Felte Form server error handling
Hello. I'm having trouble displaying errors from the server with a Felte form. According to the docs "You can add an onError function to the createForm configuration that will be called if the onSubmit function throws an error." I've added the onError function but I'm not getting the errors from the onSubmit function.
My form looks like this:
And the error handling part of my server looks like this:
I get the 400 status code back (see screenshot). But no console.log from the onError function
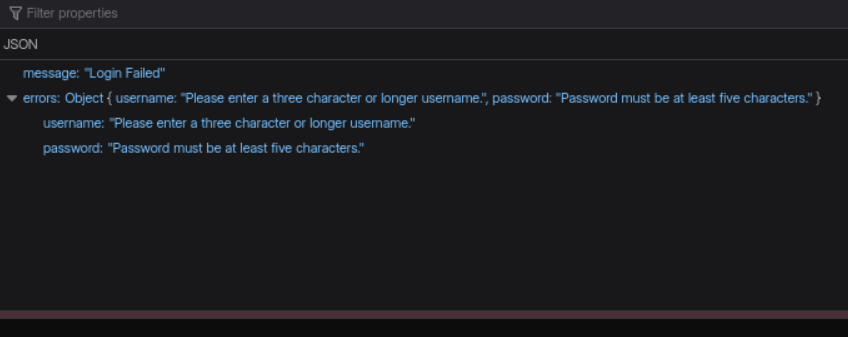
10 Replies
This is the form element. errors I'm tyring to display are commented out. Left out the client side checks because I'm trying to make sure server side checks work too and they aren't 😦
and I'm trying different things in that code to displau the errors
I am making progress
Curious but I have to task, does
createForm
support async callbacks?I saw an example of someone using it but I'm not sure. I gave up on Felte and did a regular form based off this example which uses solidJS (https://docs.astro.build/en/recipes/build-forms-api/) and it works fine. I feel like Felte should be used by more experienced people until they add more to their docs
Okay I get it now
You're expecting 400 responses to throw
Yes the 400. Because I didn't put in a username or password
It doesn't behave that way because technically speaking, 400 responses is a "successful" response.
You might want to do
The only way a
fetch
call will throw an error is if it cannot connect to the server. Otherwise, a successful response will not throw, regardless of the status codeThis would go in the try block on client side I think?
didn't realize a 400 wouldn't trigger the onError function of Felte forms. I was hoping I could get the errors from the server and display them on the page.....maybe that is pointless if there's client side validation
I mean if you have
then Felte would see it as a successful response
however if you have
then that fits what you want
This is not a Felte behavior, just a
fetch
concept
A common misconception specially from people who used axios in the pastI see. Maybe I'll go back to Felte and try it. Thanks
It's kind of weird that questions from a discord wind up on a website: https://www.answeroverflow.com/m/1182743517047562300
Felte Form server error handling - SolidJS
Hello. I'm having trouble displaying errors from the server with a Felte form. According to the docs "You can add an onError function to the createForm configuration that will be called if the onSubmit function throws an error." I've added the onError function but I'm not getting the errors from the onSubmit function.
My form looks like this:
`...