[Vue warn]: Component <Anonymous> is missing template or render function.
Hi guys!
I have multiple warnings in Nuxt - because of the spline-viewer... not sure why I got them... I created spline-viewer.clitent.ts plugin + added rule in nuxt.cfg
export default defineNuxtConfig({
// plugins
plugins: ['~/plugins/spline-viewer.client.ts'],
// compiler options
vue: {
compilerOptions: {
isCustomElement: (tag) => {
return tag === 'spline-viewer';
}
}
},
})
Any ideas what else I should do?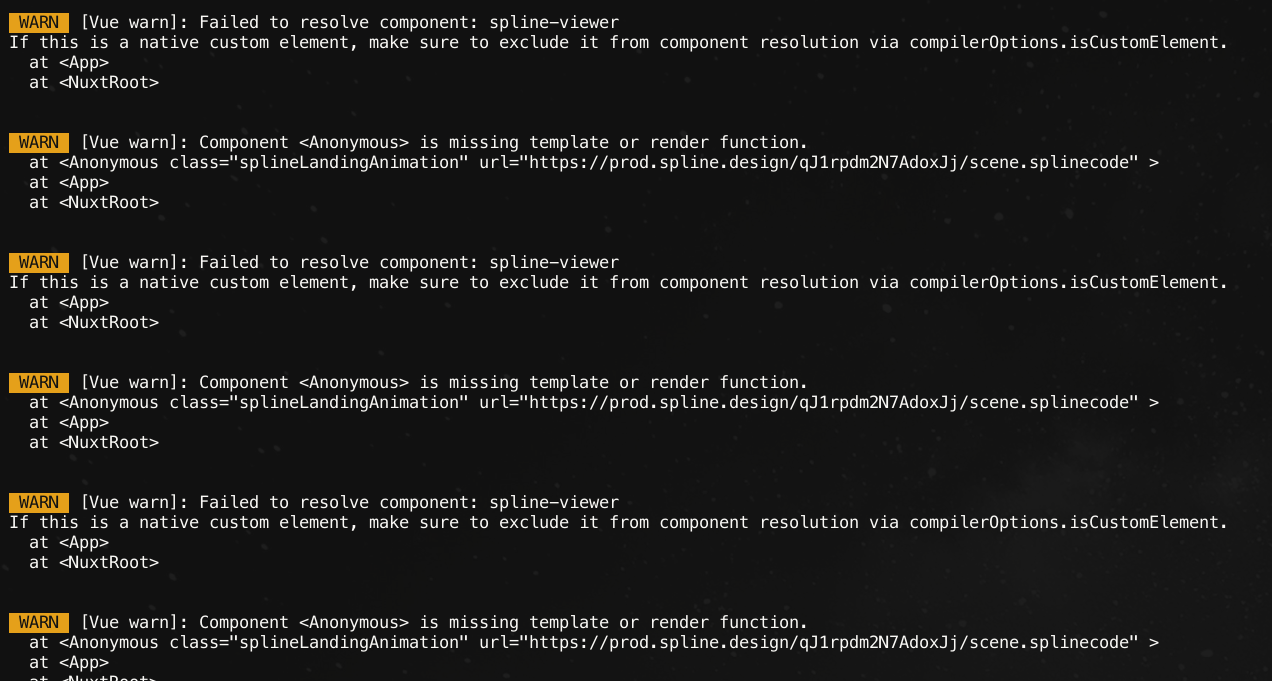
5 Replies
Hi @d4vv. I'm kapa.ai, your friendly support bot. I will try to answer your question by searching through the knowledge base. :wave:
----------------------
The warning
- Nuxt plugins directory structure
- Nuxt config plugins option ---------------------- Note: you can ask me a follow up question by @ mentioning me again :speech_balloon: ----------------------
[Vue warn]: Component <Anonymous> is missing template or render function.
typically means that Vue is trying to render a component, but it doesn't know how—either because the component isn't registered properly, or it's being treated as a Vue component when it's actually a custom element (web component).
From your config, you are correctly marking <spline-viewer>
as a custom element using compilerOptions.isCustomElement
. This tells Vue not to treat it as a Vue component, which is the right approach for web components.
