TypeError: Cannot read properties of null (reading 'useState')
TypeError: Cannot read properties of null (reading 'useState')
I am using the stock t3 app with next auth. However, when trying to setup a custom login page I get an error regarding the useState implementation. Can anyone tell me why this may be happening. I tried all I could think of, looked on stackoverflow but nothing resolves the issue.
Any help would be greatly appreciated.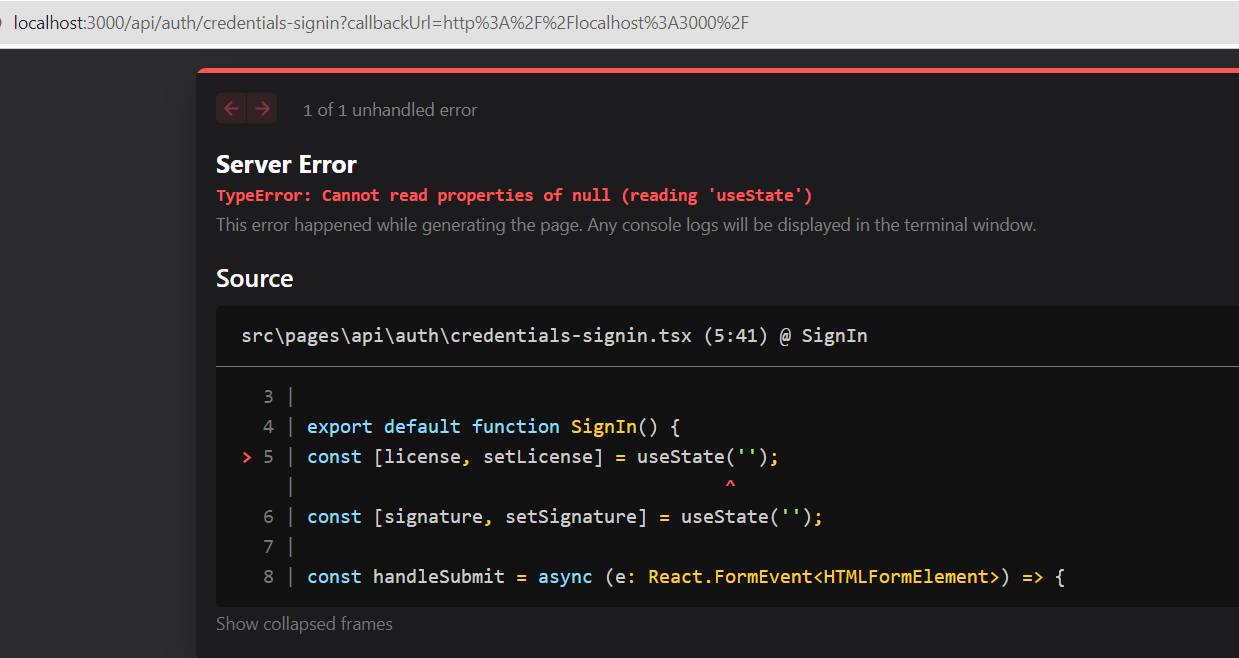
3 Replies
src/pages/api/auth/credentials-signin.tsx
You can’t use react in your API folder directory. The API folder is for the backend and since react is runs on the client it cant be used in the API folder
Routing: API Routes | Next.js
Next.js supports API Routes, which allow you to build your API without leaving your Next.js app. Learn how it works here.