Generating Access Tokens
I'm using the Kinde Management API. I've run into an issue where the Bearer Token (access token) I've generated in Postman expires after 24 hours.
I understand that I can solve this by generating a new one for each API request that I want to perform, but I cannot figure out how to do so.
The documentation (https://kinde.com/docs/build/get-access-token-for-connecting-securely-to-kindes-api/) for generating one through Postman is really thorough, but the NodeJS one isn't that much.
Could you guys expand on the existing Node.js fetch example documentation? What should I do with the response in order to get the access token?
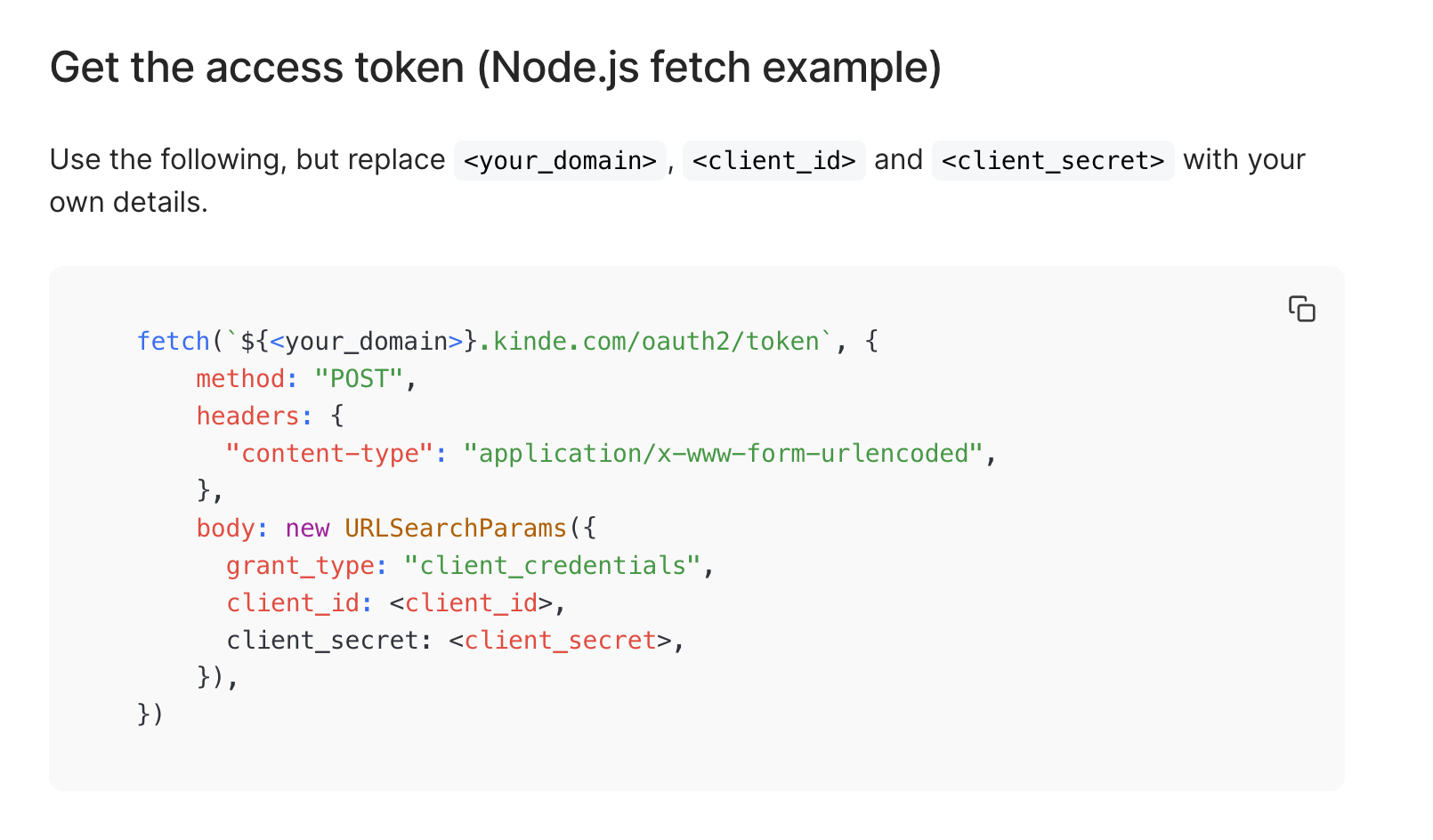
9 Replies
Solved this way, if anyone else struggles with this issue:
The access tokens generated via the client credentials are machine-to-machine tokens and won’t include the user subject. I’m not sure what you are after, but include the user part as well. For API authorization on user’s behalf, you’d need to register and enable the API in Kinde UI, then request the API’s audience as one of the auth parameters. This will include the API audience into the user token, which you can validate in you APIs.
For the long-running user sessions, you could use refresh tokens with the offline scope. https://kinde.com/docs/developer-tools/refresh-tokens/
Kinde Docs
Refresh tokens - Developer tools - Help center
Our developer tools provide everything you need to get started with Kinde.
I'm simply trying to provision a user using the Kinde Management API.
I would prefer to use the Kinde hosted UI to let a user create their account, but my specific use-case requires me to also create a user in my own database. And since Kinde Event Hooks is not accessible yet, I have to do it through the Management API.
Would be great if there was some sort of abstraction on top of the Access Tokens, because all this is so confusing and complicated...
Is there any ETA for Event Hooks being in Beta?
We have a pre beta setup that connects with Zapier. Would you be open to using that?
Event Hooks will be mid to late Q1 2024
Yeah, would love to try it out!
Hey @internetjohnny,
You can add the Kinde Zapier app (currently invite only) to your Zapier account using the following url: https://zapier.com/developer/public-invite/184402/d344c8c1953343fed6b6f36df02e733d/
Also have a read of the following related doc: Zapier App - Event Hooks (Beta)
Thank you!
Pleasure. As this feature is in beta, please don't hesitate to reach out with any feedback you have (the good, the bad, the ugly).