However, regarding your plugin registration:
- In Nuxt 3, plugins placed in the plugins/
directory are auto-registered. You do not need to add them to the plugins
array in nuxt.config
unless you want to customize their order or include plugins from subdirectories that aren't auto-scanned. If your plugin is at the top level of plugins/
, you can remove it from the plugins
array for simplicity, but including it is not harmful if you want to control order or are using a subdirectory structure. See the Nuxt plugins documentation.
- Make sure your spline-viewer.client.ts
plugin is actually registering or importing the web component if needed. If the plugin file is empty or not importing/defining the custom element, Vue will not know how to render it, leading to the warning you see.
Checklist:
1. Ensure the web component is imported or defined in your plugin file. For example:
2. Confirm that the tag name matches exactly in both your config and your template.
3. If your plugin is in a subdirectory, ensure it's included in the plugins
array as described in the Nuxt config plugins section.
If you have done all of the above and still see the warning, double-check that the web component is being loaded on the client side and that there are no typos in the tag name.
If you provide more details about the contents of your spline-viewer.client.ts
plugin, I can help further. But based on the knowledge sources, these are the main steps to resolve such issues in Nuxt 3 when working with custom elements and plugins.
References:- Nuxt plugins directory structure
- Nuxt config plugins option ---------------------- Note: you can ask me a follow up question by @ mentioning me again :speech_balloon: ----------------------
<@357201234064572416> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
@kapa.ai Here is my spline-viewer.client.ts file output:
export default defineNuxtPlugin(() => {
if (process.client) {
const script = document.createElement('script');
script.src = 'https://unpkg.com/@splinetool/viewer/build/spline-viewer.js';
script.type = 'module';
document.body.appendChild(script);
}
});
Let me check the knowledge base for you again. One moment! :books:
----------------------
Thank you for sharing your plugin code. Based on your setup and the knowledge sources, here’s what’s happening and what you can check:
1. Plugin Loads the Script Dynamically
Your plugin appends the Spline Viewer script to the document body on the client side. This is a valid approach for loading a web component, and your use of
You have correctly set up
The warning
- Wrap your
As per the Nuxt 3 documentation, plugins in the
- Your plugin code is correct for loading the script. - Use
Your plugin appends the Spline Viewer script to the document body on the client side. This is a valid approach for loading a web component, and your use of
.client.ts
ensures it only runs on the client.
2. Vue Compiler OptionYou have correctly set up
compilerOptions.isCustomElement
in your nuxt.config
to treat <spline-viewer>
as a custom element, which is required for web components in Vue 3 and Nuxt 3.
3. Potential Issue: Timing of Script LoadThe warning
[Vue warn]: Component <Anonymous> is missing template or render function.
can occur if Vue tries to render <spline-viewer>
before the script has finished loading and defined the custom element. Since you are dynamically injecting the script, there may be a race condition: the component is rendered before the browser has registered the custom element.
Solution:- Wrap your
<spline-viewer>
usage in a <ClientOnly>
component to ensure it only renders on the client, after the script can be loaded.
- Optionally, you can add a check or a small delay to ensure the script is loaded before rendering the component, but <ClientOnly>
is usually sufficient in Nuxt 3.
Example:
4. No Need to Register Plugin in nuxt.configAs per the Nuxt 3 documentation, plugins in the
plugins/
directory are auto-registered. You do not need to add them to the plugins
array in nuxt.config
unless you have a specific reason to control order or include subdirectories Nuxt plugins documentation.
5. Summary- Your plugin code is correct for loading the script. - Use
<ClientOnly>
when rendering <spline-viewer>
.
- Your compilerOptions.isCustomElement
config is correct.
- You do not need to add the plugin to the plugins
array unless it’s in a subdirectory.
If you still see the warning after these changes, it may be due to the script not loading fast enough before the component is rendered. In that case, you might need to manage the loading state more explicitly, but usually <ClientOnly>
resolves this timing issue.
If you need more details on plugin setup or custom elements, see the Nuxt plugins documentation.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@357201234064572416> Kapa.ai is still learning and improving, please let me know how I did by reacting below.