PHP Type Coercion with echo(), data-type of output. Is my example correct?
I have some examples in the attachment picture here, and I just want to confirm that the data types that I think I'm 'coercing' into are actually correct.
For example,
Everything written in red in my attmt picture is the data-type that I think things are being coerced into.
echo(string) true
returns 1
. Here, I think that means that the returned 1
is a string data-type, and that it's a string data-type because of my 'string' argument.
Whereas, for another example, echo(int) 3.14
<-- here I'm coercing a float into an integer. So when this returns a 3
, I can assume that the 3
is an integer correct? Because I used the 'int' argument, right?Everything written in red in my attmt picture is the data-type that I think things are being coerced into.
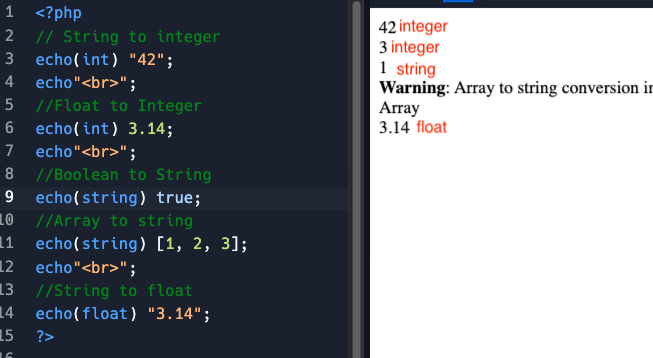
14 Replies
so
The '42' that is getting output here... Is it true, that because I'm using 'echo' to output it, it is actually outputting the '42' as a string?
even though $toInt actually has a data-type of integer?
IOW, outputting with 'echo' always reutrns a string, no matter what the variable's actual data-type was?
echo doesn't return anything, it prints strings to stdout
it'll take in any data, cast it to a string, and then output it to the standard output, either the console or apache/nginx
so
echo (int) "42";
is a bit nonsensical outside of testing and playing around, unless you want to exploit the change of value due to the casting to int and back to string, in which case you should really rewrite your logic to make that explicitSo what is the difference when we say we're 'outputting' (what echo does) versus 'returning' (which is not what echo does?)
output is a special thing echo and print (and a few others) do. returning is passing a value back to the calling context. If I have something like
$value = some_function();
, then some_function
's return value will be assigned to $value
okay so 'output' is in its own category, and not meant for performing operations or storing value, but only for quick viewing something, versuss 'returning' is for assigning values or performing operations
well, no, outputting includes things like rendering out templates. Basically, anything that leaves your script to the user's screen.
Returning is a language feature of all programming languages. It's when a function takes some data and passes it back to where the function was called. It doesn't really have anything to do with performing any operations, it's just that usually functions also perform operations and then return something
^ that function doesn't perform any operations, it just returns the string
Hello world!
to wherever it's called.
^ that function doesn't return anything, but it does perform an operationok, so by 'outputting', we're basically saying 'it doesn't have operations, but just returns a value'. ?
which is what echo is?
No, outputting in this context is just sending something to the standard output. It's not really different than updating a database or writing a file, it's pushing data somewhere else and that somewhere else gets to decide what happens with it.
Returning is a programming concept for passing data around inside your script. Outputting isn't a special type of returning or something, it's just a side effect of some functions
So outputting basically means 'print this', whereas returning refers to passing a value back to be usable in another function (put into the 'calling context').
These aren't mutually exclusive either, they can both occur in one function too, correct?
Yeah
so with
Would the function itself - greet - be considered outputting here too or is it considered to be implicitly returning a function, the echo, which is outputting?
Or do we just say it's 'outputting' since the echo inside of it is outputting and it doesn't have any other return?
I don't think that's a necessary distinction, and it depends on where you're looking at the code
echo does the outputting, but when you call greet, something gets sent to output too
ok