input file error
when reading numbers in my input file, i convert them to integers and assign specific numbers to a variable such as
but i keep getting an error saying "'Input string was not in a correct format.'" i am unsure how to fix this because the input file is automatically a string, but converting it to an int is causing an error. I normally convert this way and have not had an issue
94 Replies
two things:
1. it's better practice to use
uint.(Try)Parse
than Convert methods - those are older mostly reserved for legacy purposes
2. it doesn't hurt to separate the assignment and conversion so you can more easily see what the "input" is - if it's not the right format, it's not the right format, so you may need to find out why1. i understand, i will try. my prof taught us to code that way, so we've been using that in out coding.
2. could u elaborate please? :333
assuming all your inputs are strings
then when debugging you can inspect the values of
xString
, yString
, zString
ok lemme try,plz hold
Is this a school assignment? Are you required to read your input file using a StreamReader?
You could probably make your life much easier by using JSON serialization & deserialization instead of coding your own parser
yes this is a school assignment
my prof is very old school and encourages us to code this way :///
i just tried and got the same error
I'll look up the try parse functuion
function
don't just ignore the error, what does the error say?
im not
that's why im doing try parse instead of convertto
"input string was not in correct format"
and what does that error mean to you? what is it telling you?
i believe it's something to do on my part with the concersion because the input file is read as one big string
in the past i've ive convertto to convert to an int but thats not working here
used*
instead of "i bleive..." you should check
put a breakpoint
well yeah
that's
why im here
i've checked
and ran into tjat error
so now im trying the try parse
that likely won't help
put a breakpoint
but he never taught us that :///
on the line that is throwing the error
i did
and it gave me the error i told u
:///
putting breakpoints and debugging is a vital skill you need to learn as a software developer
so time to start learning it 🙂
I'll share an example
i am trying and it's hard :///
yes plz
my tutor was teaching me a bit of how to debug , which has helped me a lot in c++
but i admit i am struggling more with c#
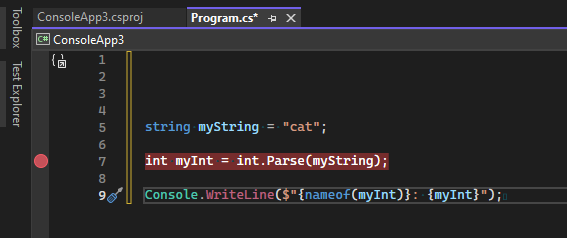
see the red dot on the left side?
that is a "breakpoint"
you click there to place breakpoints
yes i know
and you can click break points to remove them
i used those
ik how to dp that part
by using those is where i foud my error
bc i was debugging i came to that error
if that makes sense
im just now struggling to fix it
then when you run the code, it will stop at the break point and you can hover your mouse over variables and such to see their values
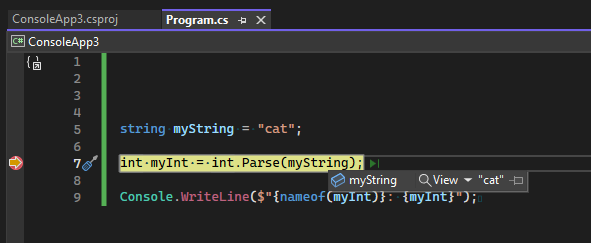
in this case, I am trying to convert/parse
myString
into an int
but the value of myString
is "cat"
and that isn't valid
for an int
so it will throw the same error you are seeingohh okay i see what ur saying
now, in your code, put a breakpoint and tell us what your string looks like that you are trying to convert into a Uint
the there's a header in my file thats "P3" that's suppose to stay a string
otherwise the rest of the file is numbers
i have a feeling it's trying to convert that
one moment plz
turns out my feeling was correct
lemme try to fix plz hold
i keep running into the same error
it keeps trying to convert a string of words to an int
but im not understanding how bc it should be moving on to the next line
it's just staying on the first
it will move on to the next line when you call
ReadLine()
are you calling ReadLine()
multiple times?
