req.body returns undefined
haya!!. So today i was thinking of trying out backend. and i installed express and nodemon and started working on it. everything seemed to be working fine until I made a form in my html and tried to receive and log data in my server.js. But it seems like req.body always returns undefined . I know the server and client side is working fine cause when I submit, it gives my the error I coded in the
server.post()
. That means i am indeed sending post req to my server . Here is the github code the code: https://github.com/glutonium69/Codespace_1/GitHub
GitHub - glutonium69/Codespace_1: Testing out github codespace
Testing out github codespace. Contribute to glutonium69/Codespace_1 development by creating an account on GitHub.
25 Replies
my code isn't that big so i'll leave the code here as well
html body
script.js
server.js
u can see thr error it is returning is the error from my server.js

In the network tab, how does the Request looks like?
Can you console log req.body? Does it have anything at all?
it console.logs empty object
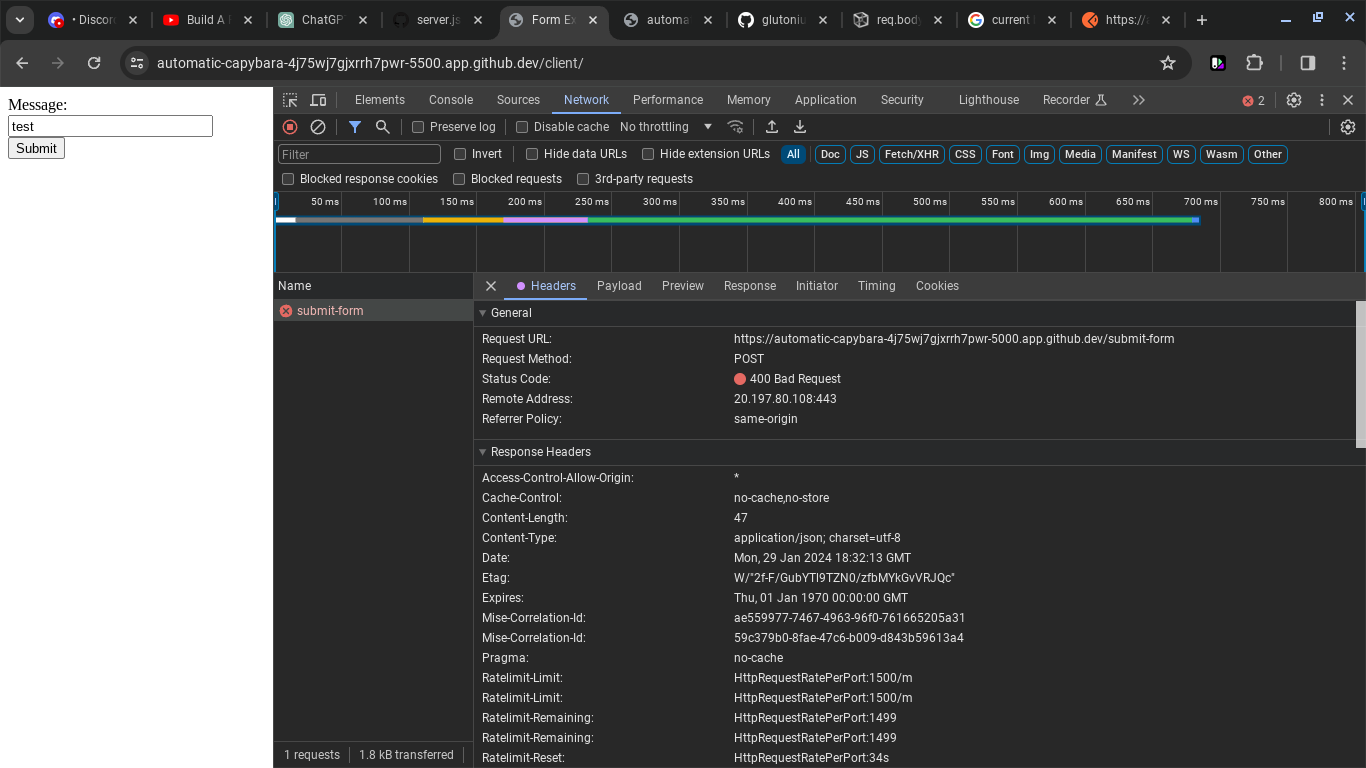
Mm I'm not sure if perhaps a specific parser for express is needed if you use formdata 😕
k so I found out that now u have to install body-parser separately
its no longer with express
so i updated my server.js
but still the same issue
I was just reading this, maybe that's the issue... https://masteringjs.io/tutorials/express/post-form
lemme check
But I find it strange that it doesn't handle FormData requests so I'm not sure if this is really necessary, even if it works.
it should tecnically do it
cause i mean its just a post req after all
That would be only for the routing not the parsing of data. It must send it in some format that is not plain text nor JSON.
