Need help with joined tables
Hi,
I have some relations in my data schema. I have the type "questionnaire" which is linked to an entity called project:
entity Questionnaire {=psl
id String @id @default(uuid())
...
project Project? @relation(fields: [projectId], references: [id])
projectId Int?
...
psl=}
That works fine in the database as I can observe in the db studio.
In mein queries.ts I made this query:
export const getQuestionnaires: GetQuestionnaires<void, Questionnaire[]> = async (_args, context) => {
if (!context.user) {
throw new HttpError(401);
}
return context.entities.Questionnaire.findMany({
where: {
userId: context.user.id
},
**include: {
project: true,**
},
orderBy: {
createdAt: 'desc',
},
});
};
That also works fine. In a console log, I perfectly see my project object as part of my questionnaire array. (see screenshot)
Now the problem (see second screenshot): when I iterate over this array, IDE says that there is an issue with the type.
I am not even sure if its an WASP issue or a simple TS/React thing where I fail to solve.
Thank you for your help π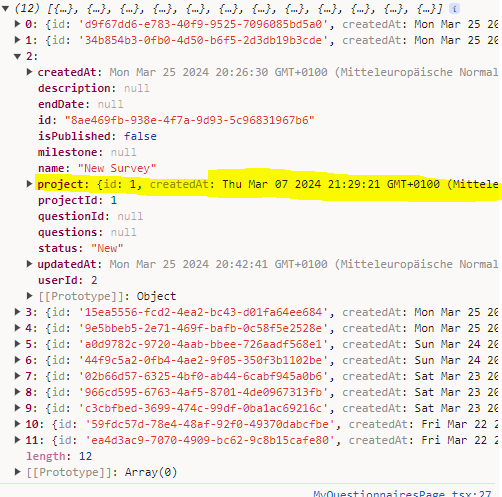
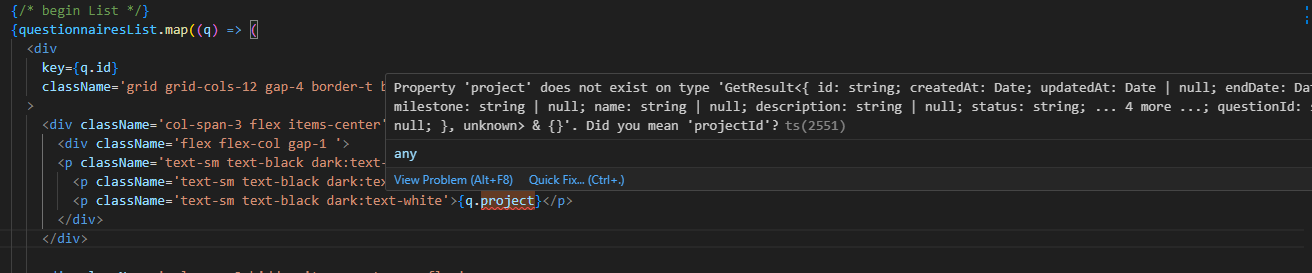
8 Replies
We honor the return value you specify in
GetQuestionnaires<void, Questionnaire[]>
specifically, the return type here is set as Questionnaire[]
Which means you are telling Typescript "hey expect just a list of questionnaires". Questionaire
normally doesn't have { project: Project }
included!
Solutions
The way you solves this is two-fold:
- Either put a type like this: GetQuestionnaires<void, (Questionnaire & { project: Project })[]>
. Here you are being explicit about the { project: Project }
part.
- Or ... Don't put any return type! And let Typescript infer the return type from the object you actually return from the function.
π The second option is my favorite TBH. Just put GetQuestionnaires<void>
(not specifying the second type) and the thing just works π
Try doing it and let me know if it works for you πThanks for your quick support. Unfortunately it does not work on the first try.
Your first solution throws an error in the queries.ts (see screenshot 1)

The second solution works out in the queries.ts. Here, I have the problem that it has an unknown type which is not working with my code.
Sorry, I am still very new into coding.
