Better way to return a specific item from json
I just want to return the strings from the definitions.definitions objects... but the way I'm doing it feels kinda not optimized as it is about to become an O(n³). Does anyone know a better way?
Here is the link of the api to understand its structure:
https://api.dictionaryapi.dev/api/v2/entries/en/oi
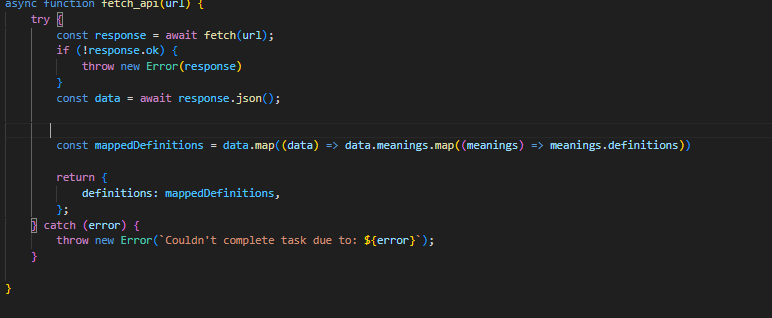
6 Replies
i think that is the way to do it.
you could use a regex, but don't know if this introduces other incertainties
(i think it will struggle if the definition includes escaped
"
's
Im not great at it, but came up with this. (it does work with your example data)
It matches all texts between "definition":"
and "
Have you benchmarked how long it takes? O(n^3) doesn’t really matter if n is small or if the tasks are fast
Aim for clear readable, editable, code first; if you notice that there is a delay, find out what is causing it. Only then try and optimise
i remember i used this api for my wordle game to check if the users input is valid or not. I also extracted definition.
here's how i used it
here it will only return the first result
Yeah
I need all of them
aah i see
hmm