What happens if you do JSON.stringify(formData) on the client?
Try with:
k so i did this
this time it didnt return empty object
but some sort of crypted msg
xD
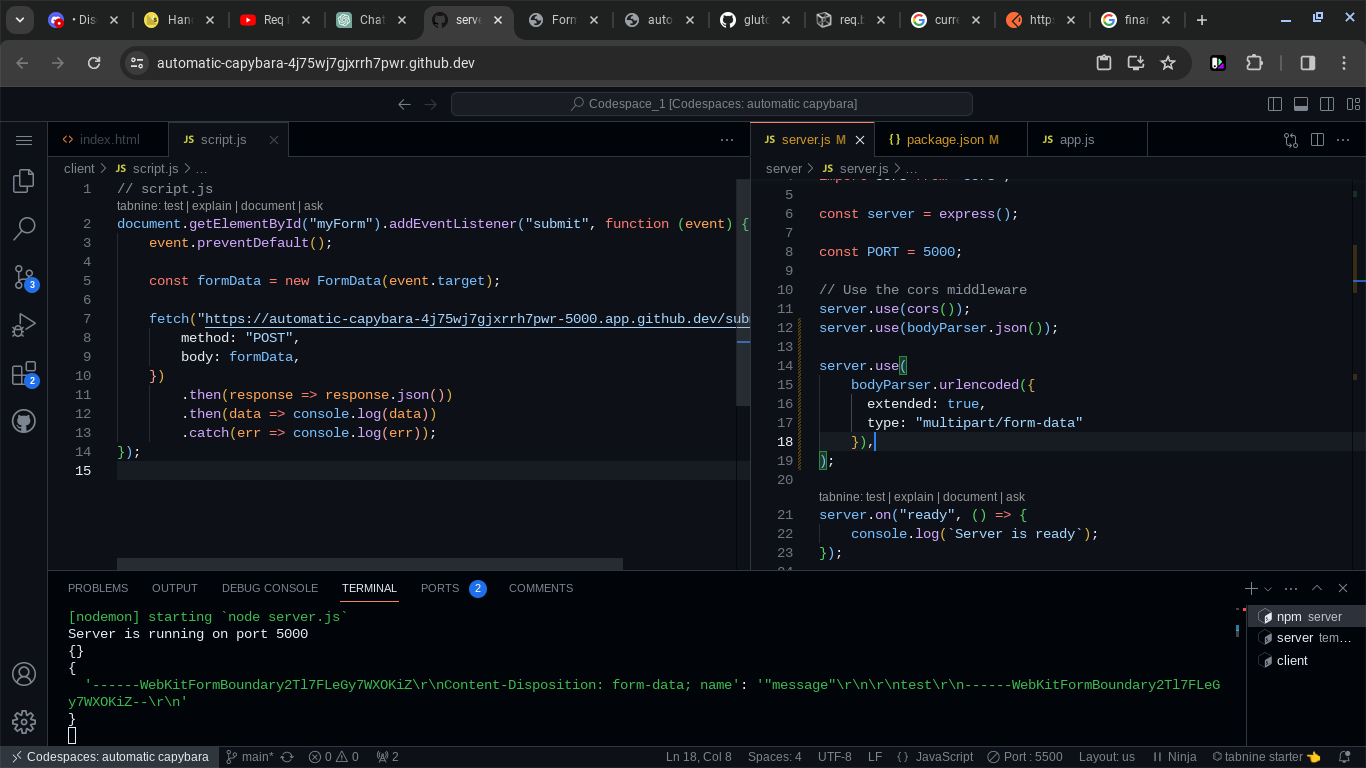
but the req wassnt a success
as it returned error to the client side
ok no wait
dis encryted msg
it does have the form data
Mmm well, try using that library from the link above. Maybe it really jsut doesn't handle it.
ok
If you find out let me know 🤷♂️
okkii
k so it turns out
formidable.IncomingForm()
is not a constructor
not sure if they changed it
or something
i also searched up the pkg
it seems like, it is used mostly for filesI've definitely seen it used for regular forms, but most commonly the form object is constructed manually using the values of each input. I would personally use that, and use JSON stringify
gotcha
tnx for the help btw
I managed to get the data from the form using multer
https://github.com/expressjs/multer
install it with npm and add the following in your code:
GitHub
GitHub - expressjs/multer: Node.js middleware for handling `multipa...
Node.js middleware for handling
multipart/form-data
. - GitHub - expressjs/multer: Node.js middleware for handling multipart/form-data
.hey.. sorry for late response
i did try this one
and surprisingly it worked
thanks you so much
@Joaohey. The middleware Heitor provided seems to work just fine.. U had told me to let u know so i thought i'd do dat
Yeah, thanks for that. It looks like it's treating it as a file somehow, but at least that's one one do get it to work
ya