Receiving "rc= -2" error with ESP32 MQTT TLS certificates
I'm trying to send encrypted messages using tls from esp32 to my localhost mqtt server ( using mosquitto ) .I'm getting an error rc= -2 when using certificates for encryption . Code was working fine without encryption . Please help .
14 Replies
Ive configured my mosquitto config file also , adding the required listeners and path to certificates
(generated the certificates using openssl)
@Aditya thakekar and @LMtx may have some insight here
Connection timeout?
Share the print logs
Rootca certificate issue seems like
serial monitor
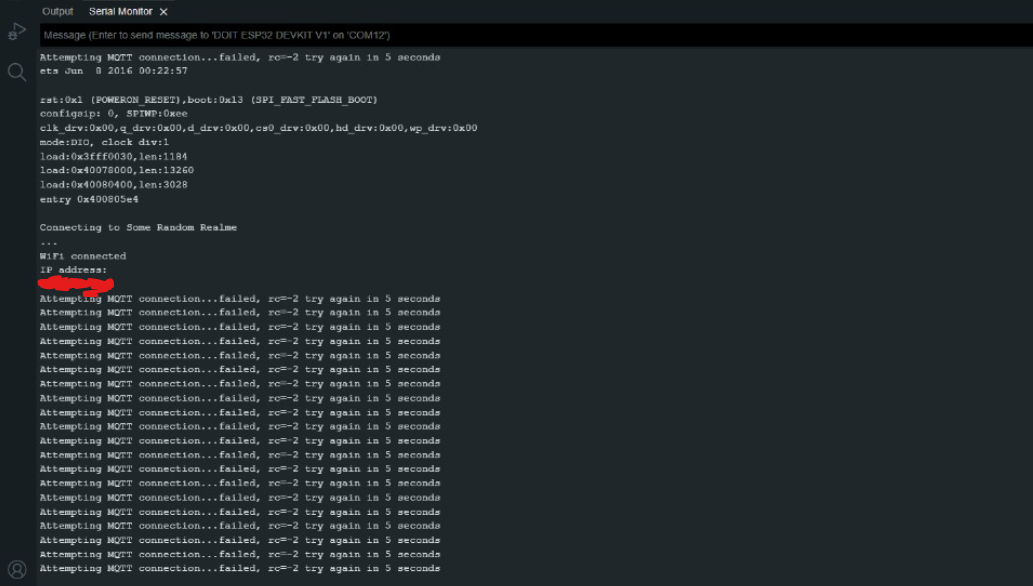
i generated certificates referring this
You are doing https on mqtt
Where is the pub sub network
I didnt quite get what you meant
isnt this how we can publish?
also i used wificlientsecure to use tls with mqtt , not https
Check the backend first if you can
Void setup() - steps check.
setup_wifi();
client.setServer(mqtt_server, 1883);
client.setCallback(callback);
Check callback...
Server ip address too
server is a broker service called mosquitto running on my localhost ... so its my localhost ipv4 address
Mqqt backend
Mosquito conf?
Tcp is not configured properly
Open the conf files and check
Firewall is stopping
ive changed mosquitto.conf like this
i tried websockeets on a different ports - 8091 and 888
Tcp connection for windows firewall
ive disabled all firewall , and added an inbound rule in windows defender firewall with advanced security to allow only specific remote ports and entered the ports i tried like 1883, 8883, 8884, 8091 etc (i tried using all these ports with/without encryption and with/without websockets)