Flask Socketio wont work in tunnels
/ works and shows hello world but /time1 shows real time counter header but not counter: number
this is the html:
on localhost it works but on the tunnel it doesnt work
39 Replies
here are some screenshots:
https://cdn.discordapp.com/attachments/860930259179929640/1217533566871932998/CYY9JBg.png?ex=66045f7f&is=65f1ea7f&hm=3caa57e82f2341400f1956c2e1dd54d184ff34b34e7a48db680ab895af18a087&
cloudflare tunnel of localhost:8000:
localhost:8000:
https://cdn.discordapp.com/attachments/860930259179929640/1217533541177364581/3uIGJaP.png?ex=66045f78&is=65f1ea78&hm=0b635ce8bf81dfd2bda8ad6d3a117a40ea0b6d7199870e1c519c13d3e579d35b&
the counter isnt showing up or working in the cloudflare tunnel but its working in the localhost, did i setup sockets wrong or smth?
Does it work with quick tunnels? If so then do you have sockets enabled for your cloudflare zone?
I quickly recreated and it works fine with quick tunnels for me
sorry im a beginner, are quick tunnels just the cloudflared --url http://localhost:8000 without an account or custom domain or anything? if yes then thats what im using for testing rn im running the py code in localhost it works fine, i run that command then i go to it it works but doesnt show the counter like in the screenshot above, and i dont know how to enable sockets i didnt do that no could you please tell me?
Yeah the quick tunnels are those and have websockets enabled. I can push my example code later with the steps I used.
but i am using wuick tunnels? and its not working and what if i dont wanna use quick tunnels and use the account tunnels and ok sure pls ping me when u do
Example: https://github.com/Cyb3r-Jak3/cf-community-content/tree/main/socket-io
Enable web sockets for a zone: https://dash.cloudflare.com/?to=/:account/:zone/network
i went to the link it showed the add site page? but i am currently using quick tunnels so idk what to do so i just left the page im not using a tunnel with my domain rn to go to the network and enable it, im guessing thats what i would do if i had one but either way i went to the repo and downloaded it i extracted it and ran main.py localhost worked i go and run cloudflared --url localhost:8000 i go to the link and it works then i go to /time1 and it stilll does the same thing i want it to work for quick tunnels right now for testing and also for normal tunnels
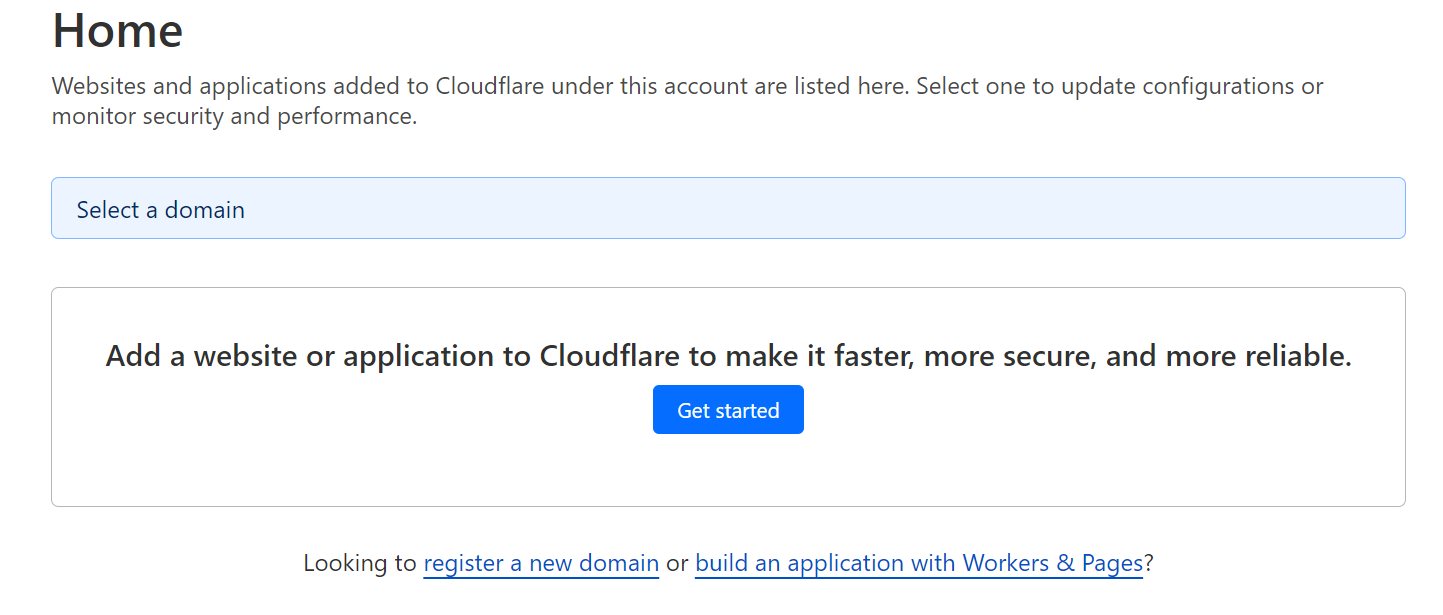
i tried reinstalling cloudflared again bec the first time i installed it alot using diff installers until it worked but the websockets still arent working and the link to enable websockets just leads to add site which i presume is for normal tunnels
Yeah the websockets link is only for your zone on Cloudflare. What do you see in your browser dev tools? Are all the requests showing either 200 or 101?
