moving from Nuxt2 useFetch to Nuxt3 useAsyncData - how would you refactor this example?
Hello, as a title says, how would you refactor this example to keep SSR working exactly 1:1?
Nuxt 2
24 Replies
I tried to refactor it this way:
But the homePageLoaded is not synced properly and I dont think using
useState
for this is a good way... could you please help/explain, how to refactor it with the smallest amount of other code changes possible?Not a expert, but I think something like this should work
Do not rely on variable name or usage, the main issue is how to modify other refs inside useAsyncData
I am not to sure about your goal, but maybe take a look at the onReponse param ?
otherwise myvar.value seems like the way
@JV Do you mean your homePageLoaded.value is at the end still false?
also note the await before the UseFetch
@BeerDuck exactly!
why not
const { data, status } = await useFetch()
and check if status is PENDING
?
ah, saw this
ideally you don't
a watcher based on data
(or status
or similar) would be betterI try to explain what happens, and maybe it is not 100% correct but. Your code within your asyncdata will not be executed a second time. because the whole function gets skipped, and the data: homepage gets filled in without executing the function again. This is why everything that happens within the function does not get executed a second time. What i think you just want is an normal function around it.
const homePageLoaded = ref(false);
const homePage = ref(1);
async function loaddata(){
const{ data: thedata } = useAsyncData( async () => {
return await loadHomePage();
});
homePage.value=thedata;
homePageLoaded.value = true;
}
await loaddata();
btw above code isnt optimal or directly logical to use in any situation in my mind, but i think this solves your issue. And looks mostly like your old codeOk, and one more question - does useAsyncData have some way how to add event listeners like onRequest like you showed in your example with useFetch? @Wild
watch(() => watachablevalue, (newVal,oldVal) => {
//use variable or do something when variable changes.
});
This keeps track on variable changes if i am correct.useFetch being a shorthand for useAsyncData, it should accept exactly the same parameters, although I can't confirm this will 100% work it should
@manniL / TheAlexLichter sorry for mention, but only you could propably know if this could be a problematic usage?
I know its not ideal usage, but its acrually working - can this lead to some unexpected behavior, or it is safe to use?
I have some purposes why I need to use it somehow like this (rewritting old nuxt2 project into nuxt3 with as little changes as possible accross whole project)
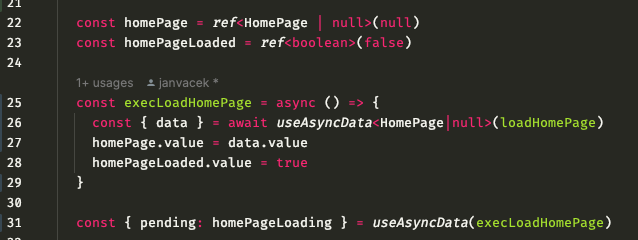
no, useAsyncData in useAsyncData is not okay
It'd help explain "some purposes" 🙂
@manniL / TheAlexLichter thank you for your answer!
Ill try to explain "some purposes"
we are using "useFetch" with our wrapper, which provides some events like "onSuccess", "onError" etc. In some cases, we need to modify data that comes from api call - this maybe could be achieved by computed properties. But I would like to keep our wrapper with "onSuccess", "onError" events
"we need to modify data" -> how about using
transform
? or doing that in asyncData after the fetching the call from an API?I maybe overengineered that, now it looks clear, Ill try to do my best and in case of any other problems Ill let you know - if I can. Thank you so much!
no problem 👍
@manniL / TheAlexLichter how would you implement these functions like "onSuccess"/"onError"? We are using "ste-simple-events" package for that
Hard to tell without a bit more code. but probably in your $fetch (as it has interceptor support)
Im now working with this in mind - trying to create composable which allows me to fetch data of HomePage on SSR and on client either, when I come from another subpage. This is just example in my mind, but I dont think this will be ok too.. @manniL / TheAlexLichter - this immediate: false and calling execute is important for me - Im executing it from another composable
good point!
besides that: execute and immediate false is a bit strange. You could just do a
refresh
which comes from the useAsyncData and return the whole composable "response object" insteadisnt
execute
and refresh
the same functionallity?yes