Problem with GuildMemberUpdate
For some reason, it happens that I add the highest (or no) role and it shows that all the roles that the participant already has have been added. How can this be fixed?
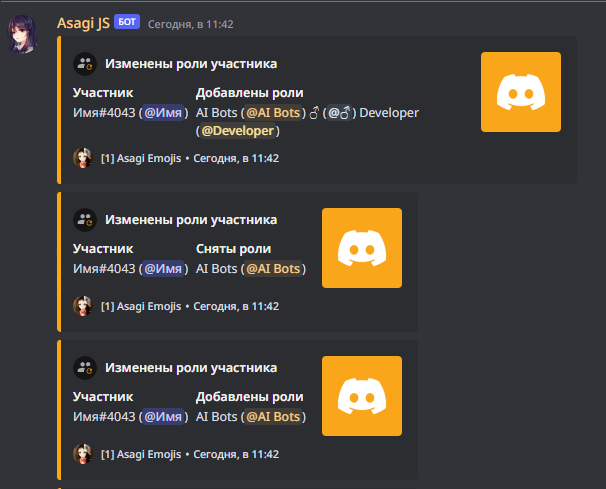
8 Replies
Just use
Btw you don't need to convert a collection to an array. Collection has methods of array, and even more.
If you have
GuildMember
partial the oldMember may be a partial structure and may not has roles.I am now displaying the value of addedRoles in the console and 3 roles are initially displayed and then 1.
Do you have
GuildMember
partial in your client options?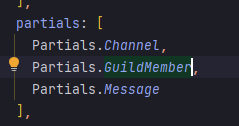
As you can read the guide you can't expect other properties to exist besides the id in partial structures. The guild member should be cached to not be partial in events, but I don't think you want to cache every single member.
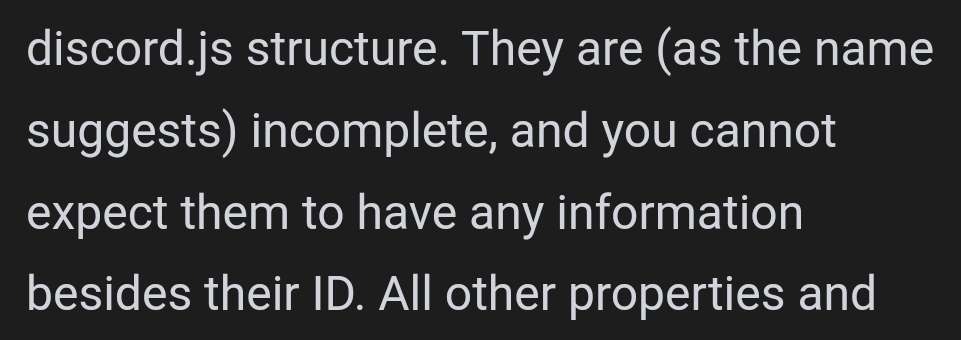
What do you suggest I do?
Ignore partial members or fetch all members