Using collector.on('end') to edit reply?
I'm trying to create a polling bot that edits their reply once the poll has concluded, but i can't figure out how to do that, and was wondering if anyone had any advice? I'm not sure if I should figure out a way to do it through collector.on('collect') or if there is a way to do it with collector.on('end'). If the right way to do it is through collector.on('collect'), i'm not sure if i can combine a switch statement with an await 60 _ 000 to update the message when the timer has ticked down
22 Replies
- What's your exact discord.js
npm list discord.js
and node node -v
version?
- Not a discord.js issue? Check out #other-js-ts.
- Consider reading #how-to-get-help to improve your question!
- Explain what exactly your issue is.
- Post the full error stack trace, not just the top part!
- Show your code!
- Issue solved? Press the button!
- ✅
Marked as resolved by OP
for reference this is my code
You can just set the timeout to
60000
and use
yuh but i tried doing i.update(0) and i.editReply() but both give me an error
i shouldve mentioned that
my bad
"an error" is pretty vague. What error did you get?
neither i.update() nor i.editReply() are actual functions, and im pretty sure its cause i is an object map
You should edit the original interaction of the command instead, I don't believe the 'end' event callback has an interaction since the collector has ended
fair point, i have this line which sends the original embed:
const response = await modalSubmission.reply({ embeds: [createPollEmbed(votes)], components: [row], fetchReply: true });
and i tried updating it with response.editReply, but that is also apparently not a valid function (i got editReply from this: https://discordjs.guide/slash-commands/response-methods.html#ephemeral-responses)
discord.js Guide
Imagine a guide... that explores the many possibilities for your discord.js bot.
also if i try interaction.editreply
" throw new DiscordAPIError(data, "code" in data ? data.code : data.error, status, method, url, requestData);
^
DiscordAPIError[10008]: Unknown Message"
1. just making sure you're aware that the native polls beta launched last week https://discord.com/channels/222078108977594368/992166350640386090/1220461677364117626
2. the
end
event does emit with a Collection
of the collected items, so it does contain the previous interactions
you'd need to pick one (<Collection>.first()
just being the first one if it doesn't matter)i did not know about native polls 😅 i'll def take a look
the collected items are interactions to buttons
i really only wanna edit the message itself
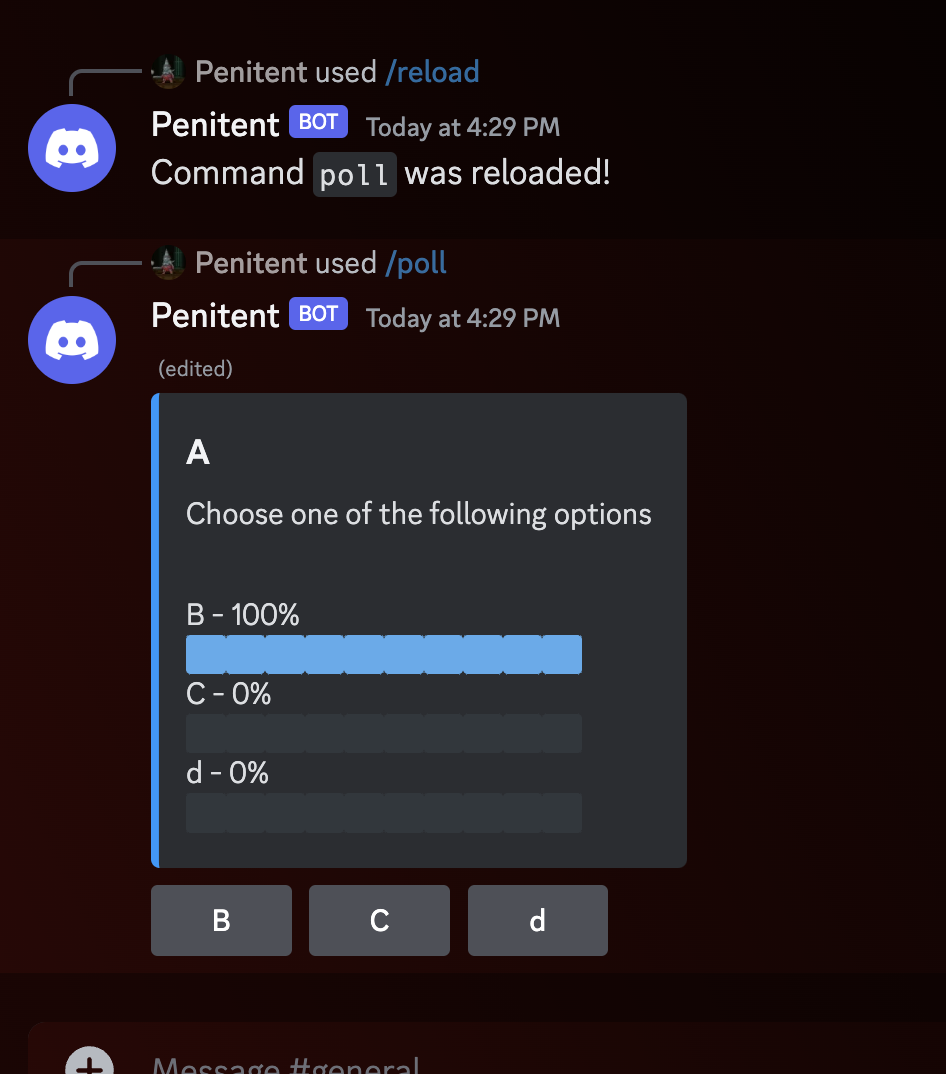
I wanna take this message and update it with saying "the poll has ended, B has the most votes with 100% of the votes"
or something like that
yes, but while
i.editReply()
doesn't exist because i
is a Collection
, <ButtonInteraction>.editReply()
does existahhh okay, that makes sense, so then it would be i.first().editReply()?
