Best way to "reorder" elements differently at different breakpoints
I have the following design I made for my portfolio hero and now that I'm going about coding it, I realize I made a bit of a problem for myself. In my desktop view, my CTA button arrives before my social links and the hand image is in its own layout section. In the mobile view, the social links seem like they would arrive first in the HTML and the CTA button after.
I've decided I should switch over to trying to do my mobile side first today, as I had pretty much completed my hero focusing on the desktop view first. Here's the relevant HTML and SCSS, which works on desktop:
What's the best way to sort my HTML and SCSS to achieve this?
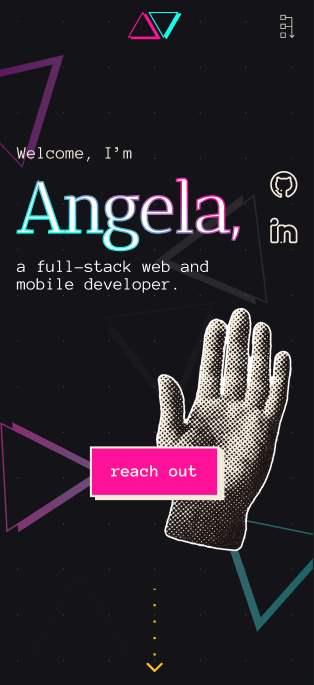
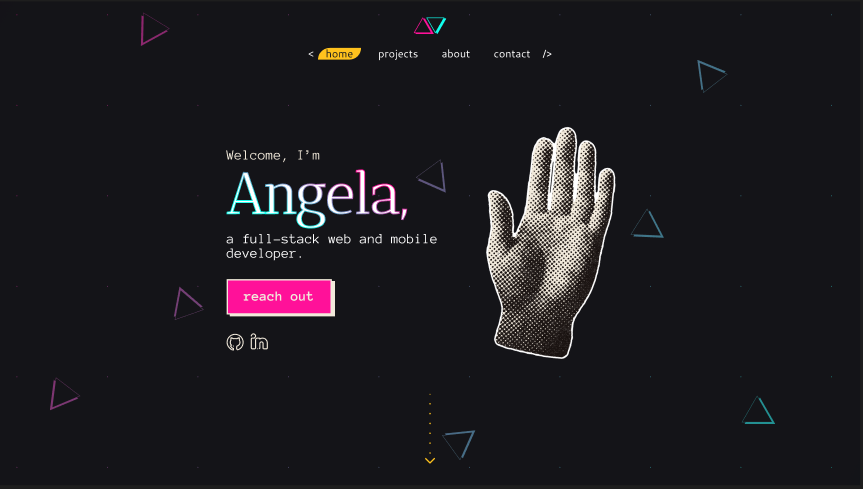
23 Replies
I've considered the flexbox order property, but at least the desktop version really feels more like a grid layout to me. I may be wrong; what do you think?
use grid-template-areas. for each breakpoint you can determine where every child is placed
https://developer.mozilla.org/en-US/docs/Web/CSS/grid-template-areas
MDN Web Docs
grid-template-areas - CSS: Cascading Style Sheets | MDN
The grid-template-areas CSS property specifies named grid areas, establishing the cells in the grid and assigning them names.
I'll try this out, thanks!
grid also accepts the order property if that helps
it does thank you!
(please let me know if this should be a separate question)
Here is what i have so far, but I'm stumped as to why my row height is not "respecting" the auto keyword for my first row. I've attatched a picture of the browser inspect with grid lines. I've tried min-content, max-content and fit-content as well. Here is the relevant scss:
Additionally, to center it in the page I have two divs wrapped around
#hero-content
:
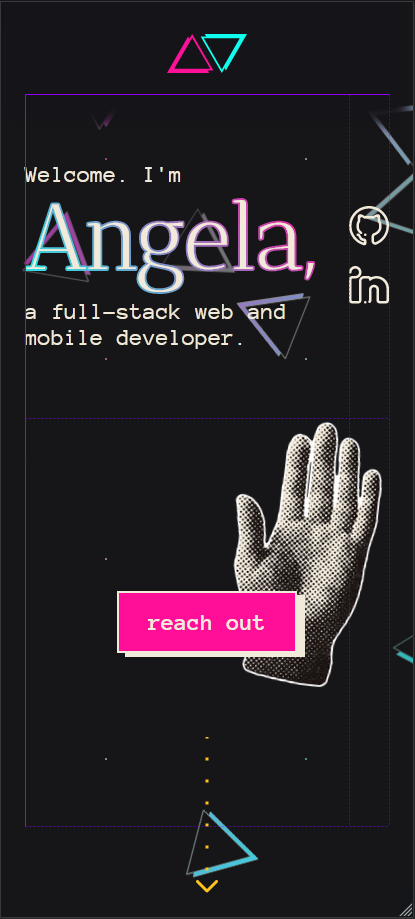
Never mind, just a simple syntax error I missed (commas in the grid template rows/columns)
I like your aesthetic
Would move the linked in and GitHub logos though
Thank you! Where would you move them to?
