How to increase the quantity on an item that already exists in my shopping cart?
Yo!
I'm trying to figure out how I go about increasing the quantity of an item that already exists in cart/page.jsx, instead of it adding that same item again as a new element each time, but I can't figure it out.
the repo is here:
https://github.com/callum-laing/shopping-site
17 Replies
I would put cart item in separate component, and then have state inside to handle quantity. Something like this:
btw I haven't tested this so there might be some bugs
Also I noticed you're using Next.js, so you should know
components/
directory should go outside of app/
directory. Right now you're creating "/components" route, which I guess you don't wantI think i've set it up all wrong tbh lol
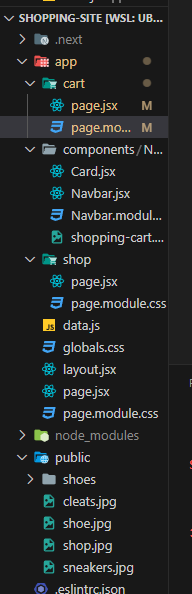
first time using nextjs
you mean like this?
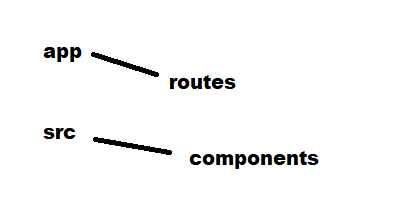
I'm kinda too deep into this to try and figure out what I pull from cart.jsx, to put into the cart component, it's all getting very uhh... confusing.. 😅
This is example how it should look like. I'm not sure what
Card.jsx
is from Navbar/
. If it's component that is used in multiple places that you should put it in components/
, but if you're going to use it only in Navbar/
then you can create it there as well.you know what, I Navbar folder shouldn't even be there, I'm not entirely sure why it's there, how it got there, and how to get rid of the bloody thing lol
What do you mean? Why not?
it seems to be joint into the components folder? I can't see why lol
I don't see a problem lol.
If you mean why is it folder and not just file you can do both. By making it as folder you can put all stuff related to Navbar inside of there. And have
index.jsx
where you would put component itself. Then when you import it you would do
Just like if it was file Navbar.jsx
I thin that is what I was trying to do but for some reason it now looks as "components / Navbar"
Let me tidy up the filing here, it's quite the mess 😄
Go for it
That looks better
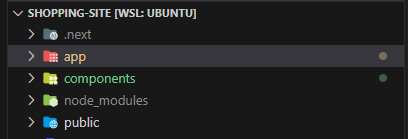
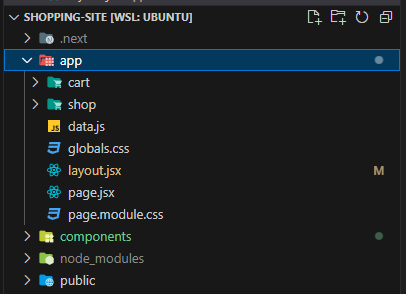
Ok sorry, back to the original point, so is it quite messy to arrange the quantity to change if I keep everything inside app/cart/page.jsx? i tried your way of creating component/cartItem.jsx and moving it, which makes sense, but I got a little lost on what I remove from cart/page.jsx to make this possible
Just
inscreaseQuantity
and decreaseQuantity
. I believe the rest can stay in cart/page.jsx
.
You can either move them to CartItem
and make them work with your new component or you can use my handleQuantity
function.I'll try adding the function into the current page for now, and if I get that to work i'll try and refactor it later, as I'm not the best at react still lol