Beginner in EF Core and SQL Server in need for help
Hello, I am new here but I needed some help using my project. I need to connect to a SQL Server DB using .NET Core with EF Core. This is a simple example of my problem:
This would be the table mapped to a C# class, right? However, I need to work with User objects to perform methods and have fields that need to have private setters and getters etc. How would I do that? I was told to user another class like this to wrap around UserModel:
But I ended up in an unsolved problem. When using my code I will work with User objects, right? But I have some questions surrounding this idea (if the best - correct me please if there is any better way).
1) How do I create an instance of User if I don't have a UserModel object? 2) When adding a new UserModel to the DB, how do I do it if I am using User objects throughtout the project, not UserModel objects?Probably dumb questions but basically, how I keep alternating between their uses? There is probably a better way to do it. If so, please I want to do this the best way possible. I am new to EF Core and SQL Server in general but willing to learn anything to make my project the best version possible.
3 Replies
Another unrelated question that has been bugging my mind recently. I have four classes: User, Player, CoachingStaff and Team. Every Player and CoachingStaff are a User and their Primary Key is the User's Primary Key as a One-One relationship and the relationships between Team and the other classes which are defined by:
This is example is simplified for an easier understanding of the question
In this case, how do I limit a User to be either a Player, a CoachingStaff or nothing? I was told this One-Many relationship is wrong and Player and CoachingStaff should have a property like instead:
This is the diagram for this part of the project
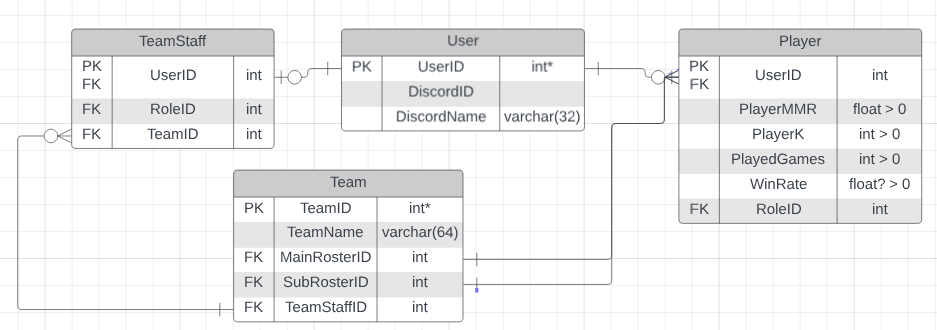
As told earlier, I am a beginner when it comes to SQL Server and EF Core. I am more used to the Object Oriented Programming way of coding so my thought process tends to go on that way. Thanks for any help anyone can give me. Appreciate it!
I have other questions too that I would love if someone could help me find out how some stuff work about EF Core. Let me know if so.