Generate new DOM
I was showing my stuff for other people and they said the way I'm generating new DOM is a bad practice. Is that so? what would be a better way to achieve athe same thing then?
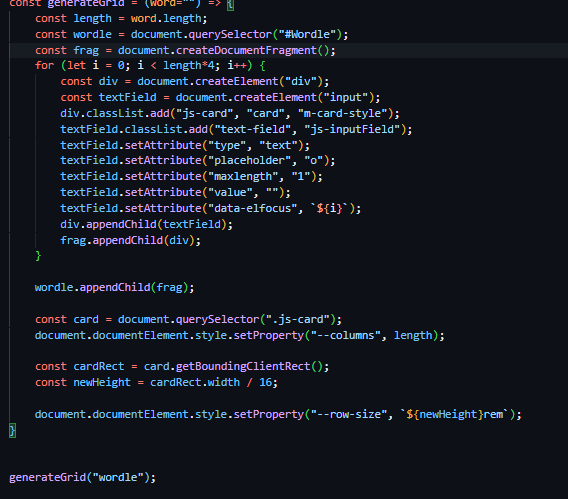
10 Replies
why are u using document fragment
and not directly appending to the main dom
I heard it is more performant using a document fragment
it is not nescessary here
i think if there are cases where u have to make dom element but add it to the main dom after a certain time or in certain state then u can use it. that way your main dom is not filled with unnescessary elements
at least thats what i think how it works
anyways, try changing your css to this
this makes it responsive
What about rows
It needs to have specifically 4 rows
it will automatically generate new row
it gets too big aswell
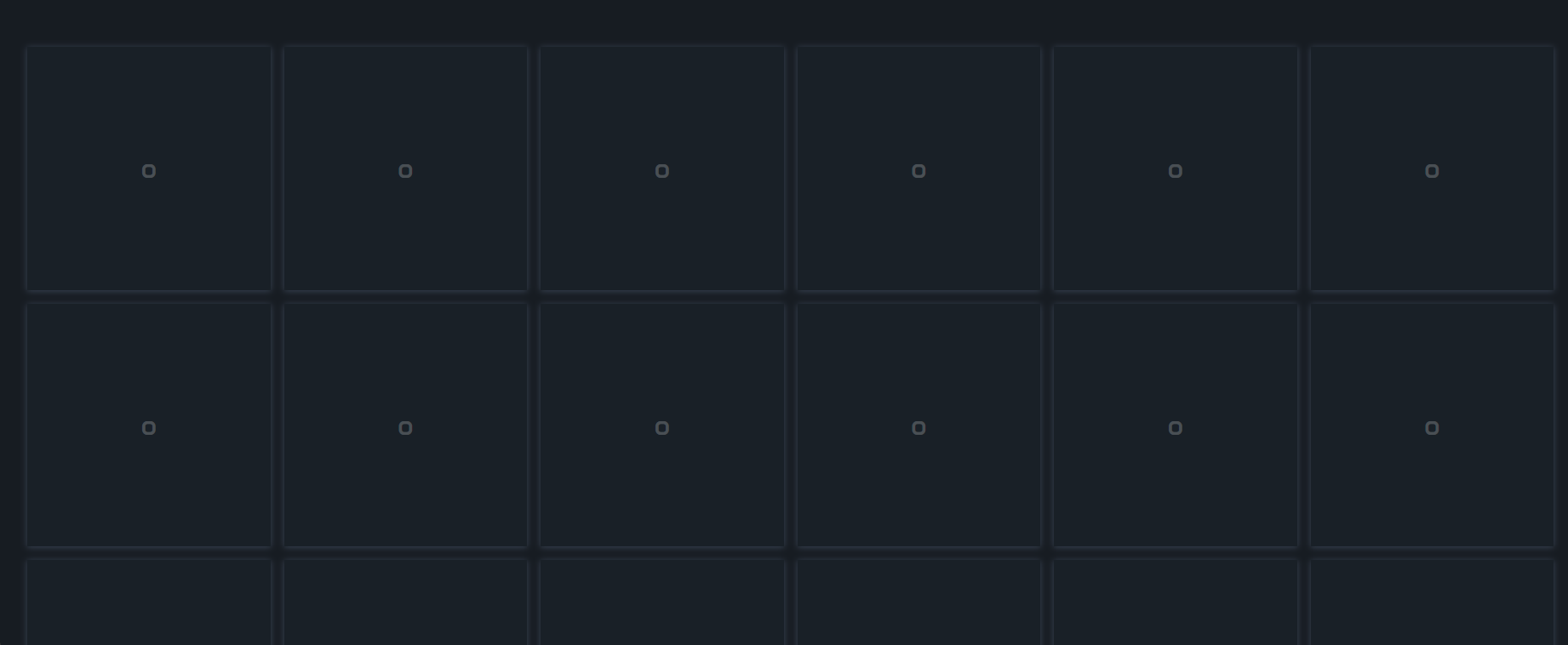
use clamp for the font
or u can set a minmax on grid items
u can try to separate the generateGrid into 2 functions
improve class names as well
you really need to stop worrying about this, unless you're running into issues
it's pretty far down the list of considerations for most webdevelopment project frontends
And multiplying by 0.5 is more performant than dividing by 2 :p
But such minimal gains are not worth the effort unless you know that this particular thing is your bottleneck.
Write code that works first. Then, if things are slow, do some perf tests to see where the slowdown is. Focus on that part. Premature optimisation is just wasted time