Unable to make a POST request to retrieve a token with `grant_type` of `authorization_code`
I'm attempting to follow the "Quick Start" guide on signing in users. I have successfully retrieved the
CALLBACK_AUTHORIZATION_CODE
, but when I attempt to request the token I get a 400 error.
I'm following this documentation: https://kinde.com/docs/developer-tools/using-kinde-without-an-sdk/#handling-the-callback
The request I'm making is to:
I have triple checked that each of the credentials being sent is correct, yet I still get a 400 response with no error message in the body of the response.
Note: Probably not super important, but my backend is written in Go, although I can't seem to get it to work even with a basic curl request:
Kinde Docs
Using Kinde without an SDK - Developer tools - Help center
Our developer tools provide everything you need to get started with Kinde.
21 Replies
Here is my backend code in case anybody noticed what I'm doing wrong:
It errors on
if res.StatusCode != http.StatusOK {
with:
I've also tried parsing the body, and it's always emptyHere's a node example:
Can you try passing that through and see if you get the token back?
@VKinde Yes I've tried this in postman and it does work for me. Isn't this for an M2M token though? I need the user token
Oh, but it doesn't work with
authorization_code
That should be just for the access token itself, not specifically tied to M2M
It only works when the
grant_type
is client_credentials
Sorry, you have to pass the
&code=<CALLBACK_AUTHORIZATION_CODE>
at the end of that request
This is what I tried just now
Can you double check and make sure you're passing the correct authorization code in the param?
Just generated a new one, double checked and same issue
I can't help but feel like I'm doing something stupid since I get no response body from the 400 res
Here is the verbose curl output:
FYI: The app I'm using is of type "Back-end web"
Ah okay, so I tried with the node example, I'm getting an error message now

Okay, I've finally managed to get a token response in JS, just need to figure out what it's doing differently, thanks @VKinde
I'll update this thread if I figure it out
Okay I've figured it out. Turns out URL query params are not sufficient to pass the data effectively to Kinde.
Instead the params need to be sent as form data. In Go, this looks like:
Full example for anyone that finds this thread:
Awesome to see that you were able to figure it out @jamie , would this guide been of help? https://kinde.com/blog/engineering/how-to-use-kinde-with-golang-oss-libraries/
Kinde Blog
How to use Kinde with golang OSS libraries
Learn how to use Kinde with golang OSS libraries
@Andre @ Kinde I came across this article really early on actually.
This article does provide useful insights into how to connect to Kinde via an M2M app, but didn't help me to understand how I can retrieve an access token for a specific user.
Having implemented both now, I may see if I can borrow ideas from this article to produce a small Go library for this.
One of the main technical differences between them is the article specified uses client_credentials, whereas the quick start recommends using authorization_code.
The implementation on the article relies quite heavily on client_credentials and so didn't seem helpful at the time.
Amazing, thanks for the feedback @jamie I will pass this along to the team. We are working on a Go SDK atm and should hopefully have it out soon.
Yes I saw, let me know if I can help at all
Amazing, I will let the team know
@jamie the golang SDK is in a non-shareable state yet, which parts are you interested in contributing to?
@ev_kinde Specifically I'd like to contribute to anything that goes towards fully implementing Kinde's API as a golang SDK. Ideally I'd like to try to abstract some of the functionality to make it more like idiomatic Go, and eventually hook into that abstraction when exposing webhooks (I realise this is a beta feature, but when released it would be nice to take advantage of them in the same lib).
We're using it in our Go API so I've already implemented the very basics, but moving forward we want much more complete integration with Kinde.
If the setup was simpler and documented I think I'd have been up and running in 30 minutes, but since there wasn't anything Go specific, I ended up spending maybe a couple of days figuring it all out start to finish.
It would have been useful to know that the information sent to the
/oauth2/token
endpoint needs to be POST form data, the doc is a little misleading as it appears that I could perhaps make a curl request (with encoded query parameters) using the format shown in the code example: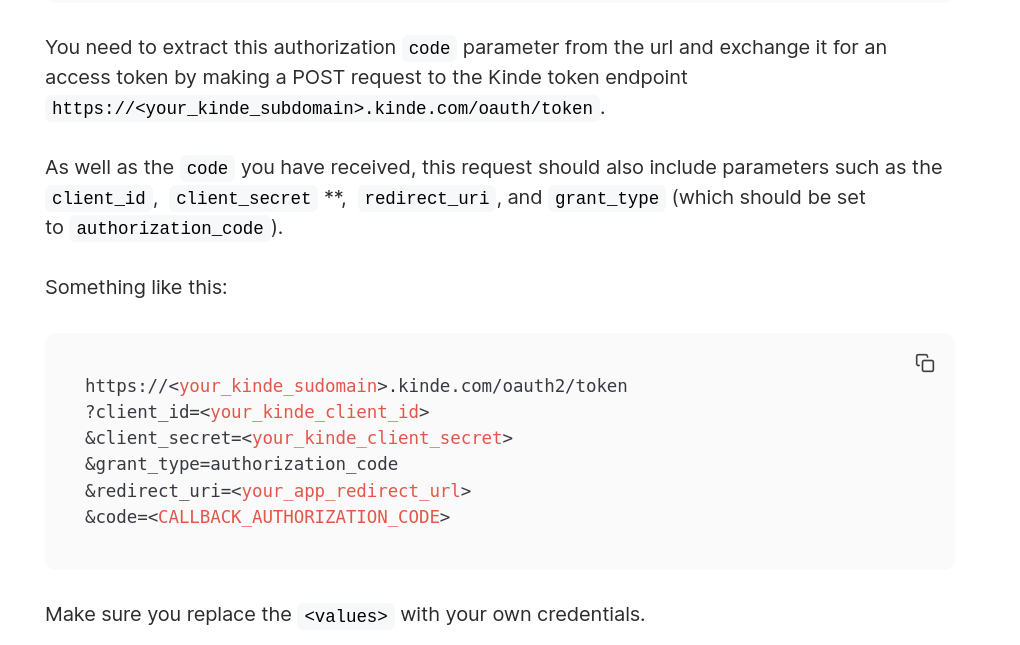
Maybe it would be worth looking at what a curl command for that might look like?
I'll have a go
Maybe you could just shorten it to this:
even if it was just on another tab in that code block, it would've saved me over a day on setup
That screenshot is from here "Using Kinde without an SDK - Handling the callback": https://kinde.com/docs/developer-tools/using-kinde-without-an-sdk/#handling-the-callback
Kinde Docs
Using Kinde without an SDK - Developer tools - Help center
Our developer tools provide everything you need to get started with Kinde.