Not totally sure, maybe make them smaller, filled instead of stroked , in a row not a col and see how they look up in the nav or underneath “mobile developer”. Or maybe the lower right hand corner. They just look kind of awkward there with the bg element /decorations showing through. Kinda disrupts the balance between the chunk of “hi I’m Angela a full stack web and mobile dev” being on left and the hand and reach out button on the right.
so something a bit more like the desktop version I suppose?
I can see what you mean. The triangular background elements are just there for me to test, the current implementation is not how they're gonna end up I just cobbled something up in CSS to see visually for comparison. The final version would hopefully have animations, lowered opacity and saturation etc. to kind of distance it from the foreground elements.
I think you're right about having it below the tagline though.
Can you screenshot the desktop version ? They are just very large compared to the other elements where they are, at least on mobile.
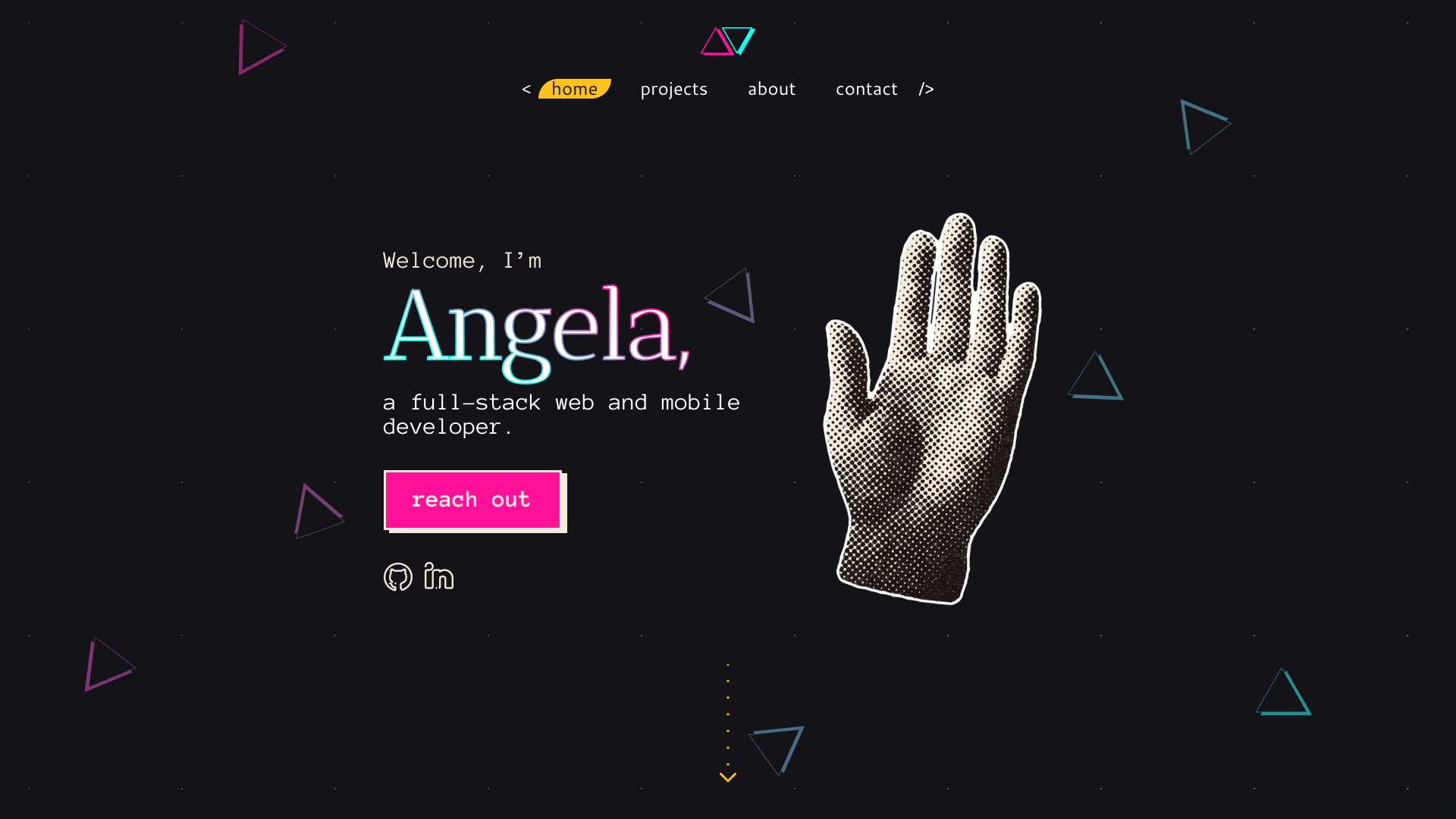
As for size, here's how it looks if its a bit smaller. I worry at this size it might be difficult to click?
