Weather APP using weatherapi.com
The goal of this is to be able to show the current weather in a specific location (searchable). Now I have access to it, and I can see a Call (the url), and a response body (bunch of JSON).
Now... I know I can use fetch to access or pull (I think pull is the word), the API url, so does that mean I can then access any of the JSON properties without having to add them to my codebase somewhere?
This is the first part of the "response body", and I imagine I do not need to add this to my code, I can just simply access the location.name in my code and it'll appear?
14 Replies
This is the initial code I have built (removed the API key)
You could use the
fetch()
and the response.json()
to get the whole object returned
That's just a simple example I found
To test you got a return
Just change the url to yoursthe url I have seems to be specific to London, I couldn't find a generalized URL
I imagine If I try to search for Amsterdam through that URL, it won't work, as it only contains JSON for London
what's the URL look like?
http://api.weatherapi.com/v1/current.json?key=&q=London&aqi=no
Though I removed my api key after key=
yeah, that's good to remove 🙂
sounds like you just need to change
q=London
to whatever city you're looking for
so http://api.weatherapi.com/v1/current.json?key=&q=Amsterdam&aqi=no
would give you results for Amsterdam
something like
would workoh I didn't think of that
I was trying to also mimic and old API project I've done but I think my lack of understanding around how the code works isn't going well 😄 I'm sure this is wrong on both the 1st line (const location = ) and the last line (app.src = response.location.name)
Waaaait a minute
that seems like it would work, yes 😄
Certainly does, I now just need to figure out how I implement the search function to pick whatever city you want
instead of "let city = "New York";"
you can use something like
document.querySelector().value
to get the value of an input nad pass that in. You would probably want to make sure the value only contains alphanumeric characters though, just to be safe got it, thanks Jochem!
crap I didn't do anything except git init this, I haven't used NPM or anything 😄
Question. I need to also include the temperature in C and F for the city chosen. Where would you put that? I was thinking adding it under here
main function:
it really depends on where you have access to that data. if it's part of the response, they yeah, that would make sense
i looked at the docs, and it should be in the response under
response.current.temp_c
and response.current.temp_f
I got this from their api-explorer: https://www.weatherapi.com/api-explorer.aspx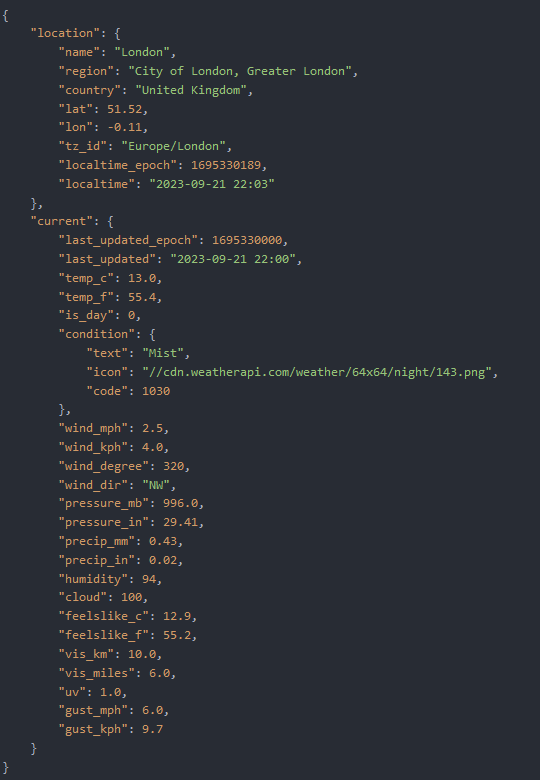
yeah done that and it weorks
I feel like this is a poor way to do it though? as it's all in a textContent
so I'm not really sure how I target them to now customize them in css
Gotta sleep but if anyone is able to offer any insight into how I could go about maybe changing the app.textContent line so that I am able to use CSS to customize the layout of the response, that'd be great. I think I need to add a Class or ID to the response but am not sure how to go about doing it. (code in my message above)