Email workers send email
Hello im trying to send an email within the email woker. I tried this:
but i get the error:
Cannot read properties of undefined (reading 'send')
. Im not the best at JS and workers but my guess is that "SEB" is not defined or not the object i need, but i dont have any clue how to fix this. I took code from https://developers.cloudflare.com/email-routing/email-workers/send-email-workers/#example-worker and https://developers.cloudflare.com/email-routing/email-workers/reply-email-workers/19 Replies
Have you configured your wrangler.toml file?
What should be in my wrangler.toml
send_email = [
{name = "SEB"}
]
oh
Now i get the error
(error) :email from gmail.com not allowed because domain was not found
Tried to send an email from my gmail
To the domain
after some fixing with my bad variable names this is what i get: (error) could not send email: could not send email: Unknown error: permanent error (521): 5.5.2 mail.sageee.xyz Error: bare <LF> received
I am unaware of this error. Check in your Cloudflare dashboard in the Email product that you have correctly configured the correct senders. If possible, post a photo here and also post your code and your wrangler.toml here
My current code is the following:
and my wrangler is
I still don't know why its not working
@Alisson Acioli sorry for my late response ^^
Any ideas ._.
@Alisson Acioli you are my last hope :(
Try change:
msg.addMessage({
contentType: 'text/plain',
data:
${ message.raw }
});
to:
msg.addMessage({
contentType: 'text/plain',
data: ${message.raw}
.replace(/\n/g, '\r\n')
});
From the error you indicated, it seems to be something referring to a final line that is being received and could not. Also try to check the text you are sending. If the solution above doesn't work, try adding a direct text to test as data in "data": testing...
Thats, i guess interessting
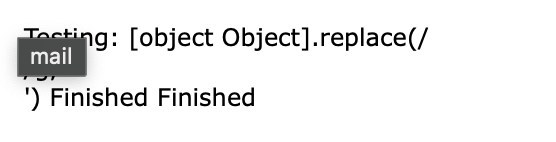
I think the error is some kind of bug with my mail server, because changing it to som other email results in this
Now using this
But still i sadly do not see the content of the mail
Try again:
msg.addMessage({
contentType: 'text/plain',
data: message.raw.replace(/\n/g, '\r\n')
});
If it goes wrong, try:
msg.addMessage({
contentType: 'text/plain',
data: 'Testing'
});
In theory, one of the two would have to work.✘ [ERROR] TypeError: message.raw.replace is not a function
Replace is only valid for strings and raw is a stream
try 2 code
Don't actually know how to convert a stream into string
I google
xd
Actually i do not use js often actually i can count my js experiences on one hand
Buffer.from(message.raw).toString();
Thx <3
After some changes i got the message.raw as string but still not the messagte content ._. https://pastebin.com/9t2gUHwG
Pastebin
Received: from mail-ej1-x62f.google.com (2a00:1450:4864:20::62f)by ...
Pastebin.com is the number one paste tool since 2002. Pastebin is a website where you can store text online for a set period of time.
Content of my mail was keyboard smash or "Asdvdsvdasvsd"
I do not find this in the output
And the docs say it is the email message content
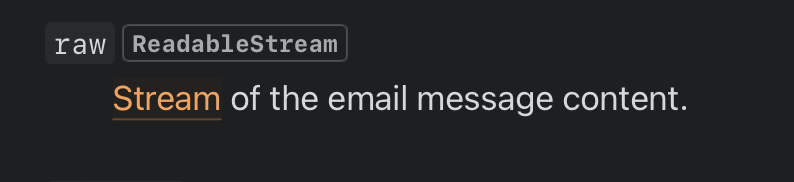
Its finally working
I still do not know why my mail server does not accept these mails but as far at it works on other mails im fine with this