ButtonBuilder > TypeError: Cannot read properties of undefined (reading 'customId')
Part of
Index.js
20 Replies
- What's your exact discord.js
npm list discord.js
and node node -v
version?
- Not a discord.js issue? Check out #other-js-ts.
- Consider reading #how-to-get-help to improve your question!
- Explain what exactly your issue is.
- Post the full error stack trace, not just the top part!
- Show your code!
- Issue solved? Press the button!
- ✅
Marked as resolved by OPstart-contract.js
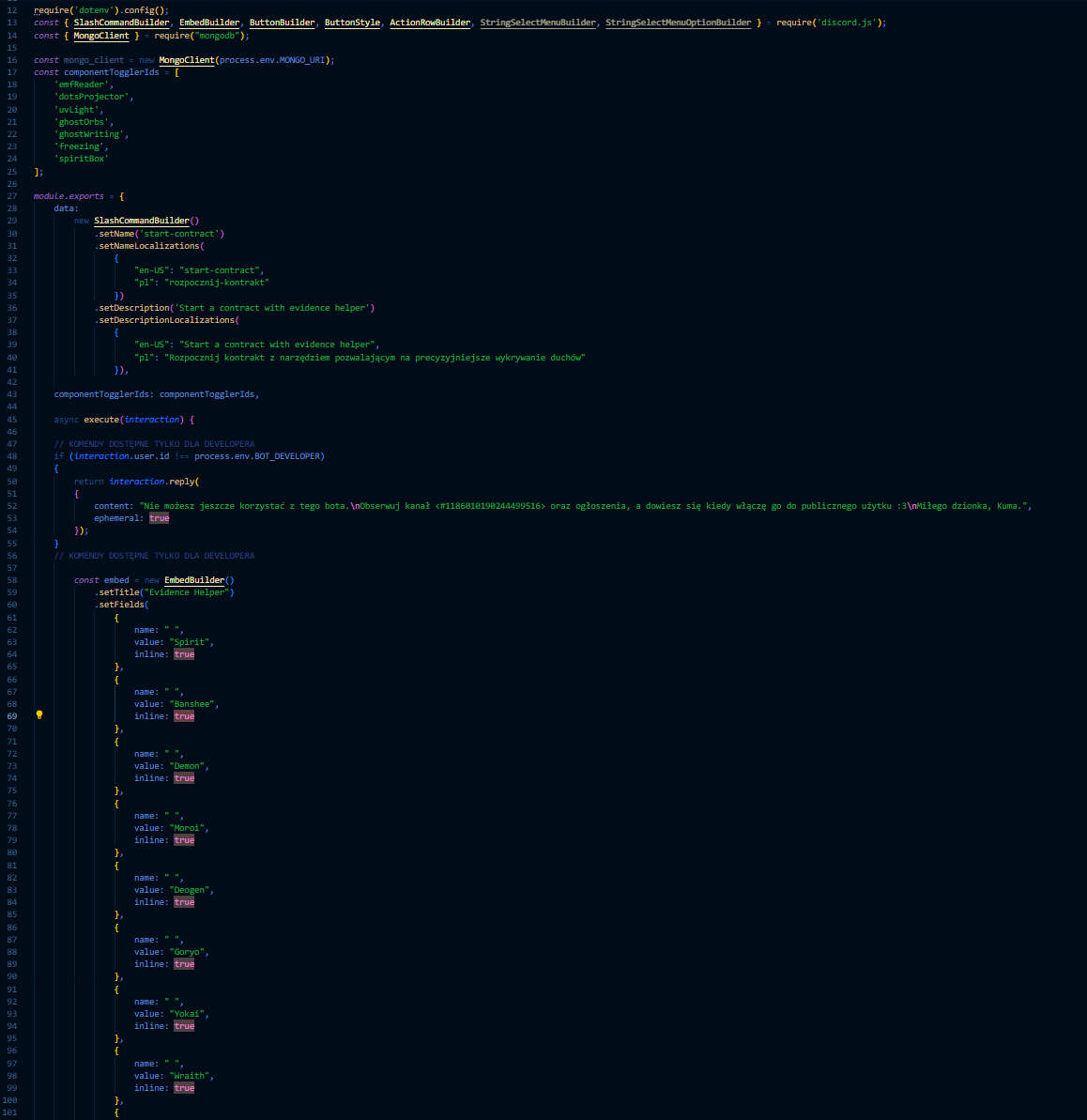
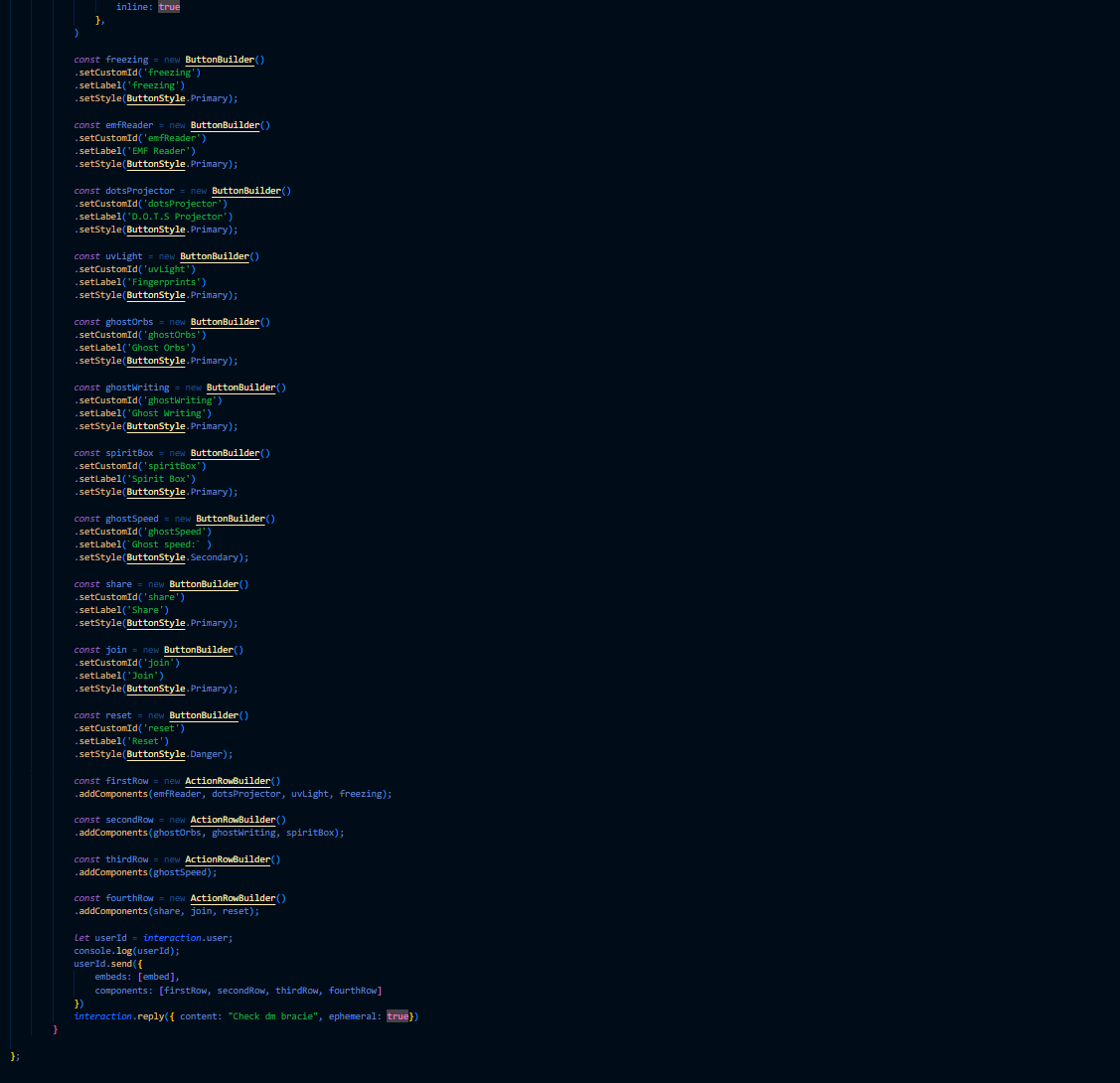
Freezing button only returns error. Other buttons work properly. Changing label and id still gives same kind of error
Show the error and stack trace.
What is index.js line 144?
const currentId = currentButton.customId;
