TypeError: Cannot read properties of undefined (reading 'user')
hi I'm trying to get some info on the user tag, but doesnt work on myself (just typing the command and passing myself as a user)
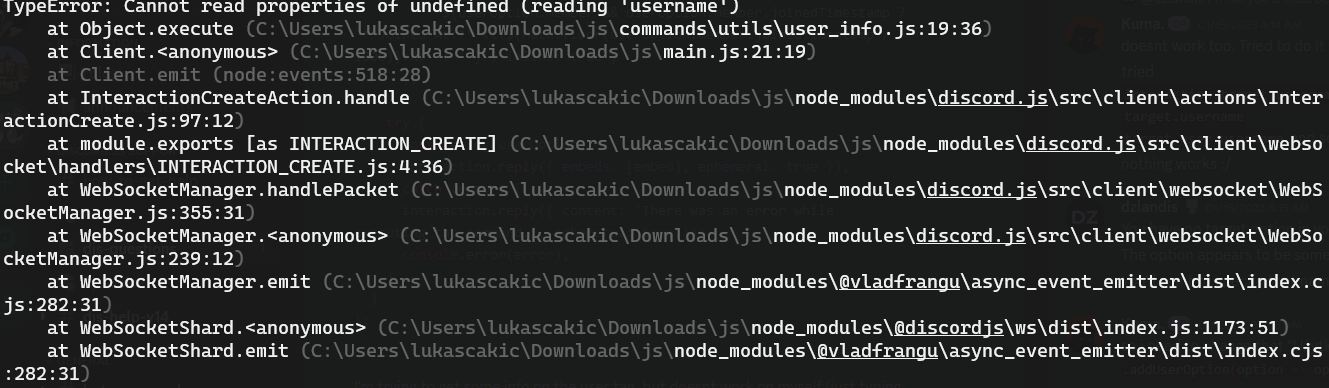
3 Replies
- What's your exact discord.js
npm list discord.js
and node node -v
version?
- Not a discord.js issue? Check out #other-js-ts.
- Consider reading #how-to-get-help to improve your question!
- Explain what exactly your issue is.
- Post the full error stack trace, not just the top part!
- Show your code!
- Issue solved? Press the button!
- ✅
Marked as resolved by staffuse getUser
i'll test that
it worked thanks