LED Strip WS2812B Issue with Arduino MEGA
Hi all,
I have an Arduino Mega, and I want to make a 2812B LED strip work. Right now, I'm just trying to figure out if my strip is functioning correctly. I simply want to display a solid color, for example, "green," on my LED strip.
I've placed the program below to display 300 LEDs in green on pin 2 of the Arduino. What happens is that with each program upload, around ten LEDs always display in white at the beginning of the strip, and the rest of the LEDs in green. Afterward, if I re-upload the same identical program, the LEDs turn red or some other color, and this happens every time I re-upload. Sometimes, random colors appear as well.
For now, I've only connected the + and - (5V) and the control wire to pin 2. I don't yet know what the other two wires are for.
Have you ever encountered this issue? I want to know if it's a problem with my LED strip or my program.
I have an external power supply: 220V ==> 5V / 20A / 200W and another backup supply of 220V ==> 5V / 10A / 50W.
6 Replies
It seems like you're experiencing some initialization issues with your WS2812B LED strip. Here are a few suggestions to troubleshoot and potentially resolve the problem:
Power Supply:
Ensure that your external power supply is providing a stable 5V output. Inconsistent power may result in unpredictable LED behavior.
Ground Connection:
Make sure the ground of the LED strip is connected to the Arduino's ground. This helps establish a common ground reference.
Capacitor on Power Lines:
Consider placing a capacitor (around 1000uF) across the power and ground lines of the LED strip. This can help stabilize the power supply.
Check Wiring:
Confirm that the data wire is properly connected to pin 2 on the Arduino and that there are no loose connections.
Try a Different Pin:
Experiment with using a different digital pin on the Arduino (e.g., pin 6) to see if the issue persists. It's possible there's interference on pin 2.
Update FastLED Library:
Ensure you have the latest version of the FastLED library installed. Sometimes, updating the library can resolve compatibility issues.
Add a Delay in Setup:
Add a short delay (e.g., 1000 milliseconds) after 'FastLED.show() ' in the 'setup()' function to allow the LED strip to stabilize before the program continues.
If the issue persists after trying these steps, it's possible there may be an issue with the LED strip itself. Try connecting a smaller number of LEDs (e.g., 10) and see if the problem persists. If it works reliably with a smaller number, there may be power limitations or data signal integrity issues with a longer strip.
Yes, I agree with @Saßì. What happens before the setup() is what you need to take care off. The random or white colour is a result of the digital noise at the DIN input during the microcontroller startup and firmware initialization. I recommend to start with a weak (10-100k) pulldown resistor at DIN. This resistor should keep low digital value during the MCU reset and init phase.
It's wise to address potential digital noise during microcontroller startup. The suggestion of using a weak pulldown resistor (10-100k) at the DIN input to maintain a low digital value during reset and initialization phases can help mitigate random or white color issues. It's a good practice for stable initialization.
Thank you @Saßì
Very true, Thank you @Petr Dvořák
led ws2812b 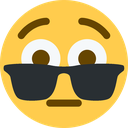
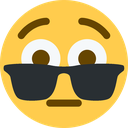