How to generate first frame of video as image?
Hello. I'm working on social network app with Next.js and MongoDB. Users should be able to post images and videos as content (btw all posts in DB have
content
field which is array of links to that content, which is either image or video).
I'm facing an issue when trying to display posts to the user. I have a <PostCard />
component which renders first element of content
as "thumbnail" and problem occurs when first element is video, which obviously can't be rendered as image. So I was thinking, if first element is video, it should somehow take first frame of that video and use it as thumbnail. But it would probably be bad to render it every time user visits the page, so I was thinking of generating it once when user creates a post, and save it as something like poster
as separate field in DB. But I have no idea how to do that lol.
Any suggestions/advices/opinions?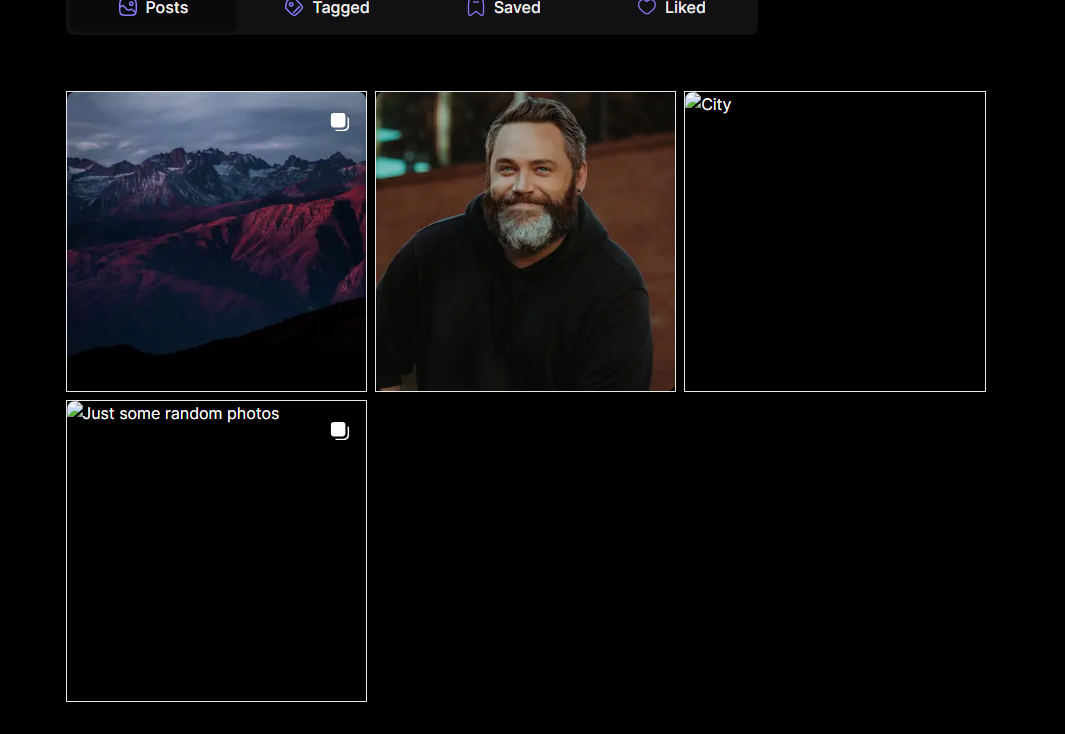
31 Replies
you can use the fluent-ffmpeg package
apparently, all you need to do is this:
Doesn't seem to work for me. Here's what I got:
main.js:
And I am getting error in console
try the screenshots
LOL
installl ffmpeg
I did? 😂
that's not ffmpeg
https://ffmpeg.org/download.html
you need that
i recommend you to use this on linux instead
And how would that work on my app?
Would that work when I deploy it?
you can set the ffmpeg path with this
well, generate it before you deploy
this is important because deployment runners don't have much/any storage, usually
That's not what I want. I need to generate first frame as image from video that user uploads. So I have
input[type=file]
where user uploads video he wants to publish on my app. Then I store that video on uploadthing (I get URL to video as response), then I take that stored video, take first frame and store it to uploadthing again. So in my DB I'd have something like
i would expect uploadthing to do that automatically
That's how I planned it out, maybe there's a better way
if it doesn't, that ... eh
Well it's just for storing files lol
i know, but it could generate previews too
but i get why it doesnt do that
you can generate the frame in js
no idea if they're functional, and I've never used them, but there are ports of ffmpeg to js / wasm
canvas can read a video
i though you wanted to generate it all and then deploy
what you want is kinda easy in the browser window
https://developer.mozilla.org/en-US/docs/Web/API/Canvas_API/Manipulating_video_using_canvas <-- this is an example of it
but, to handle more codecs, a wasm ffmpeg is a good idea
I found few example of that. But most of them are done differently so I got confused lol. I'll have to look more into that.
Just to make sure... It can work with
File
from JS right?basically, the canvas 2d context reads the contents of the video
it should
Stack Overflow
How to play video from input field in html
I want create a page that allows a user to choose a video file from their local machine, and then have that video play on html. I have each separate portion figured out, but how do I get them to in...
if the video plays, then it should be usable for the canvas
Sweet, thanks for help
you're welcome
I seem to love to overcomplicate things 😂
Here I am saying of generating image from video as user uploads it, saving it as separate field in DB etc when I can just check if content URL ends with video or image type and based on that render image or video lol.
I forgot video will just act like image if
controls
, autoplay
etc isn't provided to it lolthat won't work
videos usually have just a black backdrop before they load
you can set it to autoload the metadata, which usually renders the first frame too
It works fine though
Might be because I'm using Next.js, and it's code is rendered on server and HTML code is sent to client
is the video playing automatically?
it's not playing at all
acts like regular image
that's good
i guess it is loading just the metadata
it works, it's all that matters lol imma enjoy success while I can, there might be problems with this approach later
bandwidth is one that comes to mind
images can be lazy loaded
that video will load imediately
true
I'll find better solution later.
the simplest one is to do what i've shown you
reading the video into a canvas is super easy