CurrentButton is undefined at that point. You should log some values and find where your logic isn’t finding what you expect.
check comment above line 144
it properly returns id of freezing everywhere above
ok, i'll show u smth i've found rn
console log near 144
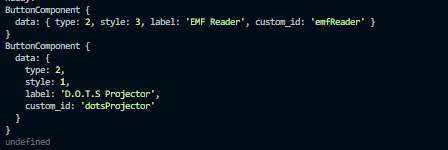
other buttons vs last freezing
Right it’s undefined.
buttonindex for first 3 buttons are 0, 1 and 2
but for freezing its 5
And how many buttons does that row have?
yeah, it should return 3, i've changed it manually to 3 and it works, but buttonindex returns 5
4
buttons
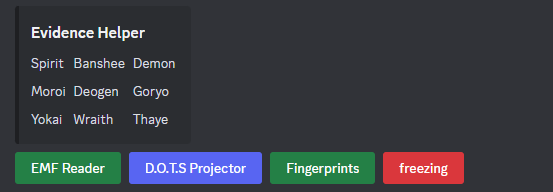
so indexes should be
0; 1; 2; 3
not 0; 1; 2; 5
hm
i think i know
let me checkCheck componentTogglerIds.
yeah, it depends on order that i've set wrong xD
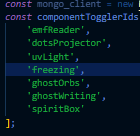
So it's solved. Wrong order inside of object. Thanks @SpecialSauce 
