Commander register api
talking about permissions, im working on the registering api for the Commander, and I wanted to ask for some general feedback: if it makes sense, if I might be missing something obvious, possible ideas for something to add, you name it.
C
ccjmk•815d ago
So, if roles/permissions/anyPermission get merged into a single function, it would look something like this:
maybe I could send the same args to the
canRunIt
function like to handler, in case they want to allow/forbid depending on some command arg. Can't think of a use case, but if Im already parsing it for A, I can send it for B 🤷♂️
That's the most versatile option, but it's certainly a lot simpler for Commander to have user roles to compare or the likeEh not sure if its easier or no. For me it's certainly easier to offload that, I just call the function, if it returns true, good, if not, don't show it, and voilá
C
Calego•815d ago
True, I'm imagining a world where someone is going to want to do async permissions calls
(which could be a pita)
C
ccjmk•815d ago
on second thought about the params to the permissions func, if I want to send the args, I need to show them the function all the time, and forbit it after trying to run it.
so I think its better to at least initially just be a () => boolean
C
Calego•815d ago
Yeah that's unnecessary IMO. The handler can escape hatch out if it gets that far
C
ccjmk•815d ago
yeah ofc.. this would all run inside a huge try-catch, and if anything smells funny I will just show a message and abort
C
Calego•815d ago
Oh a callback would actually be simple for you...
You could
filter using that cb as the return.
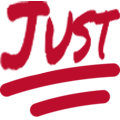
C
ccjmk•815d ago
to filter command suggestions? yeah indeed
C
Calego•815d ago
You will need to define when that check happens though, whether it's cached at load or when the user first opens the menu, etc.
As long as you define it in the docs, whatever you pick will be powerful
Changing lanes, the suggestion callback would benefit from having the current arguments, you could suggest different things based on the current input
C
ccjmk•815d ago
I'll probably do it at module start, just before calling the
commanderReady
hook, comparing against the known list of commands, and then refresh it on any additional registration.
oh that's good
I thought of it like "this is supposed to be a Player Character, go and fetch all PCs", but you could even use one arg to puppet the others with some hocus pocusWant results from more Discord servers?
More PostsAzgaars Blurry MapHey all. I'm taking a look at my importer for Azgaar's Fantasy Map Generator and wondering how I canccjmk overlaybut for what I wanted I think I *needed* to append it there so that I can overlay the whole screencliits very very early alpha demoMonarch APIAlright, adventurous ones: I have a Beta version of Monarch with the new components API!
Manifest: compacted chat cardsModules create problems to solve problems... Today's problem: "When I roll an attack, it eats the whdragdrop shenneneginsRight now, a user can drag a spell onto their character sheet. I store that in a 'spell' array and leffect mini modulesI've gotten to the point where I'm confident in releasing a slurry of tiny no-config Active Effect rdamage application hooksNew 5e Hook ideas, gimme yer thoughts:
`Hooks.call('Actor5e.preDamageApplied')`
args: `damageAmounthook conventionsRegarding hooks, is the convention that _all_ `pre...` hooks are executed on the machine that initiaexpand arraysIs there a way to `expandObject` except for an Array?edit-owned-item-effectI've made an abomination. This hacks its way past the limitations surrounding editing effects on ownItem Pile feedbackSimple is king!effects to chat@badideasbureau is the `temporary` flag you look for in Effects to Chat a convention from somewhere 5e AEsI've been messing pretty heavily with some Active Effect assumptions and mechanisms in 5e. This is mhero creator ui feedbackI'm trying to refactor the abilities' tab on the hero creation room to accomodate Race ASIs, but I deffect mechanic searchingI need someone to check me on a null hypothesis I'm making.
> There are no abilities in 5e RAW whicDialog shennaneginsTIL the button callbacks for `Dialog.prompt` and `Dialog.confirm` can be asynchronous.
```js
const Advancement Hooks@kandashi @ccjmk and anyone else who is currently working on modules that would interact with the AdAPI doc topicsGive me some ideas for what areas of the foundry core API would benefit from a wiki documentation paDiscord Permission IssueOh, I think you also need a Typescript role