function vs method
Anyone know of a good guide that explains the difference between a function and a method? I don't really understand when one should be used over another since, if I'm not mistaken, they're both functions at a base level.
C
Calego•973d ago
I don't know of any guides about this (also am interested if someone finds one), but I can answer your question by saying the words "Function" and "method" are used pretty interchangably in the javascript ecocsytem.
You could argue "method" refers to a "function which belongs to something (e.g. a
class
or other object)".
But in the end all methods are functions, though with the above argument not all functions are methods.L
LebombJames•973d ago
You could argue "method" refers to a "function which belongs to something (e.g. a class or other object)".This was my understanding, although I didn't think it could be that simple (nothing in programming is!) The main thing that kinda threw me off was args in relations to methods, I may have just not searched enough but can you have a method with args? Something like
thing.method(arg1, arg2)
?C
Calego•973d ago
Yep
So in your case
thing
might look like this:
which could just as easily be written like this:
For like 99.99999% of use cases those are identical.
Where 'method' really comes into play is with class
es and this
.
is different from the following:
(i'm like 60% sure about that, this
is confusing af and I work professionally in JS-land)
A general rule of thumb I follow is: "If this ever matters, you should restructure your code so that it no longer matters" 😅L
LebombJames•973d ago
This sounds like great advice! So
this
in the first example just points to the object/class the method is in? So is this.otherMethod()
effectively method.otherMethod()
or Thing.otherMethod()
?C
Calego•973d ago
I get better every time I explain this give me a sec
this
in the first example is 'effectively'* Thing.otherMethod()
* big caveat.
this
is "this instance of Thing
", where the syntax Thing.otherMethod
would call a static
method named otherMethod
on that class.
A method
could be defined as a function
which is a 'property' of another .... thing. In this case a class
.
confidence hovering around 80% for that example
I've never tried having the same named things in a class but some static and some notL
LebombJames•973d ago
OK, I've wrapped my head around that... just about. The only thing I don't get is why
Thing.bar()
and new Thing().bar()
call different things. My understanding is that ()
is used on classes for constructors?
I think I'm a bit out of my depth here 😅C
Calego•973d ago
gonna be honest that's the part I'm not super confident about. it might be that both call the static
bar
new Thing()
instantiates the class. Way to think about that is "Thing" may be a generic template, but when you instantiate it you get one unique thing.
We're pretty far off course from your original question and firmly in the weeds of "Object oriented javascript".
I do know of a good walkthrough of that topic:
https://developer.mozilla.org/en-US/docs/Learn/JavaScript/Objects/Object-oriented_JS
I want to stress though that you can get by perfectly fine without a deep understanding of all the nuance here.
(I certainly do lol)L
LebombJames•973d ago
I imagine you can, but I'm very much someone who learns how something works by knowing all the moving parts, and then forming my own mental "map" of how it all works. I'll take a look at that guide though, seems useful. Thanks for your help!
LTL
Leo The League Lion•973d ago
@lebombjames1 gave
LeaguePoints™ to @calego (#1 • 1175)
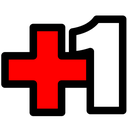
UU
Unknown User•969d ago
Want results from more Discord servers?
More PostsActive Effect HelpAnd it should be the actual ActiveEffect document for the effect, not just the id. I don't have theTOL JEs@badgerwerks I haven't had a chance to poke around in TOB/BoL yet, but I wanted to ask how you did tCI 2 minute windowAlright, I've got a Gitlab CI pipeline I'm reasonably pleased with as a result of yesterday's effortGitLab CI PipelinesToday's project is Gitlab CI.
Wow me with your pipelines if you got em.Storybook Shennanegins@wyrmisis I have a headcannon for you, one who also uses Storybook.
_What if_ we could load foundryjsxDammit I want this...react librarySo far I've seen Vue 2, Vue 3, Alpine, and Svelte pulled into Foundry. No one has yet been crazy enoweb componentsA comment @n1xx1 made over in #development-basics (https://discord.com/channels/732325252788387980/7UI element JS Classeshttps://discord.com/channels/732325252788387980/734922093967310910/882979923483037696
@wyrmisis
> Imid-milestone ping feedback@dnd5e **No Action Required**
Wanted to ping you to make you aware that the 1.5.0 milestone is >50%Hot ReloadingIs there a way to do something like hot module reloading/live reloading on change for Foundry?Tagger Code ReviewWould anyone be keen to code review Tagger? I'd like to git better 😄
https://github.com/Haxxer/Founsocket woesnot the socket firingpreUpdateSo for some reason ItemPF#_preUpdate changes aren't sticking.. what is PF1 doing that prevents this?mergeObject`mergeObject` is a beast of a function which lets you smash two objects together and that options arselect in sheetAlso, is the data actually saved on the actor? You can inspect the data in the console opened with FIC 5e DocsI'm not looking to ping about this but for anyone who sees this: I'm interested to know if anyone hecustom hud elementMaybe I'm missing something simple here. I'm making my own custom hud element (NOT based on a FoundrCover@badgerwerks how does the cover application for 5e helpers work?
I know you do black magic to calculGitpod WorkflowHas anyone figured out a codespaces workflow?