runtime of loops
Why that variation occurs between for, for...of and forEach?
I'm posting here because I was running it in a "nodejs env"
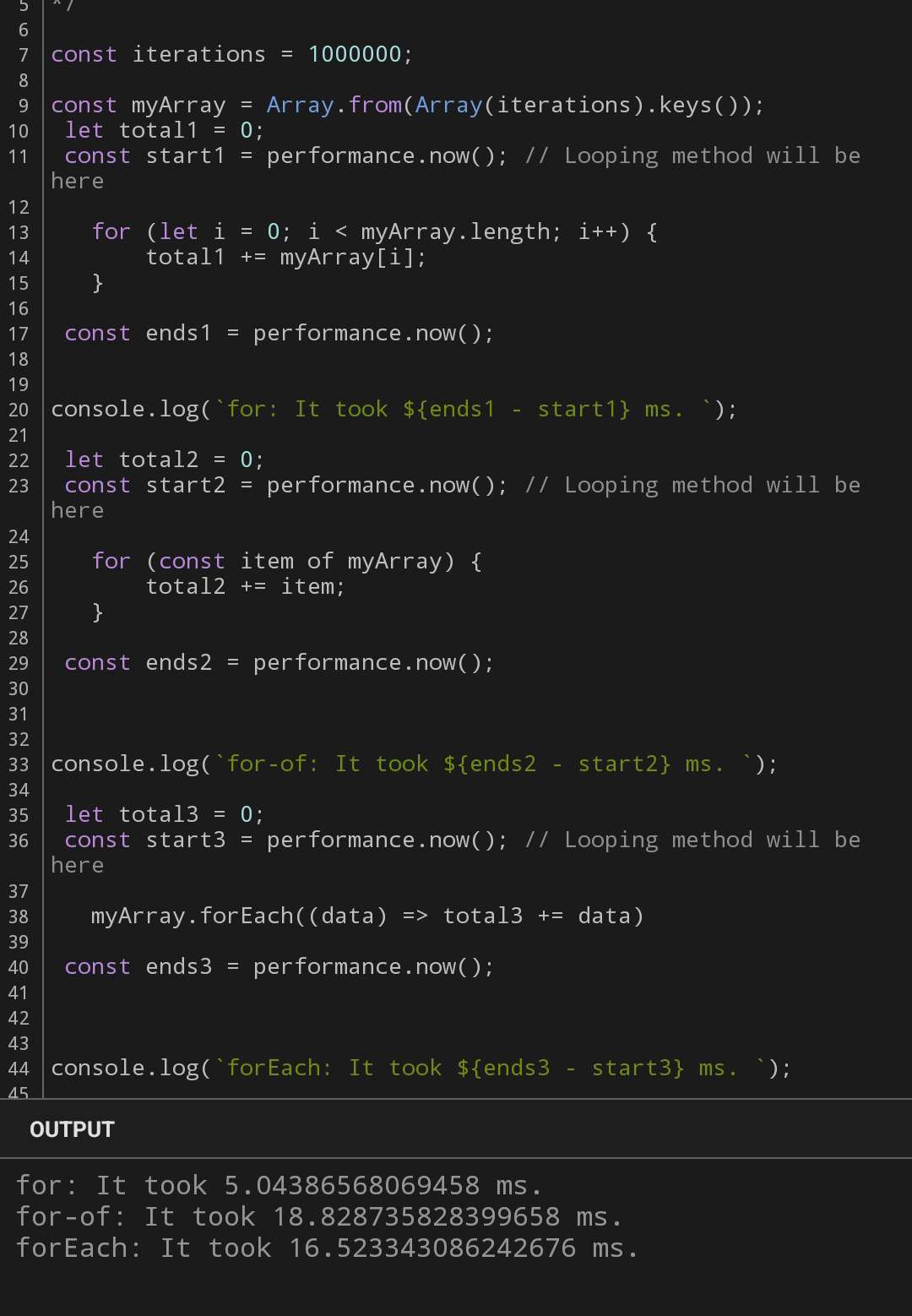
5 Replies
Code:
I don't know too much about the internal workings of JS, but the foreach calls a callback function rather than just looping over a block of code. That's got more overhead. I'm assuming for-of has a similar issue
What is even more weird is that the results also changes depending on the increase or decrease of amount of iterations
For 10.000 iterations, forEach was the winner
I would mostly like to point out that, while it's fine to wonder about this stuff and want to know the reasons, it's not worth thinking about when you're writing production code
no one is going to notice a 15ms delay in processing, and you're just measuring the overhead of the loop anyway, the real slowdown would come from the potentially complicated body of the loop (however you run it) rather than the mechanism of the loop itself
what matters much, much more than this level of performance futzing, is readable code. Write what you think is readable, and worry about the performance if you see a hit. You can always profile your code and see where the slowdown happens if it's ever noticeable
It makes sense