Kinda lost on how to handle errors on thrown on server properly on the client
i'm doing some validation on payload of the a login api i've got using, and i check the instance of the error being thrown to get something to work with on the frontend, but its the 500 error that's being thrown instead, i'm loosing my mind
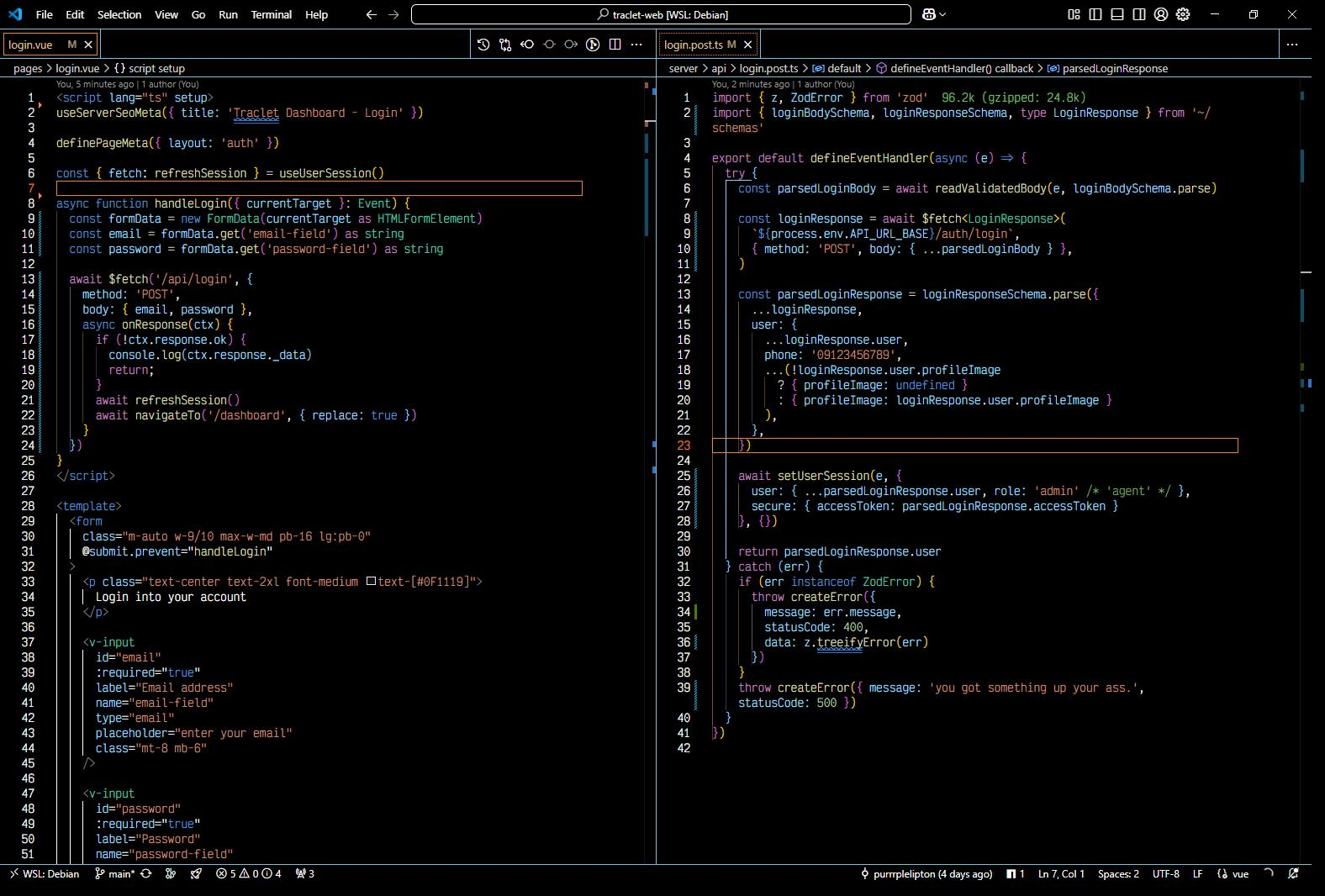
8 Replies
Hi @Tobi. I'm kapa.ai, your friendly support bot. I will try to answer your question by searching through the knowledge base. :wave:
----------------------
When handling errors thrown on the server in Nuxt (for example, from an API route), it's important to use Nuxt's built-in error utilities to ensure that meaningful error information is passed to the client.