assuming it does collect an interaction, yes
ahhh it works! however it doesnt remove the embed
just leaves me like this for some reason:
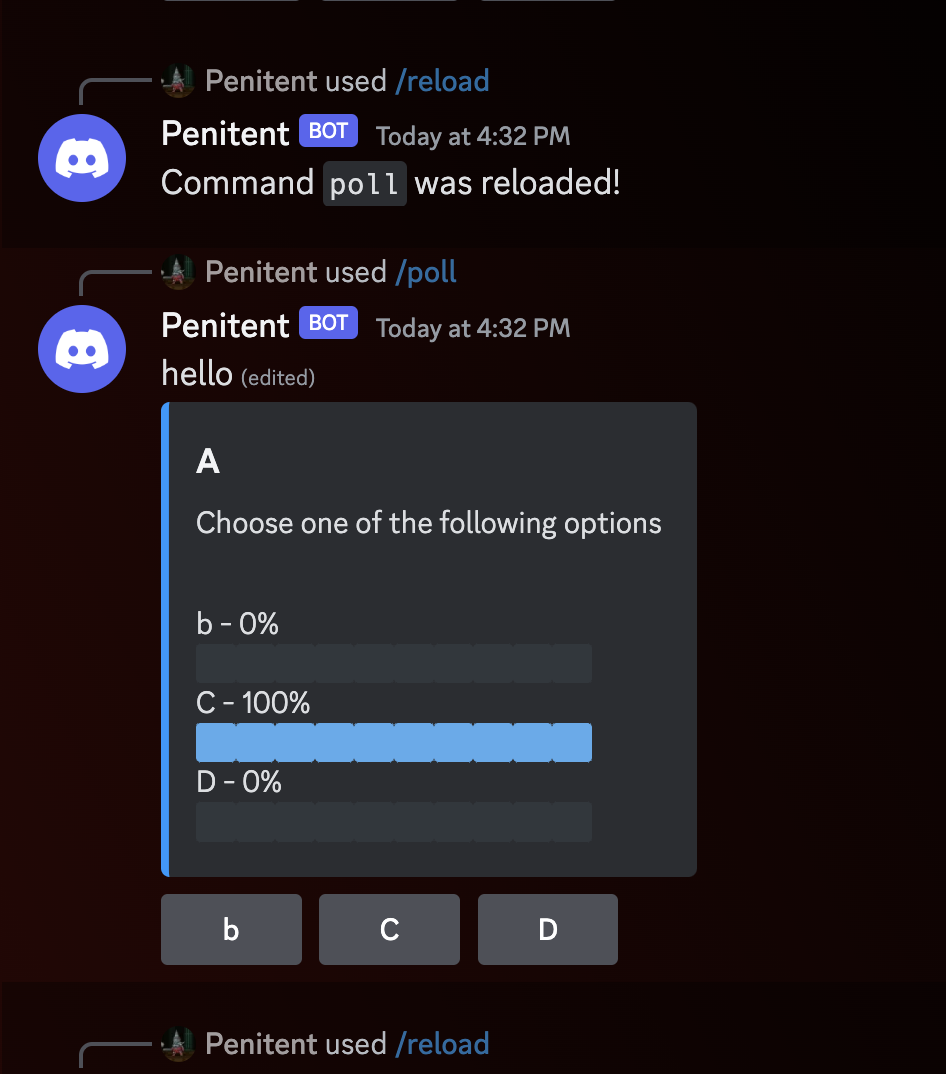
are you passing an empty array for
embeds
?
care to share your updated code?
its an array of only 1 element
not sure what you mean by it's an array of only 1 element
to remove all embeds from a message when editing, you should pass an empty array for
embeds
it does not edit properties you don't specifyahh sorry i misunderstood
i thought you were asking if in the initial interaction i was passing an empty array for embeds and then populating
sorry
got it, it works now! thanks @duck