multiple event listeners
so this is the code i have
for some reason the
end
listener count is 2?, and it seems to increase every time i speak?17 Replies
- What's your exact discord.js
npm list discord.js
and node node -v
version?
- Not a discord.js issue? Check out #other-js-ts.
- Consider reading #how-to-get-help to improve your question!
- Explain what exactly your issue is.
- Post the full error stack trace, not just the top part!
- Show your code!
- Issue solved? Press the button!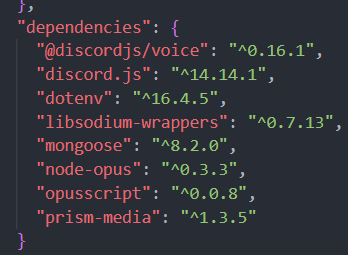
Maybe because you're not removeAllListerners'ing?
Could you give this a try?
Also, not related directly to djs/voice, but anyway
already did doesnt work, but that suggestion doesnt even make sense to do cuz why would i want to remove the listeners when im not even the one creating a new listener?
Depending of djs/voice, it can re-utilize the same EventEmitter and cause that
not sure abt it but isnt the object returned by
receiver.subscribe
a part of djs/voiceIf this is true, yep
It's a Node.js EventEmitter
ok wait lemme you give my mental model of whats happening, the code is creating a object with the
receiver.subscribe
by passing a userId and a end param, this object is named opusStream
and then im pipeing the data being recieved from this stream into a decoder, the decoder then emits data chunks whch i accumulate in the buffers
array, then when the conditions for the end
param are met the opusStream finally runs the callback function passed into the end
event, and once this is executed somehow the end
listener is doubled
that does not make any sense..Nvm, it's 2 because of the decoder
Didn't see it there
It's mostly also reading the end event
oh huh ok but in that case everytime i stop speaking the end event should only run twice am i right?
but everytime i speak it doubles from last time
Probably because djs/voice re-utilizes the same EventEmitter
for example i just started the bot
i speak then stop speaking: end runs 2 times
i speak then stop speaking: end runs 4 times
....
(Probably === I'm unsure if djs/voice does that, but if it does, it's because of that)
yea it most likely does but that just seems like a design flaw bcs who would want that?
hmm that makes things alot harder
Not really, but the opposite
It boils to you to clean the listeners anyway, as it's not djs/voice internally that is listening to it
It's far better than keep re-creating emitters
I imagine it's more for performance
it's not as if there needs to be multiple streams containing the same data, nor should djs/voice need to copy the data to a new stream for you
if you want multiple streams containing the same data, you are free to copy the data yourself
the flip side is that it's also up to you to determine whether you've already subscribed to a user's audio (and added event listeners to the stream)
.. so yeah, you'll need to remove the listeners