How do I show the data from the description of another table and not a number?
how to work relationships

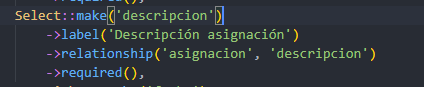
24 Replies
Does your model have a relationship for this?
Looks like your making a Select form item for a string field. Try TextInput instead 👍
my table
Ah I just re-read the title of the post... if you have a
Descripcion
model, then your table will need a descripcion_id
.
Then your Asignacion
model will need to have the relationship setup correctly like so
my form
I understand but what I want is to have the data from the description field of my stock table, that are equal to my description field of my asignaciones table. but it converts them into a number in the database
how much do I put the select button
Is it adding the id of the descripcion in that column, but you want to add the descripcion value or something? I don't know what the Descripcion model looks like, but you can use dot syntax too so maybe something like
descripcion.name
instead?
Sorry, I'm not sure I fully understand what you're trying to achieve.What I'm trying to do is simply have the description field of my stock table = the description field of my assignments table, but when I create a select in my resource assignments, it sends it to the database as a number and I want the text

So what does your
descripciones
(or whatever it's called) table look like?like this
this is what I want T_T
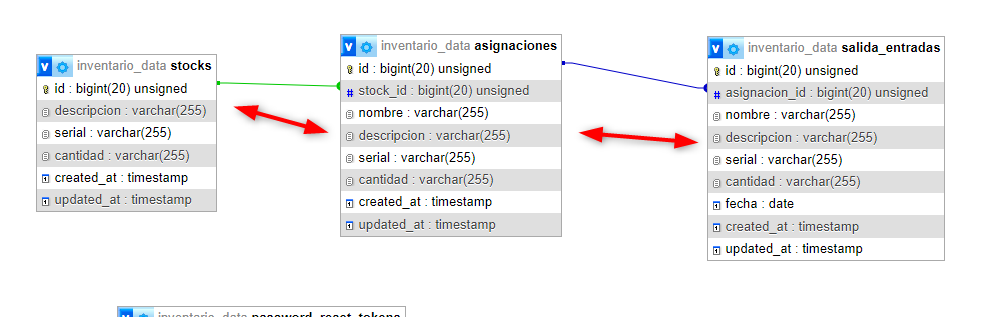
That the same values of the field be sent in the database, because there are no problems in the panel
because it is displayed correctly and searched correctly in the form
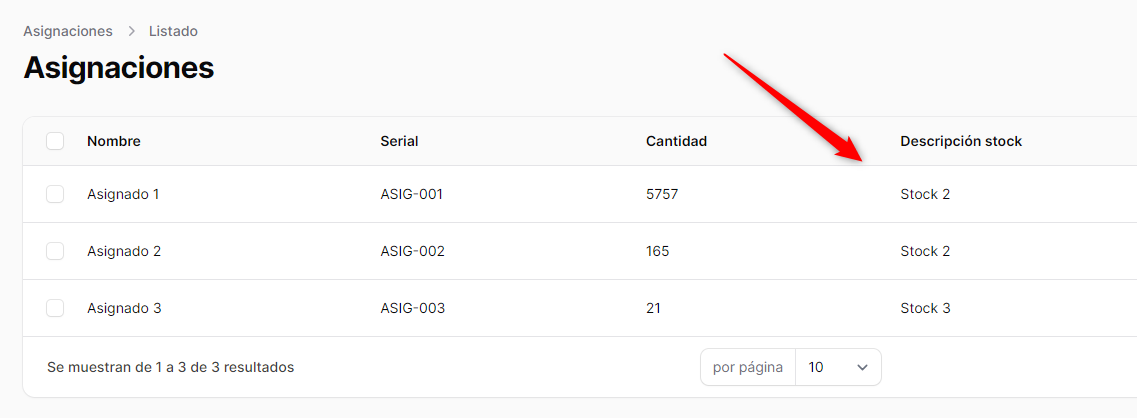
In that table, are you linking it to
stock.descripcion
?yeah in the column
So can you do the same in your form? 🤔
Is this possible? (Stock) Field (Descripcion) = (Asignaciones) Field (Descripcion)
and not a number T_T
only real record T_T TEXT!
I'm fairly new to Filament but maybe try something like this in your
EditAssignacion.php
file:

ITS WORK! :DDDDD
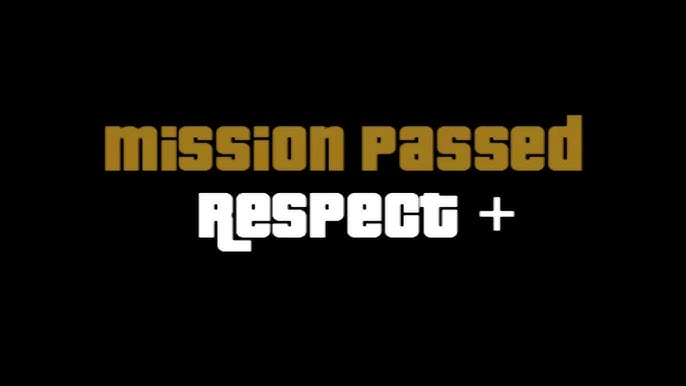
How do you know where you get those types of solutions? I'm new too
Well Filament is very well documented but sometimes you need to have some kind of clue as to what you're looking for. I had recently used the afterSave hook, so I just kind of guessed that there would also be a beforeSave or something and eventually found this:
https://filamentphp.com/docs/3.x/panels/resources/editing-records#customizing-data-before-saving
On a side note; Doing it this way means that if the stock descripcion gets updated, the assignacion descipcion won't match it anymore and they're going to be out of sync. If you want them to always match then you're better off not storing the descripcion in that table at all and instead always fetching the
$assignacion->stock->descripcion
instead.
Of course, I don't know what your specific use-case is, so there's probably a reason you're copying the data over in this way.
Either way, I'm happy you've got it working 😀Solution
thanks