Thanks and good night π
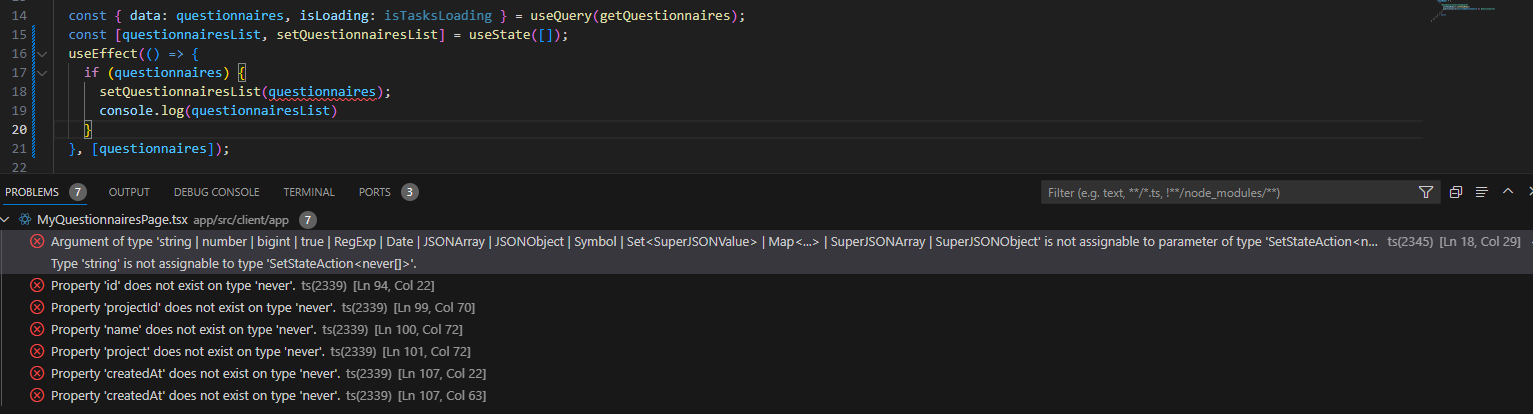
@Filip could you maybe help with this bit? I'm sure this should've work π€¨
Hi @mindreaderlupoDO,
The second solution Miho suggested needs a little tweaking to work. If you want TypeScript to infer the correct type, you must use the satisfies keyword:
If don't want to define a new type for the Query's return value, the new
satisfies
keyword will allow TypeScript to infer it automatically.
In your case, the code should look like this:
Check this message for a detailed explanation: https://discord.com/channels/686873244791210014/1186410195732140073/1186603845418237952
Let us know if this fixes the issueThanks for this input and also the Link to the other thread (shame on me that I didn't found it before).
But unfortunately I still did not manage to make that code runing.
```js
export default function MyQuestionnairesPage() {
const [showModal, setShowModal] = useState(false);
const [activeQuestionnaire, setActiveQuestionnaire] = useState<Questionnaire>(); const { data: questionnaires, isLoading: isTasksLoading } = useQuery(getQuestionnaires); const [questionnairesList, setQuestionnairesList] = useState([]); useEffect(() => { if (questionnaires) { setQuestionnairesList(questionnaires); console.log(questionnairesList) } }, [questionnaires]); ´´´
const [activeQuestionnaire, setActiveQuestionnaire] = useState<Questionnaire>(); const { data: questionnaires, isLoading: isTasksLoading } = useQuery(getQuestionnaires); const [questionnairesList, setQuestionnairesList] = useState([]); useEffect(() => { if (questionnaires) { setQuestionnairesList(questionnaires); console.log(questionnairesList) } }, [questionnaires]); ´´´

Here I tried the approach with satisfies. the queries.ts file works without any issue. but in the implementation with useQuery, I am stuck
... Wow, after failing over 3 hours this afternoon, I finally got it...
My solution (based on the linked thread solution 2):
import { QuestionnaireWithProject } from '../../shared/types';
const [questionnairesList, setQuestionnairesList] = useState<QuestionnaireWithProject[]>([]);
useEffect(() => {
if (questionnaires) {
setQuestionnairesList(questionnaires as QuestionnaireWithProject[]);
console.log(questionnairesList)
}
}, [questionnaires]);
and in the queries.ts:
export const getQuestionnaires: GetQuestionnaires<QuestionnaireWithProject[]> = (async (_args, context) => {...}
Thanks again for the suport!
You're welcome!
We're here if you need anything else π