comparing fomula
Anyone know of a foundry utility to compare two strings that might be dice formulae to tell which has the lesser deterministic value?
23 Replies
🧵
use case:
I have two strings that
- might just be numbers ('3' and '4')
- might be simple formula ('3 + 3' and '4')
- might be nonDeterministic formula ('3 + 1d4' and '4')
I want to know:
- if both are deterministic formula, what is the
Floor
of their values
- if they aren't deterministic, I'll do nothing with either value
oh i can use dnd5e's simplifyRollFormula
for this
if the output of simplifyRollFormula
is parsable as a Number
, I can compare them easilyok wtf js
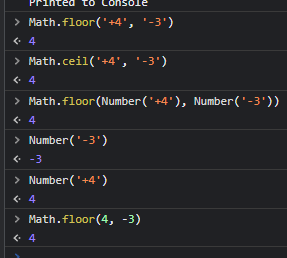
oh, i'm dumb.
.floor
is for rounding
I'm looking for .min
this is definitely excessive...
BUT
it does do what I was looking for and covers all the edge cases I can think of
Most of the time I know of, if there's a bonus, it'll be adding to both msak
and rsak
to represent a global increase
but the data does support disparate mods, in which case, applying the lesser one is 'safe' to this display of 'spell attack modifier'.evaluate({min:true, async:false})
doesn't work for you?
Or did I misunderstand what you were after?is that a method on
Roll
?Yes.
min
would return the minimum possible based on the formula provided?Yes. It sets the dice to minimum value they'd give instead of randomizing them and returns the result.
gotcha, not quite what i'm looking for
I'm really looking to compare two formulas, and use the 'min' one
(but only if they're deterministic)
Roll.isDeterministic is a thing
It also exists for individual terms.
That is available pre-eval
so the alternative to using the dnd5e
simplifyRollFormula
would look something like :
Almost, .isDeterministic is a getter.
And .evaluate() returns Roll instance, so you'd need to add .total there.
Also eval is async unless you tell it to be non-async.
that I could probably DIY that actually if jk not as easy i expected in js
async:false
is being deprecated right?
isDeterministic
is truePeople keep saying it's not. The requirement for it is, because async is becoming the default.
And there's no warning in it that sync option is going away, just that it won't be the default.
goootcha
Anyway, you'd have this:
so then the question i have is 'is the overhead of creating two
Roll
s to compare these strings greater than or less than using simplifyRollFormula
?'
odds are, less than, simplifyRollFormula is a heavy function now that I look closerI'd favor whichever makes the intent of the code clearer.
suspect this will never be clear 😛 but this is a solidly simpler solution and doesn't rely on 5e code
thanks @manaflower
@calego gave
LeaguePoints™ to @manaflower (#15 • 203)
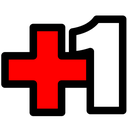
🎉
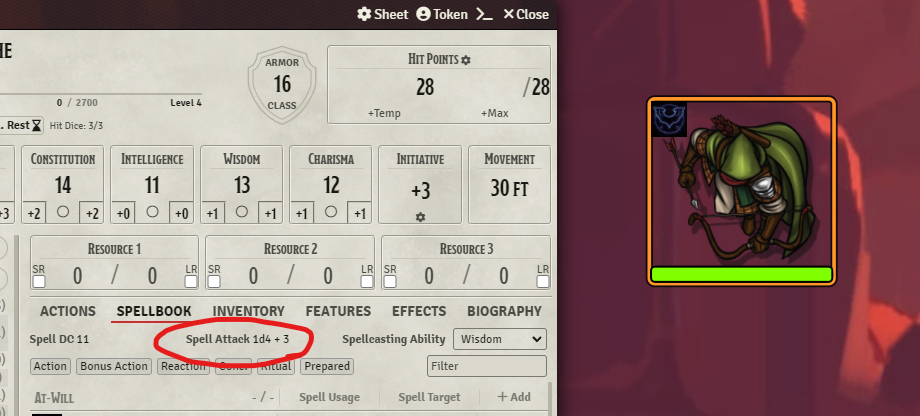
out of interest why do you only want to figure out which is better if it's deterministic?
is it effectively a MVP or do you genuinely never think it will be a non-number
I'm mostly asking because if it's always gonna be a number I'd expect to store it as a
number
but it could be an external constraint.External constraint, could be a simple formula like "+1 +1 +2"