can you share your code and maybe your input file?yes one moment
that isn't your full code
where are you defining
nextItem
?oh i thought u meant the portion i was messing up on
one moment
do u need my other files too or no @ZP ░▒▓█├■̶˾̶͞■┤█▓▒░
not yet. trying to get you an example
okay sorry, take ur time
there is an example that seems to work with the input file you provided
you don't need to make both a file stream and a stream reader. you can just directly make the stream reader. also you want to use
using
in front of the stream reader. that is better than closing it later
because it really needs to be in a try
-catch
and using "using
" will do that for you
you had multiple loops and things, but you didn't need som eof the extra stuff you had
also, you can just change "Color" back to "Pixel" from my example. I didn't have your "Pixel" class so I just used System.Drawing.Color as an alternativeI have to keep my stream reader and writer the same because that how my prof wants us to do it
tell him to fuck off
LMAOOOO
im sorry he's very old fashioned :////
seriously though. he shouldn't count off points if you use proper coding practices. if he did I would complain to your school abot that
i doubt he would, but for now, i'd rather stick to what he taught us because, as amazing of an example it is, there's a lot of new stuff i am not familiar with and I don't wanna confuse myself
does my example make sense?
im still reading it, plz hold
what's the "!" for in the 7th line
that is a null forgiving operator
you don't need it
it tells the compiler to ignore a potential null value
what version of C# are you using
visual studios is version 17.7.4 and my .NET version is 4.8.04084
you are using old stuff
that is .NET Framework
that is now legacy
".NET" is the new stuff
".NET Framework" is old and legacy
it is .NET framework
sorry i left out the framework part
i didn’t realize there was a difference
since this is a school assignment for an asshole of a teacher... you are probably stuck using the old shit... but I'm just trying to inform you that you really shouldnt' be using it anymore
ðŸ˜ðŸ˜ðŸ˜
ur not the only one hoenstly
i’ve gotten help multiple times and they’ve told me the same thing
a lot of what he teaches isn’t even in use anymore
it suck’s but he’s the only c# prof my college has :/
how goes on understanding my example? do you need me to convert it back to .NET Framework? what can I do to help you understand it?
yes plz, could u do that
i’m trying to compare it to my own code
i think i get the gist but
i just wanna make sure
i apologize if this is such a hassle
I coded it using .NET 8, which came out last month and has several features you don't have in that older version
One sec I'll back port it
tysm
that compiles in .NET Framework
you can remove the
class Pixel {...}
I just added that since I don't have your class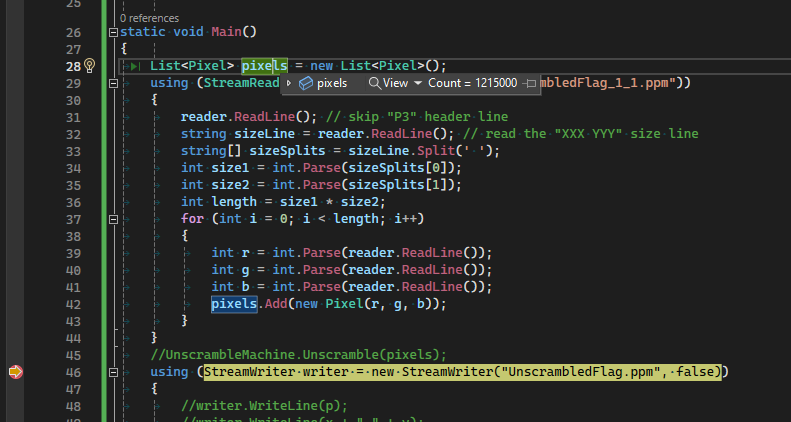
if I put a break point after the
using
for the stream reader, you can see that the list of pixels has 1215000 items in it
because 1350 * 900 = 1215000
(1350 and 900 are the sizes listed in the file on line #2)
If you need to go back to the code your teacher wanted for the stream reader/writer... the reason why I moved them around is because in general you should only keep files open while you need them
the way you were doing it, your file was open the entire time the program is running
but you should only keep the input file open while you are reading it (the first half of your app)
and you should only keep the output file open while you are writing to it (the 2nd part of your app)
keeping files open for longer than necessary causes issuesok lemme read up and make some adjustments
one moment plz
heads up... I may have swapped green and blue compared to your code. in general most graphics libraries tend to use RGB, not RBG
feel free to use RBG if necessary, but that is why I changed it 😛
waitso for the "skipping header" part, i have
because my prof said we had to read it to let our program know we will be working with pixels and pictures
i gotta get rid of that ?
