What is the max time for a Collect Interaction?
I have a Discord bot that automatically sends the status of the translations we have for a specific app, with an Update button attached to it.
what is the max time that I can pass for the collector component, as it seems that I'm unable to make use of 24 hours <_<
See the following line in the following code snipper:
const collector = message.createMessageComponentCollector({ filter, time: 86390999 });
(Also other tips are welcome)
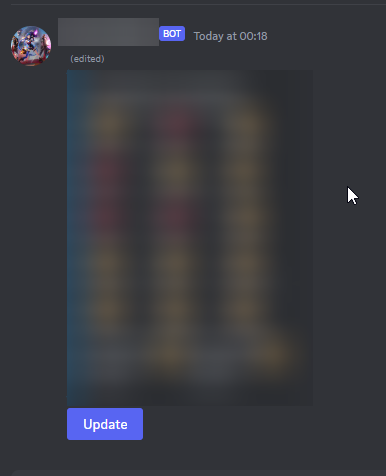
3 Replies
- What's your exact discord.js
npm list discord.js
and node node -v
version?
- Not a discord.js issue? Check out #other-js-ts.
- Consider reading #how-to-get-help to improve your question!
- Explain what exactly your issue is.
- Post the full error stack trace, not just the top part!
- Show your code!
- Issue solved? Press the button!Just omit the time
Then it'll run untill your bot restarts
Or just use the interactionCreate event
store data with id (generate a random id)
then attach the generated id to button custom_id
and handle the data with interactionCreate event