@efivg sorry for lack of ping
oh nws and sorry about the late replies but atleast discord i respond faster
for the tunnel? /time1?
this keeps happening over and over
when i opened the console
That’s weird. Does the browser log show it trying to connect via http?
wait not sure, what browser log exactly?
likee where do i find that
Chrome for Developers
Inspect network activity | DevTools | Chrome for Developers
A tutorial on the most popular network-related features in Chrome DevTools.
If you’re on Chrome
That will show all the requests being made
OH ye i am
the network tab ight ill see
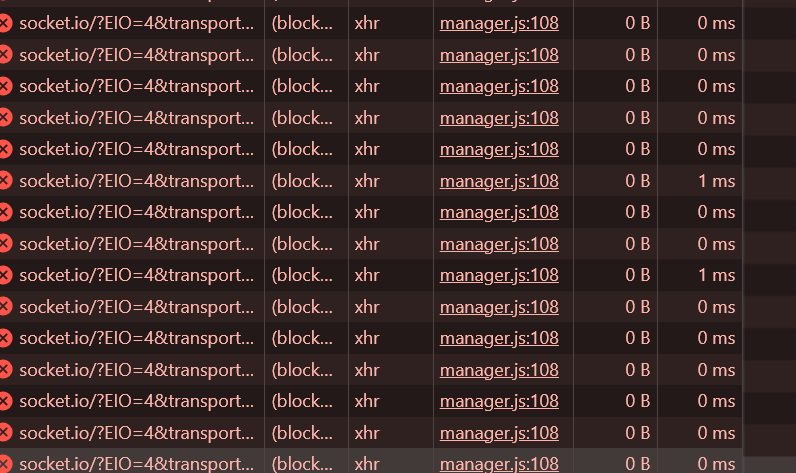
i can copy one and send it or smth
they all the same
Oh looking at the template file it is going to http. You should set that to use the protocol of the request
The
var socket
part.var socket = io.connect('http://' + document.domain + ':' + location.port + '/counter');
this?
what should i change to make it work?
Replace the
http
with location.protocol
like this?
var socket = io.connect(location.protocol + document.domain + ':' + location.port + '/counter');
or literally change 'http://' to 'location.protocol://'
cuz
var socket = io.connect(location.protocol + document.domain + ':' + location.port + '/counter');
didnt work
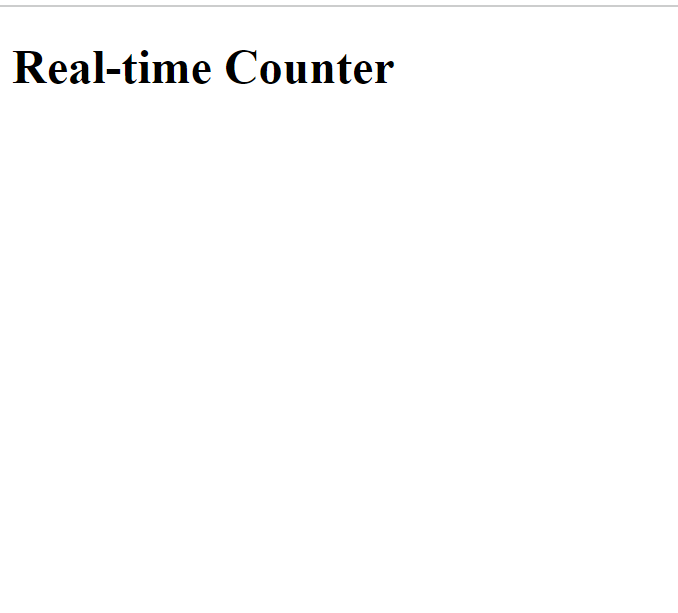
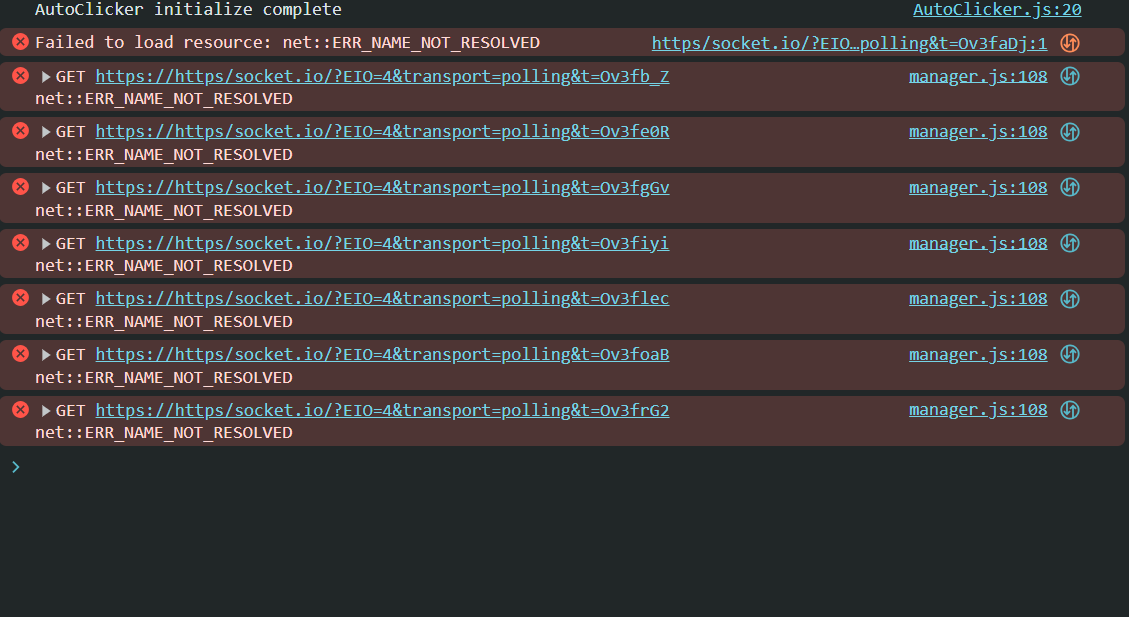
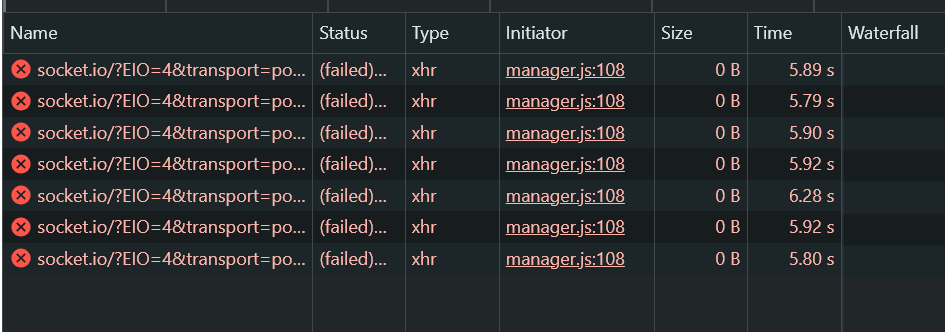
ye doing that didnt work either which i figured
oh wait
huh
one sec
ok nvm
i thought vs code didnt auto save but i think it was just visual
Can you double check the code because the URL it is trying to access is
https://https/socket.io
the html?
i think its right
this is the html from the repo but with 'http://' replaced with location.protocol
What happens if you try
