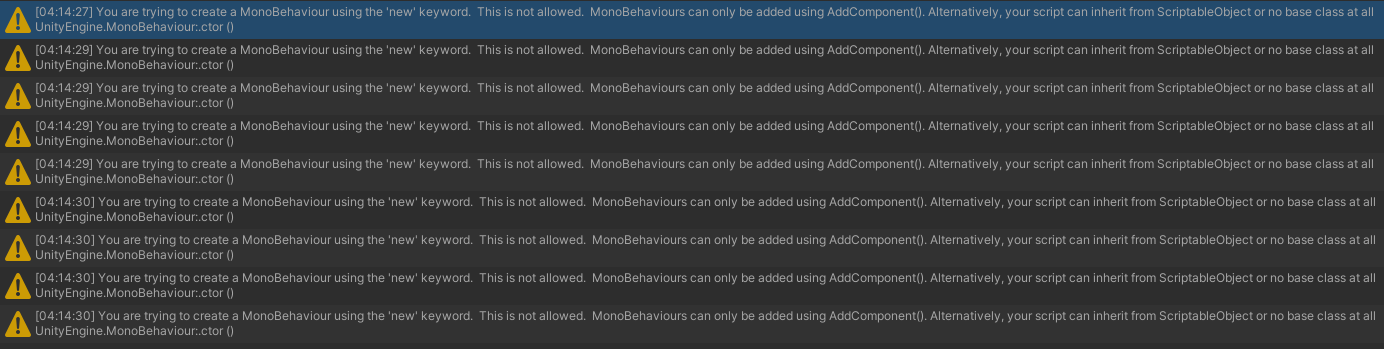
I'm now just getting a bunch of these errors
This is the script that is supposedly causing issues:
Unity Discussions
You are trying to create a MonoBehaviour using the 'new' keyword. ...
You are trying to create a MonoBehaviour using the ‘new’ keyword. This is not allowed. MonoBehaviours can only be added using AddComponent(). Alternatively, your script can inherit from ScriptableObject or no base class at all I am trying to connect these two scripts and I don’t know which one this error is for. The full error is: You...
Looking at this link, it suggests
MyScript script = obj.AddComponent<MyScript>();
However I don't understand the syntax
We're creating a script - where does it go? Is it a temporary file? Why are we adding a component?
Also isn't MonoBehaviour.Awake()
Unity's version of a constructor?I was doing some reading and found this:
https://docs.unity3d.com/ScriptReference/MonoBehaviour.Awake.html
Found the fix for this.
GitHub
CipherDecoderApp/Assets/Scripts at main · AdiZ-1579887/CipherDecode...
Adi, Karter, Kavya. Contribute to AdiZ-1579887/CipherDecoderApp development by creating an account on GitHub.
You can find it here if you're curious 🙂
Read what it says
Altho a bit late, I suggest watching tarodev's videos. Excellent resource and he also has a discord.
https://www.youtube.com/watch?v=tE1qH8OxO2Y
Tarodev
YouTube
Unity Architecture for Noobs - Game Structure
Unity Architecture is one of those things which can completely overwhelm new devs. This video will introduce you to a handful of architectural design patterns, which shy away from over-engineering, allowing you to learn the basics and get on with developing your game. Simple patterns are perfectly fine for most projects solo devs and small teams...
it covers basically everything for beginners. I am also learning from it
@Dharmang thank you, definitely needed something like this, I'll go check it out.