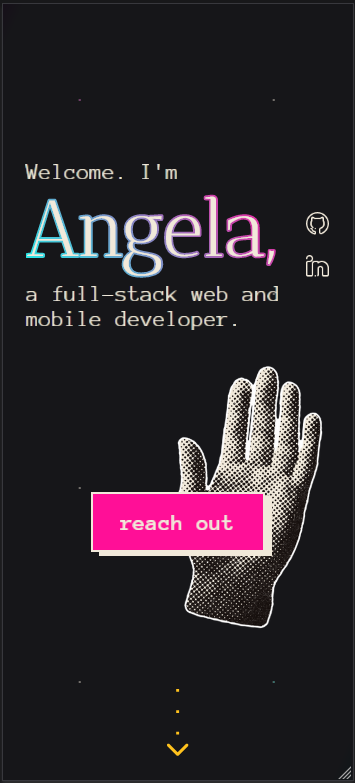
I give icons enough padding so they are visually the size I want but the tap area is still large enough.
Hmm it still looks awkward to me in that spot. What happens to the menu on mobile ?
It looks like so on mobile
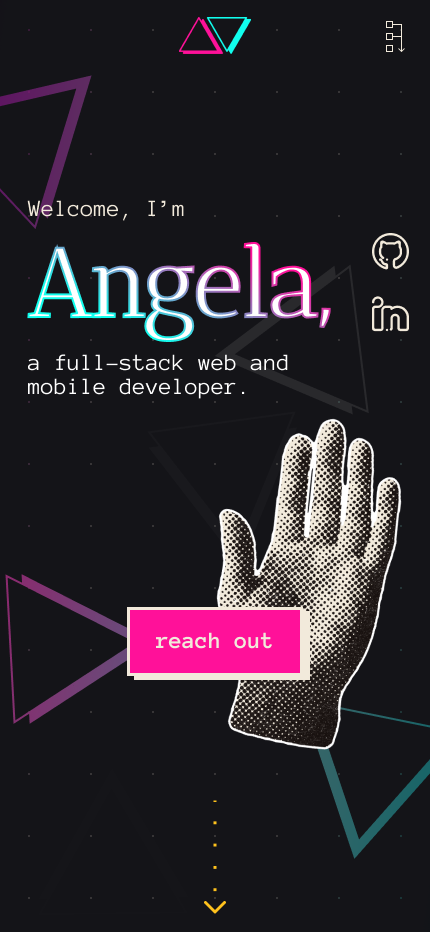
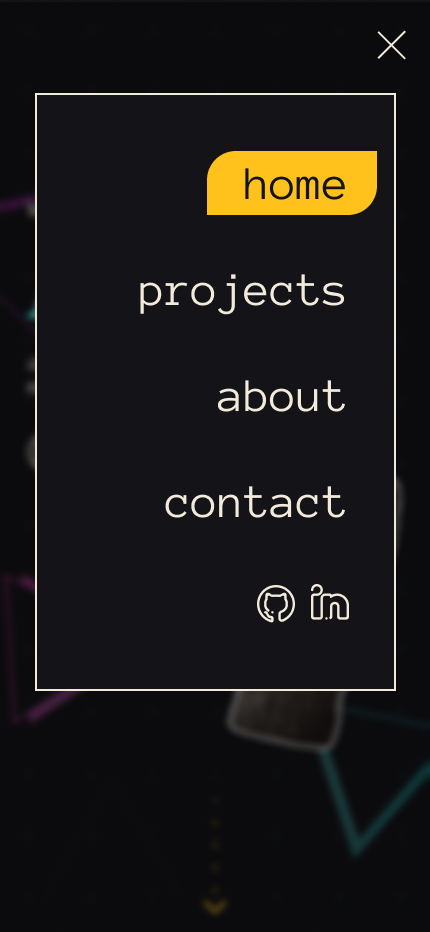
I just haven't actually implemented it yet
I was just gonna suggest to maybe throw them into that menu for mobile but you’re way ahead of me!
haha yeah i have my socials in a few places. I've watched some portfolio critiques and a common one is that people seem to like having the socials front and center, and easily accessible. I think I'll go ahead and just add them underneath the tagline and increase their padding like you said for a larger tap area.
What monospace font is that you’re using? It’s nice
Isn't it? It's called "Anonymous Pro" found here: https://fonts.google.com/specimen/Anonymous+Pro
Google Fonts
Anonymous Pro - Google Fonts
Anonymous Pro is a family of four fixed-width fonts designed especially with coding in mind. It is inspired by Anonymous 9, a freeware Macintosh bitmap font dev
I usually use source code pro for monospace typefaces but this one has a little flair , I like it a lot. Thank you for sharing it!
Of course haha thanks for your help! Yeah I really loved it as soon as I saw it, I wasn't originally planning on using a monospace at all 🙂