or just get rid of the left half
you just need to skip past the first line of the file. the first line of the file you provided has "P3" which doesn't matter for us
every time you call
ReadLine
it will progress the reader to the next line of the file
one of the problems you had in your code is that you had two loops and that probably wasn't necessary:
you only really need one loop to loop through all the ints in the filebut my prof does the "while !reader.EndOfStream" so we put that in our code
im so sorry this is so lik extra
ðŸ˜
idk y he teaches that way honestly
i feel like i give ppl a stroke whenever they see how i have to code lmao
using
while (!reader.EndOfStream)
is fine
there are often multiple ways to do any task in code
but what I'm getting at is that you only need one loop
not twooh i have three loops
the while loop to read the entire input file, the first for loop to read the pixels and add them to the list, and the last for loop to display the list in the input file
Yes but you are misunderstanding loops
when you put one loop inside another loop it is a "nested loop"
you were nesting loops when at least for the output part of your code you should do that loop after the input loop finishes
Did you try out my code? Did you get it to read in the entire input file?
im still tweaking parts of my code to what you've shown me
one moment
ok that part is solved, but i am not getting that same error in my override ToString() method
one sec
this is my pixel file
the ToString() method at the bottom, I get the same error from earlier
that is ugly... I'm assuming your teacher provided that code?
i call this method at the end when displaying my pixel list
YES LMAOOO
we don't make
Get
and Set
methods in C#... that is a Java thing...apparently no one uses doc strings either ??
C# has "properties"
so we don't need getter and setter methods
he also teaches java :///
according to him we do :///
he should not be teaching C#...
i feel like imma have to unlearn everything he's taught when i transfer to a 4 year uni :////
can you elaborate on what error you are currently seeing?
if the error is happening in the output, it should be a different error
the same one from earlier, 'Input string was not in a correct format.'"
can you get a screenshot of where the error is being thrown?
yes plz hold

o... you have ")" when you need "}"
take a closer look 😉
-__-
i need a cig after thisðŸ˜
not actua;;y but oml
it really be the littlest things i swear
you will get used to finding stuff like that
it never stops 😛 you will always make little typos and crap while coding
but as you improve you will get better and catching it
i remeber i spent an error when i simply needed to make something a word lowercase instead of capitilized ðŸ˜
ok the code compiled
imma check my output file
so it compiled but this def is NOT the dutch flag LMAOOO
i gott tweak my unscrambler file
lol.... it sounds like your original issue of how to handle the input and output is resolved?
yes it is !
tysm like real kitty
really**
do you have any questions about the code?
like i’m truly grateful for ur help
uhhh
i honestly might 💀💀💀
just gimme one second
i did learn a lot from u tho !
