Worker to Worker fetch
Hi there,
I would like to ask for some help with my cloudflare worker. When i make a request to another cloudflare worker of mine that holds a variable with data in it from another cloudflare worker. The data returned is empty, however i make the data request from my browser or api tester, it returns the correct data. It is specifcally when I request the data from a cloudflare worker.
72 Replies
Apparently you can't do workers-to-workers fetch. You have to use service binding: https://developers.cloudflare.com/workers/configuration/bindings/about-service-bindings/
Service bindings - Configuration Ā· Cloudflare Workers docs
Service bindings are an API that facilitate Worker-to-Worker communication via explicit bindings defined in your configuration.
That's correct, the Workers either have to be on separate Cloudflare zones (e.g. example.com, example.net and example.workers.dev are all separate zones) or use Service Bindings
Service Bindings is the recommended solution
my workers are on seperate cloudflare zones, however it still cannot return the information correctly
What do you get back exactly?
a json containing order data
@Erisa | Support Engineer
its here in the code I provided
thats the cloudflare worker that hold the data
this is how i get data from it
@Erisa | Support Engineer
What URL is the Worker that has this code?
the one i sent now?
@kian
https://server.syntaxrecoveriesorders.workers.dev
https://manager.syntaxrecoveries.workers.dev/
@kian
anyone?
server.syntaxrecoveriesorders.workers.dev
and
server.syntaxrecoveriesorders.workers.dev
Are both on the same zone, so you can't make requests between them. You have to use service binding@McSneaky
bro
ready
their not the same
@Erisa | Support Engineer
can u both read
their not
.syntaxrecoveriesorders
.syntaxrecoveries
Aah, I was looking at your code example..
I assume everything under workers.dev need to use service binding tho
But that would be security issue, if they are all the same and can bind like that
Try to put one under your custom domain and keep other under workers.dev
Can u help me with how service bindings work?
@McSneaky
how do i communicate to server
Worker 1 called
"MY_SERVICE_TO_CALL"
:
Worker 2:
And inside your wrangler.toml
of Worker 2 define BINDING
and SERVICE
in services
That service has to be other worker name
https://discord.com/channels/595317990191398933/1193924170749317161/1193924170749317161
Learned it yesterday š
@McSneaky
what is MY_SERVICE_TO_CALL
services = [
{ binding = "server", service = "server.syntaxrecoveries.workers.dev" },
]?
It's your worker name
server?
can i get an example?
Worker
no bro
example of the name
wym by name
please
What's the name of your other worker to call
You can check it's name from other worker
wrangler.toml
or from CloudFlare dashboardserver
its called
server
Aah, thought you have literal server, like VPS š
Then yeah, "server" in your case š
lol
š«¶
Then
env.server.fetch('https://server/orders/10')
Something like this should work for youhow do you get env if its not a request?
like if i want to postData just as a function
not in a route
i need env still
Most likely need to pass it to that function
i dont think i can
its hard to explain
no
im not using router.get
like where do i get env from
without getting it from router
oh
wait
lmao
nvm
sorry
im dumb
also
error code: 1042
https://server.syntaxrecoveriesorders.workers.dev/orderhttps://developers.cloudflare.com/workers/runtime-apis/service-bindings#interface
It's also 2nd arg in there
Service bindings - Runtime APIs Ā· Cloudflare Workers docs
Facilitate Worker-to-Worker communication.
Replace all that
https://server.syntaxrecoveriesorders.workers.dev/order
With just https://server/order
no something is wrong
can i show u in a screenshare?
i did
in my code
Hmm.. Then maybe it works differently in
workers.dev
š¤
I was using on actual domainim getting error 1101
No idea what that is
internal service error
No idea what that is either š
on my manager worker
š„ŗ
looks good to me
idk why its throwing an error
im using getData btw
just for now
Yeah, looks good
You can try to wrap some try-catches in there or .catch()'es where to log out errors
See maybe there's something more useful than just that error code
env
is undefined?!yerr lmao
Eeee š
How so š¤
idk
You are using that
itty-router
?positive
No idea..
That's what I have
Worker 1 called "edge-encoder":
Worker 2 called "edge-collector-dev":
Works perfectly š¤
No idea why env is undefined for you
And what are those odd error codes you got
^
Log out
env
in router to see if it exists therewhat?
Aah, are you running on local with
dev
?
Service binding might work only in prod
Have to wrangler deploy
and then test in prod š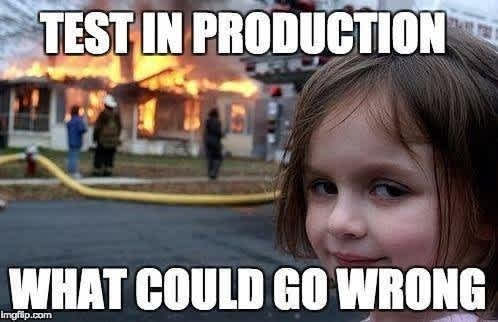
idk what ur saying
im new to cloudflare workers
How are you running your test env?
wrangler dev
?
npm run dev?
Yeah, I'm not sure if that that works
You might need to deploy both workers with
wrangler deploy
and then test on production (real URL, not local)i have been doing that
And then you get same error?
yes
Welp š
Then I'm all outta ideas
TypeError: Cannot read properties of undefined (reading 'order_server')
TypeError: Cannot read properties of undefined (reading 'order_server')
TypeError: Cannot read properties of undefined (reading 'order_server')
TypeError: Cannot read properties of undefined (reading 'order_server')
so annoyinh
@McSneaky
I have no idea..
env
is undefined for you for some reason, but I have no idea why
For me it worked outta box
ĀÆ\_(ć)_/ĀÆ@McSneaky
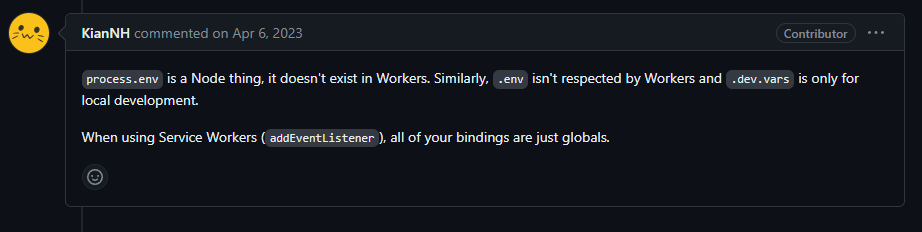
Aah, but you don't have Node env. It's ctx env kinda thingy
@McSneaky
do both workers have to be binded?
Nope, only one
can i send u my full code?
can someone help
pls
anyone?
https://developers.cloudflare.com/workers/configuration/bindings/about-service-bindings/
This guide should help you understand service bindings and how to use them.
Service bindings - Configuration Ā· Cloudflare Workers docs
Service bindings are an API that facilitate Worker-to-Worker communication via explicit bindings defined in your configuration.
@Unsmart | Tech debt
The issue is that it doesnt work.
It literally doesnt work.
ive done all that
Did you follow the tutorial exactly in order to create one and understand how it works instead of trying to do it in your own project first?
It does indeed work many people use it. If you cant figure it out I highly suggest trying to pay a developer to either do it for you or support you through to understanding how it works š
Hey bro @Unsmart | Tech debt, yeah no i did read it, all is set up perfectly fine. All my code is in the above messages.
Like it really has no reason not to work.
Because the code of the binded worker is fine, i can sned a request to it on my browser perfectly fine. It just doesnt work with the worker that is binding with it.
The issue is my
env
is undefined...
We cant figure out why tho.