Implementing MediatR
How can i implement MediatR library to redirect the request relevant Service class (like GenerateCodeService, CreateProductService)
And i don't wanna use CQRS pattern
35 Replies
Makes no sense to use mediatr or similar if you're gonna use service classes anyways
MediatR works fine without an explicit CQRS split (commands vs queries), but it still relies heavily on the idea that a request has a handler
My folder structure for this thing is like this
ProductService.cs
ProductValidator.cs
AddProductCommand.cs
Your structure doesnt include a
ProductService.cs
It does but i just didn't sent it
if you just rename your "service" to
AddProductCommandHandler
you're doing things correctly.The thing is
wait i'll explain
do not mix and match services with command handlers. thats just silly.
AddProductCommandHandler.cs
ProductController.cs
It returns ok but it's empty
and it gives this error on console
But i'm %100 sure that my db connection string works
Because it works on other endponits
You are overengineering this massively. And using generic repositories over EF Core is redudant and bad practice.
Sounds odd that EF would throw that error if the connection worked, so I can only guess that its related to your service lifetime?
How can i simplify this like as i said my supervisor asked me to "implement MediatR library to redirect the request relevant Service class (like GenerateCodeService, CreateProductService)"
You did not say that, fyi.
This is the first time you mentioned a supervisor or being ordered to do something this way.
Still, this is an anti-pattern.
But other than being a bad pattern, I don't see any obvious problems in the code you have shared that would explain why EF can't connect specifically for this endpoint.
She also said
Injecting repositories into the controller directly is not suitable. We can build a new structure like this:
Controller > Service Class > Repository
I mean that works like a charm
But when i tried to use MediatR library to redirect the request relevant Service class
it's so messy
So i'm stuck...
That part is correct, you should not have business logic in a controller. What Im trying to get across is that you are doubling up on the intermediate layer.
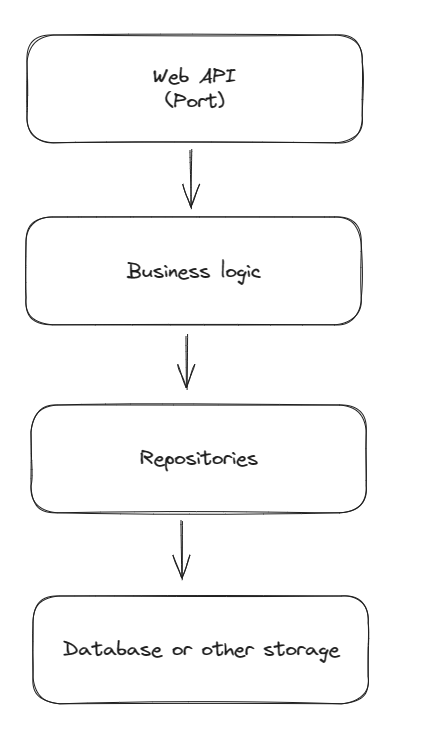
this is what it should look like
So do you suggest should i remove all the Features folder
use service instead
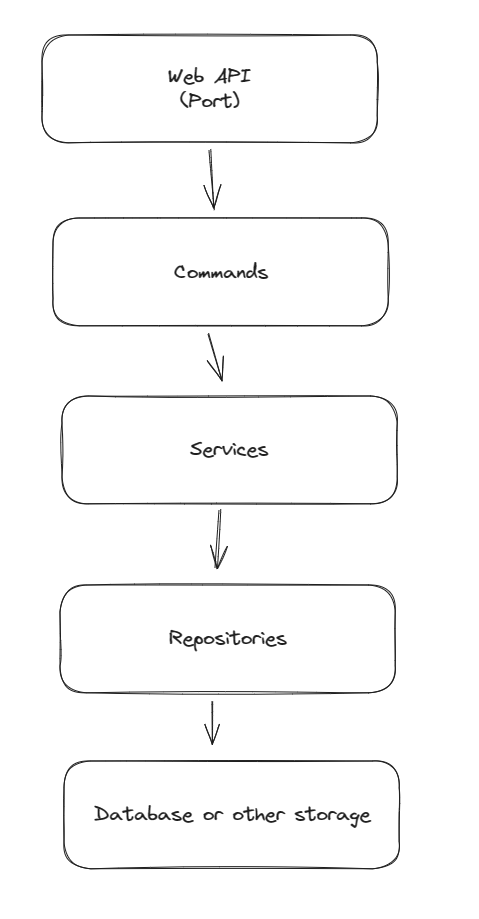
and this is what you are doing
And bytw
i'm not working on a real project
this is my internship assessment
I actually suggest skipping the
Services
layer, as thats the least useful
comand handlers are almost the exact same thing, but more granular
services are just multiple handlers grouped together for no purpose
so skip the service, use the handlers.
figure out why your database isnt workingit is kinda odd error
is this the correct way to inject mediart?
Program.cs
seems fine yeah
assuming all your handlers are actually in that assembly
Here is my full program.cs
new JsonDateTimeConverter()
? is this .net 6?
also, I personally prefer the services.AddMediatR(cfg => cfg.RegisterServicesFromAssemblyContaining<Startup>());
syntax
but they do the same thingnope 8.0
then you shouldn't need that line
STJ has full support for DateOnly and TimeOnly since .net 7
It didn't convert datetime utc to json
?
DateTimeOffset
?
it absolutely supports that, even since .net 5btw
my validators also not working
and if you are using anything other than
DateTimeOffset
, you are doing something wrong
DateTime
for example should never be usedHonestly
Wait
i just clicked execute again
and sql worked
first time it didnt
why are you using sqlexpress anyways
please use a more appropriate database. sqlserver, postgres, sqlite...
It creates the product in db but
Response body is {}
Response body is {}
well.. uh.. yes?
your command returns
Unit
lol
Unit
is MediatRs version of void
, aka nothing
so this is a comand that has no return valueoh yeah sorry
i'm kinda new