my unscramble file literally looks great, i did it with my school tutor
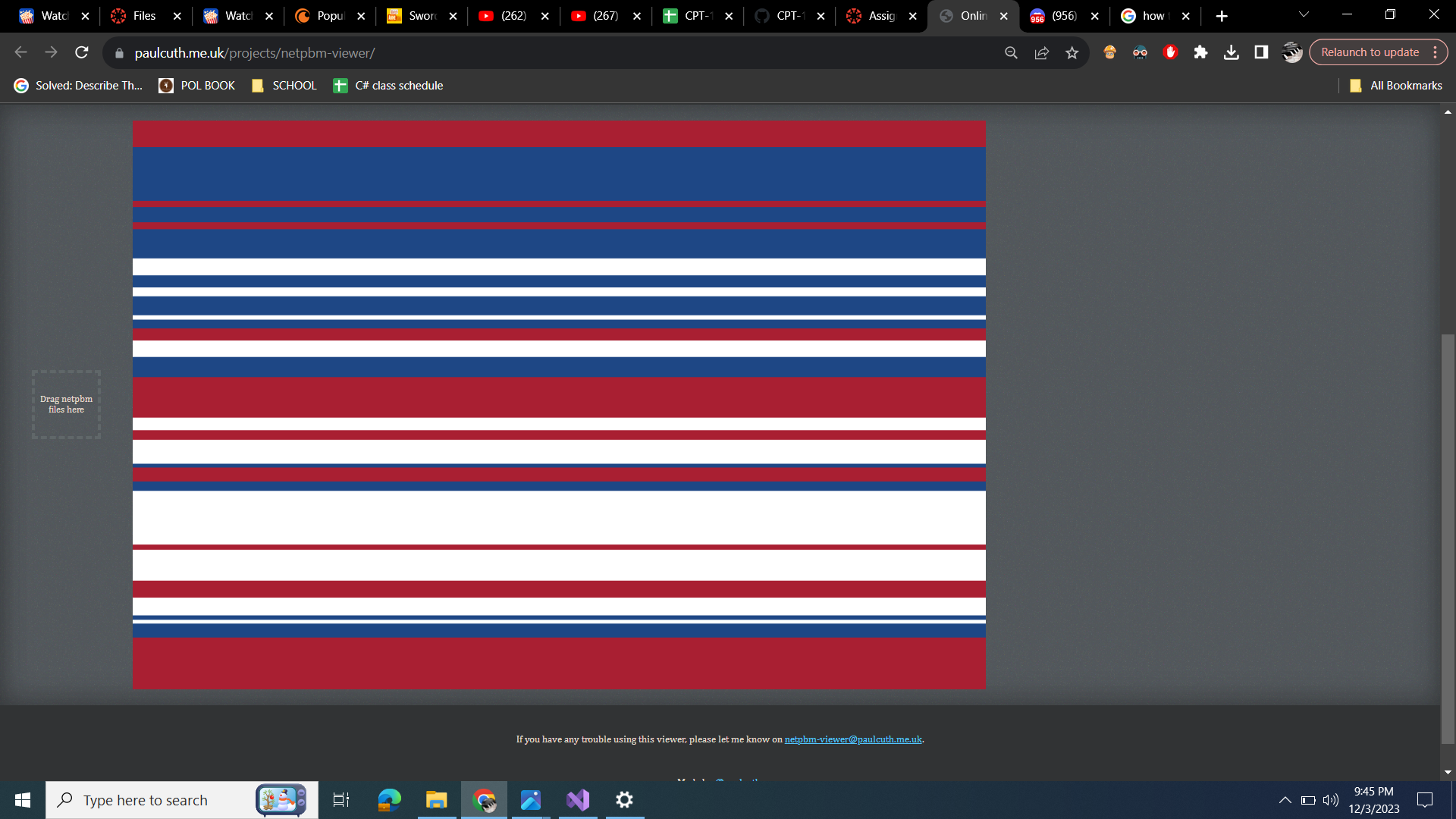
idk how it's ending up like this'it should be solid red, then white, then blue
I will need more info in order to help. did your teacher provide you some instructions explaining what about the input file is "scrambled" and therefore needs to be unscrambled?
also can you share the code for your unscrambling method?
---
I'll have to go offline for the night soon. I would recommend you close this help post since the original issue of handling the input and output is resolved (so other discord users don't have to skim this entire chat), but open a new help post for your unscrambling logic not working correctly. You will need to provide a lot of extra infor for us to help though.
i understand
ty !
i will