changing stuff around when a button is clicked
how would i make it so when a button is clicked on an embed, it does the following things;
change the embed into something else (title, desc, etc.)
make the buttons disable (gray out)
make the bot reply with an epheral message saying whatever
32 Replies
- What's your exact discord.js
npm list discord.js
and node node -v
version?
- Not a discord.js issue? Check out #other-js-ts.
- Consider reading #how-to-get-help to improve your question!
- Explain what exactly your issue is.
- Post the full error stack trace, not just the top part!
- Show your code!
- Issue solved? Press the button!You need to create a message component collector or simply use interactionCreate event
Make a new action row using static
ActionRowBuilder#from
and change button disabled property (Or make a normal action row with using button (ButtonBuilder#from
) and use ButtonBuilder#setDisabled
method)
Then edit the original message with the other embed (you can also use static EmbedBuilder#from
method) <Buttoninteraction>.update(...)
he would need to use
<Interaction>.message.edit
instead of update cus he wants to send a reply after editingthey could also just use
<ButtonInteraction>.followUp()
after using <ButtonInteraction>.update()
this would be the means of achieving this through the interaction webhookDoes followUp support ephemeral?
yes
oh, good to know
im sorry im a bit lost here
i'm new to DJS 😅
this is what i have thus far
Wdym?
interactions can only be responded to once, so you can't use both
<ButtonInteraction>.update()
and <ButtonInteraction>.reply()
, as these are both initial interaction responses
I imagine lavaboy was suggesting using <ButtonInteraction>.reply()
to send the subsequent ephemeral message and <ButtonInteraction>.message.edit()
to update the button's message
personally I would still suggest using <ButtonInteraction>.update()
to update the button's message and <ButtonInteraction>.followUp()
to send the subsequent ephemeral message since both of these methods go through the interaction rather than needing to edit the message as a bot user
utilizing the interaction webhook bypasses the need to think about any sort of restrictions such as permissions, whereas <ButtonInteraction>.message.edit()
at least requires your bot to be able to view the channel and its messagesWhere would I use this though. And how would I make it so it checks for which button was clicked (because one button needs to edit the embed to one thing, while the other button needs to edit it to something else
Also what's <ButtonInteraction>
Every button has a custom id
I see.
But where would I put this into my code, I don't know where to add it into
Read guide
that I sent
and this
right so the problem im having now is when i'm trying to use update() it's telling me "Unresolved function or method update()"
here's the piece of code where it's in
That does not how buttons and interaction work
Please read guide
There's everything you need to know about component interactions and buttons
Right, but I have multiple permanent buttons the The Client#interactionCreate event part of the guide only explains how to do it for one any button, which is not what I'm looking for
I'm looking for how to make a specific button on an embed do what I tell it to
Every button have custom id
You need to check in every button interaction it's custom id (
ButtonInteraction#customId
)
If custom id matches
Then execute code for that buttonYeah but my ID is in another file
this is interactionCreate.js
You will receive custom id in interaction object
what do you mean
You have this property on interaction
Use that
oh wait
so can i make an if statement in the else if loop
saying if the id is one of the button's, it executes code?
so like
alright so i've gotten to the point where when i click a button, the content updates
what i need now is when the button is clicked, the embed updates, but all the embeds and data that's in the embed is stored in seperate file from the button handler
is it possible that i can onlyupdate a piece of the embed wihout rebuilding the entire embed from the ground up and if so how
As I said higher you can use static
EmbedBuilder#from
methodyeah but how do i utilize JSON here? my embed builders look like this, i'm not sure how this static thingy works
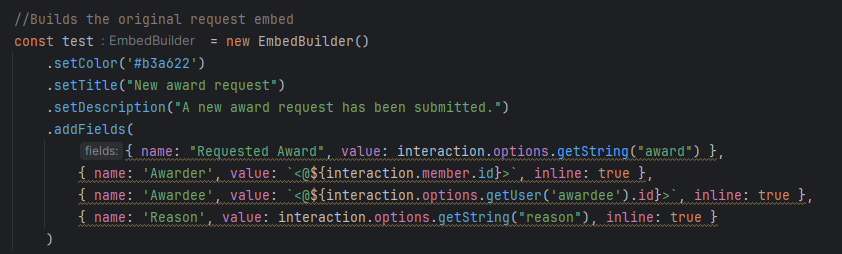
EmbedBuilder.from()
would be used to create an EmbedBuilder
from existing data when you don't already have an EmbedBuilder
in the given context, so you probably wouldn't use it here where you presumably send the embed and the buttons in the first place
the aforementioned <ButtonInteraction>.message
is the message the button is on, so you're free to grab your Embed
from <ButtonInteraction>.message.embeds
wherever you're handling button interactions