Collector buttons work but just once
Hello! I'm working on a leaderboard system that lets you swap through pages by clicking buttons as a menu of sorts. It seems to work fine enough the first time you click a button (with the slight annoyance that the three loading dots disappear way too early). By the second time something goes off, and the bot crashes with a
DiscordAPIError[10062]: Unknown interaction
error. Anyone got an idea of why that might be?
9 Replies
- What's your exact discord.js
npm list discord.js
and node node -v
version?
- Not a discord.js issue? Check out #other-js-ts.
- Consider reading #how-to-get-help to improve your question!
- Explain what exactly your issue is.
- Post the full error stack trace, not just the top part!
- Show your code!
- Issue solved? Press the button!
- ✅
Marked as resolved by staffUsing djs
14.14.1
and node 9.5.0
.
Error trace:
Demo, for bonus points. Takes forever because it needs to fetch usernames from an ID.
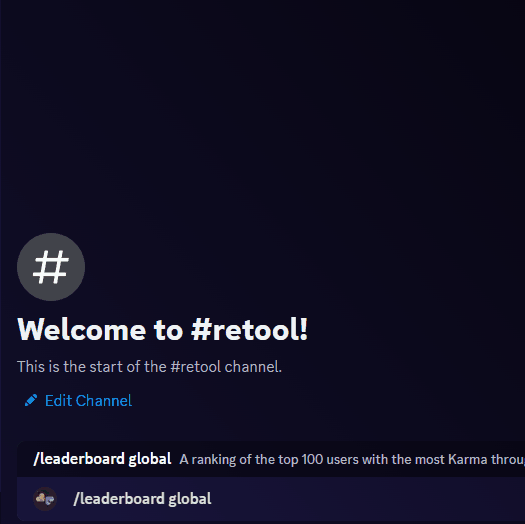
Nice use of fields features for your leaderboard
love it
Thank you thank youu!
Common causes of
DiscordAPIError[10062]: Unknown interaction
:
- Initial response took more than 3 seconds ➞ defer the response *.
- Wrong interaction object inside a collector.
- Two processes handling the same command (the first consumes the interaction, so it won't be valid for the other instance)
* Note: you cannot defer modal or autocomplete value responsesI might be missing something but I'm nto sure if any of these apply? The response is being deferred (it just crashes on the second go), and there are two interactions inside the function but they've got different names
Maybe I'm misunderstanding the third but the new interaction created by the collection is passed to the function that generates the embed and back to a newly created collection, so I'm not sure where the issue lies
If you are waiting for button or select menu input from a specific message, don't create the collector on the channel.
- Channel collectors return component interactions for any component within that channel.
Okay, so the good part is that the The not-so-great part is that I don't really have a message?
Hold on, I might be stupid -
Ayup, that makes sense. Had to base it on the message I'm supposed to be editing, had a bit of a brainfart there
But yeah, thank you! Would have never figured it out myself :)
max: 1
setting worked! Thanks a ton
interaction.message
returns null, it being a slash command and all - don't think I've got a choice but to use interaction.channel