Won't work with localhost but worth checking the tunnel
oki one sec
🤯 IT WORKED
OHH THANK U SM
THAT DOES MAKE SENSE
@Cyb3r-Jok3 thank u smm
🎉
THATS VERY AMAZING FR
do i close this now?
i dont wanna be rude and close it all of a sudden
Just mark it as solved and it can let it close itself out
U HELPED ME SO MUCH
alrightt
thank youu
oh @Cyb3r-Jok3 can i ask u smth else real quick i think it will be short or would it be preferred i make another ticket?
Feel free to just ask in here
ight thx so
smth like this doesnt work with quick tunnels im assumin cuz of the domain but when i use actual tunnels with my domain subdomains would work? cuz rn it just says
You'd need to make a DNS record that is
sub.<tunnel domain>.com
. One thing to keep in mind is that Cloudflare Universal SSL only covers first level subdomain unless you purchase ACM. So sub.domain.com
✅ but sub2.sub1.domain.com
❌where would i need to add the dns record? like under my domain make a dns record for sub.domain.com? and what tyoe if record if thats the case alsoo.. i dont plan on doing sub.sub anytime soon but curious, whats ACM ik like SSL and that sub.sub would say not secure but idk what ACM is IM very new so sorry
sorry if there is some common sense im missing...
It would be a CNAME record to the tunnel. If you use remote tunnels and add the subdomain as a public hostname to it then it will auto make the record.
ACM is advanced certificate manager which gives you more control over the certificates that Cloudflare issues for your zone. Without a certificate covering
sub.sub
you will see an error in browser that no certificate workedohh
mm so sub.domain.com would have a cname record to domain.com? if im getting it right?
or vice versa?
Correct
okayy thank you very much!
have a good day/nightt