✅ System.Data.SQLiteDatabase .NET 7.0 Reading Items From Database
I use this exact code (change a couple of variable names) in my other version of the math game I created and it keeps hollering at me
object reference not set to an instance of an object
when it comes to reader = cmd.ExecuteReader();
. What is happening? There are 2 records in the database, but it just will not read.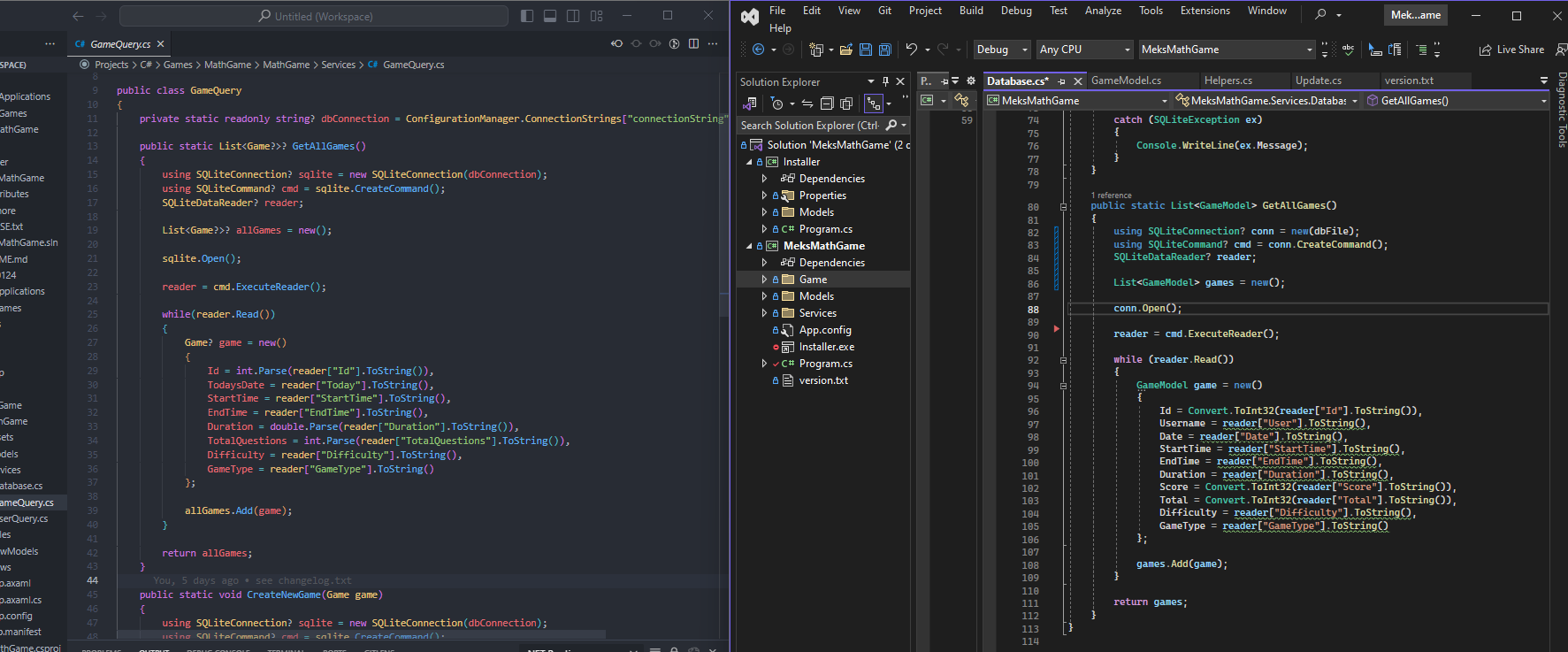
39 Replies
debug to determine what is null
most likely
cmd
assuming dbFile
is correctthis is my
App.config
file and at the top of my database file, I have the line
which I know is correct since it's saving the game records to the database, and creating the database if I delete it and re-run the programdebug on what's null
no need to guess
on a side note,
dbFile
is not a db file, it's a connection string. Naming is kinda confusingit's the reader that's null
so in that case
cmd.ExecuteReader()
is returning null?yes
what is the actual query you're running against the db
what is the
CommandText
of the cmd
the code I have is in the initial post.
I've never had to create CommandText when reading all items from the database.
I use this exact code (change a couple of variable names) in my other version of the math game I create...It reads just fine and normal in my other math game version
I've no idea how you would read anything from a db without an actual query. Maybe someone else can help you, I'm out of suggestions
I'll try putting in a query for the CommandText and see what happens
I appreciate your suggestions thus far
before I try that though, I do have a question for you. What is a better way to write this?
generally I don't do strings on predefined "types", I use enums
I tried doing
but it told me that not all code paths returned a value...
wdym?
I don't like matching strings anywhere, especially when you know what gametypes and difficulties you have
so what do you do instead?
so let's say I did this in my Helpers.cs file, how would I go about using that for what I'm wanting to do?
I think you need a discard for your switch expression
oh
the discard plus changing if else if to an else fixed that problem
are Enums more efficient?
you're not handling the case where gametype is not addition/substraction/multiplication/division
ah, you changed to else
all good
I switched it from an if/else if to an if/else and that fixed that
so let's say theoretically I created the two enums shown here. How would I use them in place of my strings and such?
instead of passing in "Addition" as the
gameType
you pass in GameType.Addition
same goes for the difficulty
oh ok. I'm trying to set one up to learn how to use them, but I'm not sure what I'm doing wrong
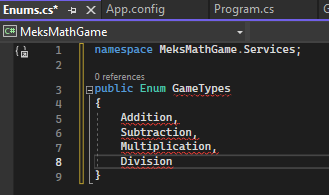
do they getters/setters?
no
ok
are you using top level statements
so it's
enum
not Enum
ah yes
I don't know what a top level statement is and I've never used enums like this before so I think I'll keep the strings if it's not going to hurt anything
the idea is that the developer cannot pass in gameType as "asdf" and difficulty "fdas"
as they are predefined as enums
and generally matching magic strings is a code smell
but yes, one problem at a time
well if I use those enums, then these functions will have to update accordingly and I don't know how to do that
you can always improve the codebase later
ofc you do
so what.
List<string> choices = new() { "e", "m", "h" };
would become....what....List<enum> choices = new() { Difficulties.Easy, Difficulties.Medium, Difficulties.Hard };
?no, those are user inputs
you map them to gametype/difficulty later on
so then should it be
?
yea I'm lost. I'm just not going to worry about it. Thanks anyways though
always make it work first, then improve
the game is done. It's complete. Everything works now and works as it should. I'm pushing a release to my github as we speak. I just wanted to learn about the enums and how to use them is all
you figured out the db problem?
yep. I thought for some odd ball reason that I didn't need to set a
cmd.CommandText = "SELECT * FROM [table]";
and that turned out to be my problem