How to Throw Custom Errors in API Routes
Instead of just throwing a generic
- Use
Error
, use Nuxt's createError
utility in your server API routes. This allows you to set a custom statusCode
, statusMessage
, and even a data
property for extra details:
See docs example
How to Access the Error on the Client
When you use composables like useFetch
or $fetch
on the client, you can catch the error and access the properties you set (statusCode
, statusMessage
, and data
). For example:
See createError API docs
Important: If you throw a plain Error
or don't use createError
, Nuxt will default to a 500 Internal Server Error, and you won't get your custom message or data on the client. Always use createError
for custom error handling in API routes.
Passing Custom Data
If you want to pass additional data to the client, use the data
property in createError
. This is the recommended way to send structured error information to the frontend, as the message
property may not always propagate from server to client in API routes, but statusMessage
and data
will.
In API routes, usingcreateError API docs --- Summary:createError
by passing an object with a shortstatusMessage
is recommended because it can be accessed on the client side. Otherwise, amessage
passed tocreateError
on an API route will not propagate to the client. Alternatively, you can use thedata
property to pass data back to the client.
- Use
createError
in your server API routes for custom errors.
- Access statusCode
, statusMessage
, and data
on the client in your error handler.
- Avoid throwing plain Error
objects if you want custom error info on the client.
If you follow this pattern, you should be able to handle and display server-thrown errors on the client as intended.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@642130694394478612> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
@kapa.ai are try-catch not a thing in nuxt's server api defineEventHandler thingamabob?
Let me check the knowledge base for you again. One moment! :books:
----------------------
Yes, you can absolutely use try-catch blocks inside Nuxt server API routes defined with defineEventHandler. This is a standard way to handle errors that may occur during the execution of your handler logic.
For example, you might see code like this:
This pattern is shown in community examples as well, where errors are caught and either logged or re-thrown as a custom error using createError, which is the recommended way to send structured error information to the client in Nuxt API routes see example.
So, try-catch is definitely a thing in Nuxt server API handlers!
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------
<@642130694394478612> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
@kapa.ai , alright i readValidatedBody using a zod schema and my error generates from there and checking the error instance in the catch block doesnt seem to be working
Let me check the knowledge base for you again. One moment! :books:
----------------------
When you use
readValidatedBody
with a Zod schema in a Nuxt server API route, if the validation fails, an error is thrown. However, the type and structure of this error may not be a standard JavaScript Error
or a Zod error directly—it is handled internally by Nuxt/Nitro.
The knowledge sources do not provide explicit details about the exact error instance or type thrown by readValidatedBody
. However, the Nuxt documentation shows that you can use a try-catch block around your handler logic, and you can throw a custom error using createError
in the catch block if you want to control the error response.
Here’s a pattern you can use:
See Nuxt server error handling
Key points:
- The error thrown by readValidatedBody
may not be a Zod error instance directly, so instanceof
checks may not work as expected.
- For consistent client-side error handling, always wrap and re-throw with createError
in your catch block, passing relevant details in the data
property.
If you need to access specific validation error details on the client, include them in the data
property of your custom error.
If you need more granular control or want to check for Zod errors specifically, you may need to validate manually with Zod and handle the result, but this is not shown in the official Nuxt documentation.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@642130694394478612> Kapa.ai is still learning and improving, please let me know how I did by reacting below.