I don't really understand the registering slash commands part of the guide
It says to put this codeinside a
deploy-commands.js
script9 Replies
- What's your exact discord.js
npm list discord.js
and node node -v
version?
- Not a discord.js issue? Check out #other-js-ts.
- Consider reading #how-to-get-help to improve your question!
- Explain what exactly your issue is.
- Post the full error stack trace, not just the top part!
- Show your code!
- Issue solved? Press the button!then on another page, it says to put this code in index.js
and some of that code looks the same as the first script I sent
What don’t you understand? Do you get an error when running that code or what do you want to know?
I don't understand why it says here to put this code in index.js
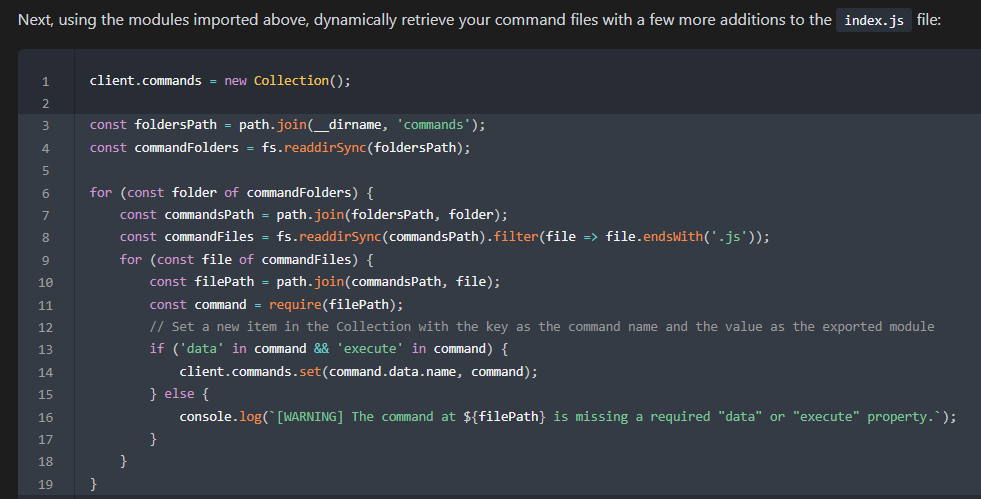
but here it says to put almost the exact same code in
deploy-commands.js
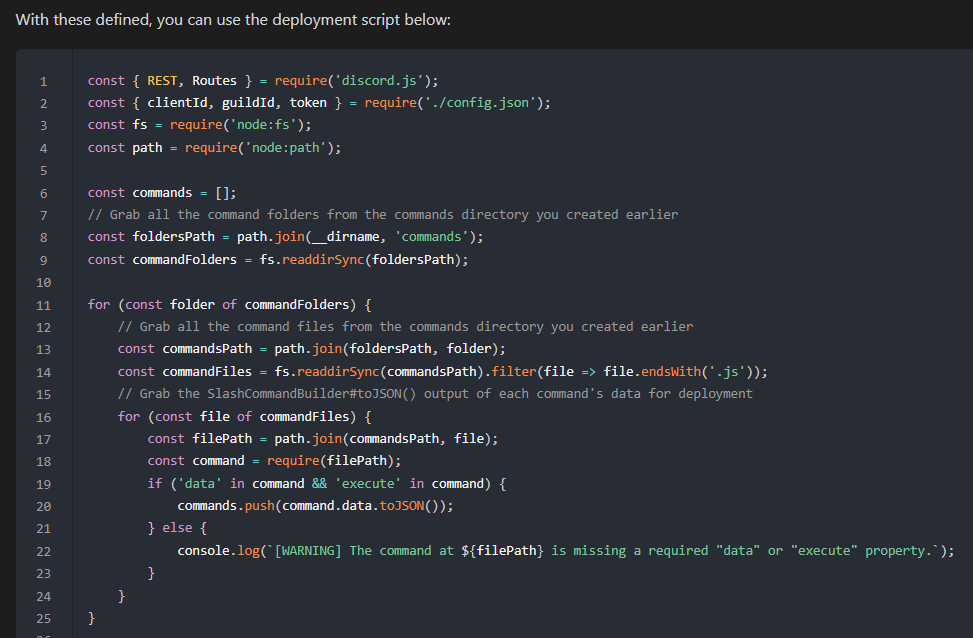
https://discordjs.guide/creating-your-bot/command-handling.html#loading-command-files
This guide from the official website doesn’t say anything about an deploy commands file
discord.js Guide
Imagine a guide... that explores the many possibilities for your discord.js bot.
On the "Registering slash commands" page it does
They do both go through all commands in the commands folder, but the deploy commands script registers the command to discord, while the index.js script only adds said commands to a Collection in the client
I'm also a noob, but what I understood till now is initializing slash commands (this is done by the deploy-command.js) for your bot in Discord is a separate process then running them (this is done in the index.js).
Due to this you might initialize commands in a deploy-command.js, for which you don't have a function yet in index.js. So you could see the command in Discord, but it would throw an error if you click on it.
The tutorial solves this elegantly by having the initialization code together with the executable code. This is why they run through the same folders. The deploy-commands uses the data: part of the slashcommandbuilder, and index.js the async execute(interaction) {} part.
I hope I'm somewhat correct 😄
PS: my little struggle: if you want to change the initialization parameters for your slash command (for example: you addStringOptions in the data: part), then you will need to run deploy-commands.js for them to be visible in discord.