learning JS with FCC, trying to understand something.
As title says i’m learning JS through FCC to understand syntax and for some explanation without having to google everything yet. In the attached photo it’s taking a variable and running it through 2 functions. What i don’t understand is the “global” “local” supposedly things inside the function are local so how is this code taking the sum of the first function and running it through the second when there wasn’t any return. Also why is it showing in console as “undefined” i know it’s because it isn’t asked to return the value but i feel like i’ve saw things before with no return and it’s done the simple math and output a number. Sorry for the phone pic as printscreen isn’t working.
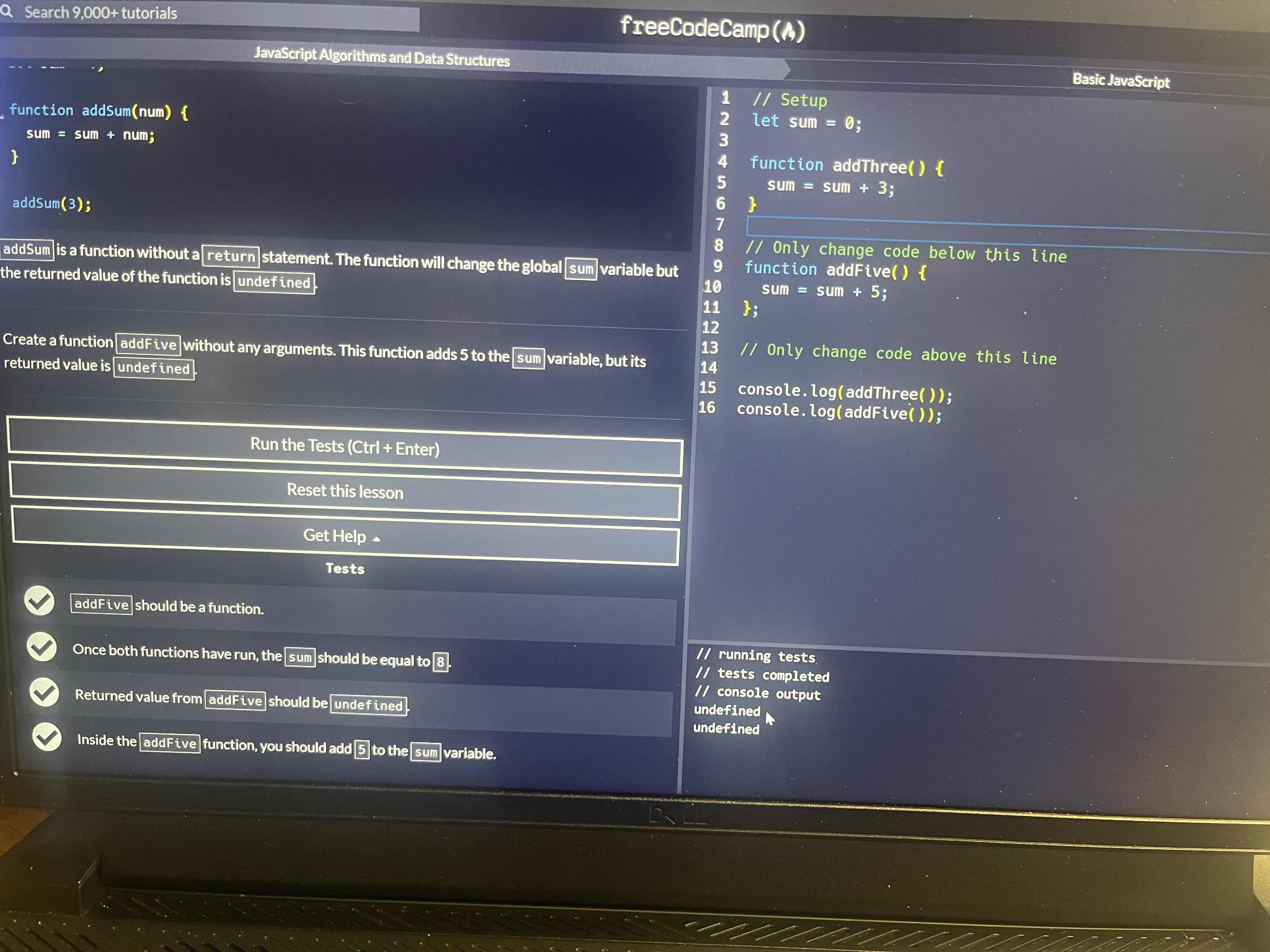
13 Replies
you've probably seen implicit return arrow functions:
That middle one is an arrow function, which automatically returns the result of the statement when you don't include curly brackets
anytime you use the
function
keyword, or you put curly brackets in your arrow function definition, you do need to use return
.
you could rewrite addThree like this to have it return without using return
though I think in this case, you're not really supposed to log out the return of the function, but the value of sum
after running the function:
should output
I’ve yet to see the arrows. Just kinda wondering how if the sum starts off globally and then once it runs through the first function the returned value for that should be local to that function? If so how does it take that value (3) and add 5 more in the next function for it to be 8.
sum is in the global scope, which the functions can access. There's no returned values, it's just editing the value of sum directly
(that rewrite wasn't super helpful btw, now that I'm reading it again. It's not identical to the function you linked, it's the same as
return sum+3;
instead of sum = sum + 3
, sorry for the confusion)Ahh okay. Yeah i see now. The sum is global which allows anything to use it and doesn’t change it to local just because a function used it.
exactly
And since there isn’t a return we get the undefined. Because it doesn’t know what to console log?
it knows what to do with the console log, which is print out the return value of the function, which is undefined because there is no return
let test = addThree()
would result in test
being undefined
Yeah, that’s the part i’m having a little trouble understanding. It’s obviously wrong but in my head i keep saying okay it knows it’s 8 why not show 8 on the addFive()
because you're not asking it to show 8
computers do absolutely nothing without you explicitly asking it to do exactly that precise thing
So the 8 isn’t asked to be shown just stored directly into “sum”?
yeah
Okay, thanks. I get it now.
Being new is hard, appreciate the help lol.
no worries, we all start somewhere 🙂